
Test of the BMP180 sensor (Temperature and pression) with the nRF51822 platform.
Fork of BMP180_example by
main.cpp
00001 #include <stdio.h> 00002 #include "mbed.h" 00003 #include "BMP180.h" 00004 00005 I2C i2c(D14, D15); 00006 BMP180 bmp180(&i2c); 00007 00008 int main(void) { 00009 00010 while(1) { 00011 if (bmp180.init() != 0) { 00012 printf("Error communicating with BMP180\r\n"); 00013 } else { 00014 printf("Initialized BMP180\r\n"); 00015 break; 00016 } 00017 wait(1); 00018 } 00019 00020 while(1) { 00021 bmp180.startTemperature(); 00022 wait_ms(5); // Wait for conversion to complete 00023 float temp; 00024 if(bmp180.getTemperature(&temp) != 0) { 00025 printf("Error getting temperature\r\n"); 00026 continue; 00027 } 00028 00029 bmp180.startPressure(BMP180::ULTRA_LOW_POWER); 00030 wait_ms(10); // Wait for conversion to complete 00031 int pressure; 00032 if(bmp180.getPressure(&pressure) != 0) { 00033 printf("Error getting pressure\r\n"); 00034 continue; 00035 } 00036 00037 printf("Pressure = %d Pa Temperature = %f C\r\n", pressure, temp); 00038 wait(1); 00039 } 00040 }
Generated on Wed Jul 13 2022 18:24:38 by
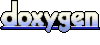