
MCP3425 sample
Dependencies: AQM0802 MCP3425 mbed
main.cpp
00001 //********************** 00002 // A/D Converter 00003 // MCP3425 sample for mbed 00004 // 00005 // Voltage=(Vin+)-(Vin-) 00006 // Voltage Range:-2.048V to 2.048V 00007 // Resolution:2.048V/0x7FFF=62.5uV, 16bits 00008 // Sampling Per Second 00009 // 240 SPS, 12bits 00010 // 60 SPS, 14bits 00011 // 15 SPS, 16bits (default) 00012 // 00013 // (C)Copyright 2014 All rights reserved by Y.Onodera 00014 // http://einstlab.web.fc2.com 00015 //********************** 00016 #include "mbed.h" 00017 #include "AQM0802.h" 00018 #include "MCP3425.h" 00019 00020 #if defined(TARGET_LPC1768) 00021 I2C i2c(p28,p27); 00022 #endif 00023 // for TG-LPC11U35-501 00024 #if defined(TARGET_LPC11U35_501) 00025 I2C i2c(P0_5,P0_4); 00026 #endif 00027 // for Nucleo 00028 #if defined(TARGET_NUCLEO_F401RE) 00029 I2C i2c(D14,D15); 00030 #endif 00031 00032 AQM0802 lcd(i2c); 00033 MCP3425 mcp3425(i2c); 00034 00035 00036 int main() { 00037 00038 char msg[10]; 00039 short a; 00040 float v; 00041 00042 sprintf(msg,"MCP3425"); 00043 lcd.locate(0,0); 00044 lcd.print(msg); 00045 wait(1); 00046 00047 while(1) { 00048 00049 a = mcp3425.get(); 00050 sprintf(msg,"dat=%X",(unsigned short)a); 00051 lcd.locate(0,0); 00052 lcd.print(msg); 00053 v = (float)a*2.048/0x7FFF; 00054 sprintf(msg,"%f",v); 00055 lcd.locate(0,1); 00056 lcd.print(msg); 00057 wait(1); // Do not exceed 15SPS with 16bits 00058 } 00059 00060 } 00061
Generated on Wed Jul 13 2022 03:05:41 by
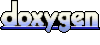