AQM0802 library
Dependents: mbed_EEPROM mbed_UV mbed_DEMO mbed_LPS25H ... more
AQM0802.cpp
00001 //********************** 00002 // AQM0802.cpp for mbed 00003 // 00004 // AQM0802 lcd(P0_5,P0_4); 00005 // or 00006 // I2C i2c(P0_5,P0_4); 00007 // AQM0802 lcd(i2c); 00008 // 00009 // (C)Copyright 2014 All rights reserved by Y.Onodera 00010 // http://einstlab.web.fc2.com 00011 //********************** 00012 00013 #include "mbed.h" 00014 #include "AQM0802.h" 00015 00016 AQM0802::AQM0802 (PinName sda, PinName scl) : _i2c(sda, scl) { 00017 init(); 00018 } 00019 AQM0802::AQM0802 (I2C& p_i2c) : _i2c(p_i2c) { 00020 init(); 00021 } 00022 00023 void AQM0802::put(unsigned char a, unsigned char b) 00024 { 00025 buf[0]=a; 00026 buf[1]=b; 00027 _i2c.write(AQM0802_ADDR, buf, 2); 00028 } 00029 00030 00031 void AQM0802::get(unsigned char a) 00032 { 00033 buf[0] = a; 00034 _i2c.write(AQM0802_ADDR, buf, 1, true); // no stop, repeated 00035 _i2c.read( AQM0802_ADDR, buf, 1); 00036 00037 } 00038 00039 void AQM0802::cls() 00040 { 00041 // Clear Display = 0x01 00042 put(CMD, 0x01); 00043 // Wait 1.08ms 00044 wait_ms(2); 00045 00046 } 00047 00048 void AQM0802::locate(int x, int y) 00049 { 00050 00051 // 8x2 00052 put(CMD, 0x80 + y*0x40 + x); 00053 00054 } 00055 00056 void AQM0802::print(const char *a) 00057 { 00058 00059 while(*a != '\0') 00060 { 00061 put(DAT, *a); 00062 a++; 00063 } 00064 00065 } 00066 00067 void AQM0802::init() 00068 { 00069 // Wait 40ms 00070 wait_ms(100); 00071 // Function set = 0x38 00072 put(CMD, 0x38); 00073 // Wait 26.3us 00074 wait_ms(1); 00075 // Function set = 0x39 00076 put(CMD, 0x39); 00077 // Wait 26.3us 00078 wait_ms(1); 00079 // Internal OSC frequency = 0x14 00080 put(CMD, 0x14); 00081 // Wait 26.3us 00082 wait_ms(1); 00083 // Contrast set = 0x70 00084 put(CMD, 0x70); 00085 // Wait 26.3us 00086 wait_ms(1); 00087 // Power/ICON/Contrast control = 0x56 00088 put(CMD, 0x56); 00089 // Wait 26.3us 00090 wait_ms(1); 00091 // Follower control = 0x6C 00092 put(CMD, 0x6C); 00093 // Wait 200ms 00094 wait_ms(200); 00095 // Function set = 0x38 00096 put(CMD, 0x38); 00097 // Wait 26.3us 00098 wait_ms(1); 00099 // Display ON/OFF control = 0x0C 00100 put(CMD, 0x0C); 00101 // Wait 26.3us 00102 wait_ms(1); 00103 // Clear Display = 0x01 00104 put(CMD, 0x01); 00105 // Wait 1.08ms 00106 wait_ms(2); 00107 00108 } 00109 00110 00111
Generated on Thu Jul 14 2022 03:05:49 by
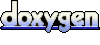