XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
CoreAPI Class Reference
The core API class which responseable for processing frame data, but not the serial operation. More...
#include <CoreAPI.h>
Public Member Functions | |
void | setVerifyChecksum (bool isCheck) |
Set whether to verify checksum during receiving, default is not verify. | |
void | start () |
Start send and process response, must call this method before starting processing data. | |
void | stop () |
Stop the serial port. | |
void | send (APIFrame *request) |
A general function to send frame out. | |
APIFrame * | getResponse () |
Read the next avaliable API frame, and the type of fram can be retrieved from getFrameType(). | |
XBeeRx64Indicator * | getXBeeRx64 () |
Read the next avaliable API frame. | |
XBeeRx16Indicator * | getXBeeRx16 () |
Read the next avaliable API frame. | |
XBeeRx64IOSampleIndicator * | getXBeeRx64IOSample () |
Read the next avaliable API frame. | |
XBeeRx16IOSampleIndicator * | getXBeeRx16IOSample () |
Read the next avaliable API frame. | |
XBeeTxStatusIndicator * | getXBeeTxStatus () |
Read the next avaliable API frame. | |
ATCommandIndicator * | getATCommand () |
Read the next avaliable API frame. | |
ModemStatusIndicator * | getModemStatus () |
Read the next avaliable API frame. | |
ZigBeeTxStatusIndicator * | getZigBeeTxStatus () |
Read the next avaliable API frame. | |
ZigBeeRxIndicator * | getZigBeeRx () |
Read the next avaliable API frame. | |
ZigBeeExplicitRxIndicator * | getZigBeeExplicitRx () |
Read the next avaliable API frame. | |
ZigBeeIOSampleIndicator * | getZigBeeIOSample () |
Read the next avaliable API frame. | |
SensorReadIndicator * | getSensorRead () |
Read the next avaliable API frame. | |
NodeIdentificationIndicator * | getNodeIdentification () |
Read the next avaliable API frame. | |
RemoteCommandIndicator * | getRemoteCommand () |
Read the next avaliable API frame. | |
RouteRecordIndicator * | getRouteRecord () |
Read the next avaliable API frame. | |
ManyToOneRouteIndicator * | getManyToOneRoute () |
Read the next avaliable API frame. | |
ATCommandIndicator * | setPinFunction (Pin *pin, unsigned char function) |
RemoteCommandIndicator * | setRemotePinFunction (Address *remoteAddress, Pin *pin, unsigned char function) |
bool | forceXBeeLocalIOSample () |
The command will immediately return an "OK" response. | |
IOSamples * | forceZigBeeLocalIOSample () |
Return 1 IO sample from the local module. | |
IOSamples * | forceXBeeRemoteIOSample (Address *remote) |
Return 1 IO sample only, Samples before TX (IT) does not affect. | |
IOSamples * | forceZigBeeRemoteIOSample (Address *remote) |
Return 1 IO sample only. | |
Protected Member Functions | |
int | readByte () |
Read one byte payload, which allready handle the escape char, if less than 0 means error occured. | |
void | writeByte (unsigned char data) |
Write one byte to the payload, which allready handle the escape char. | |
void | packetProcess () |
Processing API frame. | |
int | getLength () |
Get the next avaliable API frame length. | |
void | readPayLoad (int length) |
Read the next avaliable API frame data. |
Detailed Description
The core API class which responseable for processing frame data, but not the serial operation.
Definition at line 39 of file CoreAPI.h.
Member Function Documentation
bool forceXBeeLocalIOSample | ( | ) |
The command will immediately return an "OK" response.
The data will follow in the normal API format for DIO data event.
- Returns:
- true if the command is "OK", false if no IO is enabled.
Definition at line 545 of file CoreAPI.cpp.
IOSamples * forceXBeeRemoteIOSample | ( | Address * | remote ) |
Return 1 IO sample only, Samples before TX (IT) does not affect.
<param name="remote"Remote address of the device>
- Returns:
Definition at line 568 of file CoreAPI.cpp.
IOSamples * forceZigBeeLocalIOSample | ( | ) |
IOSamples * forceZigBeeRemoteIOSample | ( | Address * | remote ) |
Return 1 IO sample only.
- Parameters:
-
remote Remote address of the device
- Returns:
Definition at line 575 of file CoreAPI.cpp.
ATCommandIndicator * getATCommand | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 181 of file CoreAPI.cpp.
int getLength | ( | ) | [protected] |
Get the next avaliable API frame length.
Definition at line 114 of file CoreAPI.cpp.
ManyToOneRouteIndicator * getManyToOneRoute | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 281 of file CoreAPI.cpp.
ModemStatusIndicator * getModemStatus | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 191 of file CoreAPI.cpp.
NodeIdentificationIndicator * getNodeIdentification | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 251 of file CoreAPI.cpp.
RemoteCommandIndicator * getRemoteCommand | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 261 of file CoreAPI.cpp.
APIFrame * getResponse | ( | ) |
Read the next avaliable API frame, and the type of fram can be retrieved from getFrameType().
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 90 of file CoreAPI.cpp.
RouteRecordIndicator * getRouteRecord | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 271 of file CoreAPI.cpp.
SensorReadIndicator * getSensorRead | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 241 of file CoreAPI.cpp.
XBeeRx16Indicator * getXBeeRx16 | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 141 of file CoreAPI.cpp.
XBeeRx16IOSampleIndicator * getXBeeRx16IOSample | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 161 of file CoreAPI.cpp.
XBeeRx64Indicator * getXBeeRx64 | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 131 of file CoreAPI.cpp.
XBeeRx64IOSampleIndicator * getXBeeRx64IOSample | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 151 of file CoreAPI.cpp.
XBeeTxStatusIndicator * getXBeeTxStatus | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 171 of file CoreAPI.cpp.
ZigBeeExplicitRxIndicator * getZigBeeExplicitRx | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 221 of file CoreAPI.cpp.
ZigBeeIOSampleIndicator * getZigBeeIOSample | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 231 of file CoreAPI.cpp.
ZigBeeRxIndicator * getZigBeeRx | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 211 of file CoreAPI.cpp.
ZigBeeTxStatusIndicator * getZigBeeTxStatus | ( | ) |
Read the next avaliable API frame.
- Returns:
- a API frame, NULL means data not avaliable.
Definition at line 201 of file CoreAPI.cpp.
void packetProcess | ( | ) | [protected] |
Processing API frame.
int readByte | ( | ) | [protected] |
Read one byte payload, which allready handle the escape char, if less than 0 means error occured.
- Returns:
- if less than 0 means error occured.
Definition at line 67 of file CoreAPI.cpp.
void readPayLoad | ( | int | length ) | [protected] |
Read the next avaliable API frame data.
Definition at line 123 of file CoreAPI.cpp.
void send | ( | APIFrame * | request ) |
A general function to send frame out.
- Parameters:
-
request any API frame
Definition at line 48 of file CoreAPI.cpp.
ATCommandIndicator * setPinFunction | ( | Pin * | pin, |
unsigned char | function | ||
) |
- Parameters:
-
function DISABLED = 0x00, RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, ANALOG_INPUT_SINGLE_ENDED = 0x02, DIGITAL_INPUT_MONITORED = 0x03, DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09
Definition at line 525 of file CoreAPI.cpp.
RemoteCommandIndicator * setRemotePinFunction | ( | Address * | remoteAddress, |
Pin * | pin, | ||
unsigned char | function | ||
) |
- Parameters:
-
function DISABLED = 0x00, RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, ANALOG_INPUT_SINGLE_ENDED = 0x02, DIGITAL_INPUT_MONITORED = 0x03, DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09
Definition at line 535 of file CoreAPI.cpp.
void setVerifyChecksum | ( | bool | isCheck ) |
Set whether to verify checksum during receiving, default is not verify.
- Parameters:
-
isCheck true only to process API frame when checksum matches. false ignore the checksum.
Definition at line 43 of file CoreAPI.cpp.
void start | ( | ) |
Start send and process response, must call this method before starting processing data.
Definition at line 25 of file CoreAPI.cpp.
void stop | ( | ) |
Stop the serial port.
Definition at line 35 of file CoreAPI.cpp.
void writeByte | ( | unsigned char | data ) | [protected] |
Write one byte to the payload, which allready handle the escape char.
- Parameters:
-
data one byte [0x00-0xFF]
Definition at line 77 of file CoreAPI.cpp.
Generated on Tue Jul 12 2022 18:56:10 by
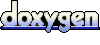