XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
CoreAPI.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_CoreAPI 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_CoreAPI 00003 00004 #include "APIFrame.h" 00005 #include "ISerial.h" 00006 00007 #include "ATCommandRequest.h" 00008 #include "CreateSourceRouteRequest.h" 00009 #include "IOCDetectionConfigRequest.h" 00010 #include "PinConfigurationRequest.h" 00011 #include "RemoteATCommandRequest.h" 00012 #include "RemoteIODetectionConfigRequest.h" 00013 #include "RemotePinConfigurationRequest.h" 00014 #include "XBeeTx16Request.h" 00015 #include "XBeeTx64Request.h" 00016 #include "ZigBeeExplicitTxRequest.h" 00017 #include "ZigBeeTxRequest.h" 00018 00019 #include "XBeeRx64Indicator.h" 00020 #include "XBeeRx16Indicator.h" 00021 #include "XBeeRx64IOSampleIndicator.h" 00022 #include "XBeeRx16IOSampleIndicator.h" 00023 #include "XBeeTxStatusIndicator.h" 00024 #include "ATCommandIndicator.h" 00025 #include "ModemStatusIndicator.h" 00026 #include "ZigBeeTxStatusIndicator.h" 00027 #include "ZigBeeRxIndicator.h" 00028 #include "ZigBeeExplicitRxIndicator.h" 00029 #include "ZigBeeIOSampleIndicator.h" 00030 #include "SensorReadIndicator.h" 00031 #include "NodeIdentificationIndicator.h" 00032 #include "RemoteCommandIndicator.h" 00033 #include "RouteRecordIndicator.h" 00034 #include "ManyToOneRouteIndicator.h" 00035 00036 /** 00037 * The core API class which responseable for processing frame data, but not the serial operation. 00038 */ 00039 class CoreAPI 00040 { 00041 private: 00042 static const unsigned char KEY = 0x7E; 00043 static const unsigned char ESCAPED = 0x7D; 00044 static const unsigned char XON = 0x11; 00045 static const unsigned char XOFF = 0x13; 00046 static const unsigned int INITIAL_FRAME_LENGTH = 10; 00047 00048 ISerial * serial; 00049 bool isEscapeMode; 00050 bool isRunning; 00051 bool isChecksum; 00052 Timer timer; 00053 00054 unsigned char waitFrameID; 00055 00056 APIFrame * msg; 00057 XBeeRx64Indicator xBeeRx64Indicator; 00058 XBeeRx16Indicator xBeeRx16Indicator; 00059 XBeeRx64IOSampleIndicator xBeeRx64IOSampleIndicator; 00060 XBeeRx16IOSampleIndicator xBeeRx16IOSampleIndicator; 00061 XBeeTxStatusIndicator xBeeTxStatusIndicator; 00062 ATCommandIndicator aTCommandIndicator; 00063 ModemStatusIndicator modemStatusIndicator; 00064 ZigBeeTxStatusIndicator zigBeeTxStatusIndicator; 00065 ZigBeeRxIndicator zigBeeRxIndicator; 00066 ZigBeeExplicitRxIndicator zigBeeExplicitRxIndicator; 00067 ZigBeeIOSampleIndicator zigBeeIOSampleIndicator; 00068 SensorReadIndicator sensorReadIndicator; 00069 NodeIdentificationIndicator nodeIdentificationIndicator; 00070 RemoteCommandIndicator remoteCommandIndicator; 00071 RouteRecordIndicator routeRecordIndicator; 00072 ManyToOneRouteIndicator manyToOneRouteIndicator; 00073 00074 protected: 00075 00076 /** Read one byte payload, which allready handle the escape char, if less than 0 means error occured 00077 * @returns if less than 0 means error occured. 00078 */ 00079 int readByte(); 00080 00081 /** Write one byte to the payload, which allready handle the escape char. 00082 * @param data one byte [0x00-0xFF] 00083 */ 00084 void writeByte(unsigned char data); 00085 00086 /// Processing API frame. 00087 void packetProcess(); 00088 00089 /// Get the next avaliable API frame length. 00090 int getLength(); 00091 00092 /// Read the next avaliable API frame data. 00093 void readPayLoad(int length); 00094 00095 public: 00096 CoreAPI(ISerial * serial, bool escape); 00097 00098 ~CoreAPI(); 00099 00100 /** Set whether to verify checksum during receiving, default is not verify. 00101 * 00102 * @param isCheck true only to process API frame when checksum matches. 00103 * false ignore the checksum. 00104 */ 00105 void setVerifyChecksum(bool isCheck); 00106 00107 /// Start send and process response, must call this method before starting processing data. 00108 void start(); 00109 00110 /// Stop the serial port. 00111 void stop(); 00112 00113 /** A general function to send frame out 00114 * 00115 * @param request any API frame 00116 */ 00117 void send(APIFrame * request); 00118 00119 /** Read the next avaliable API frame, and the type of fram can be retrieved from getFrameType(). 00120 * 00121 * @returns a API frame, NULL means data not avaliable. 00122 */ 00123 APIFrame * getResponse(); 00124 00125 /** Read the next avaliable API frame. 00126 * 00127 * @returns a API frame, NULL means data not avaliable. 00128 */ 00129 XBeeRx64Indicator * getXBeeRx64(); 00130 00131 /** Read the next avaliable API frame. 00132 * 00133 * @returns a API frame, NULL means data not avaliable. 00134 */ 00135 XBeeRx16Indicator * getXBeeRx16(); 00136 00137 /** Read the next avaliable API frame. 00138 * 00139 * @returns a API frame, NULL means data not avaliable. 00140 */ 00141 XBeeRx64IOSampleIndicator * getXBeeRx64IOSample(); 00142 00143 /** Read the next avaliable API frame. 00144 * 00145 * @returns a API frame, NULL means data not avaliable. 00146 */ 00147 XBeeRx16IOSampleIndicator * getXBeeRx16IOSample(); 00148 00149 /** Read the next avaliable API frame. 00150 * 00151 * @returns a API frame, NULL means data not avaliable. 00152 */ 00153 XBeeTxStatusIndicator * getXBeeTxStatus(); 00154 00155 /** Read the next avaliable API frame. 00156 * 00157 * @returns a API frame, NULL means data not avaliable. 00158 */ 00159 ATCommandIndicator * getATCommand(); 00160 00161 /** Read the next avaliable API frame. 00162 * 00163 * @returns a API frame, NULL means data not avaliable. 00164 */ 00165 ModemStatusIndicator * getModemStatus(); 00166 00167 /** Read the next avaliable API frame. 00168 * 00169 * @returns a API frame, NULL means data not avaliable. 00170 */ 00171 ZigBeeTxStatusIndicator * getZigBeeTxStatus(); 00172 00173 /** Read the next avaliable API frame. 00174 * 00175 * @returns a API frame, NULL means data not avaliable. 00176 */ 00177 ZigBeeRxIndicator * getZigBeeRx(); 00178 00179 /** Read the next avaliable API frame. 00180 * 00181 * @returns a API frame, NULL means data not avaliable. 00182 */ 00183 ZigBeeExplicitRxIndicator * getZigBeeExplicitRx(); 00184 00185 /** Read the next avaliable API frame. 00186 * 00187 * @returns a API frame, NULL means data not avaliable. 00188 */ 00189 ZigBeeIOSampleIndicator * getZigBeeIOSample(); 00190 00191 /** Read the next avaliable API frame. 00192 * 00193 * @returns a API frame, NULL means data not avaliable. 00194 */ 00195 SensorReadIndicator * getSensorRead(); 00196 00197 /** Read the next avaliable API frame. 00198 * 00199 * @returns a API frame, NULL means data not avaliable. 00200 */ 00201 NodeIdentificationIndicator * getNodeIdentification(); 00202 00203 /** Read the next avaliable API frame. 00204 * 00205 * @returns a API frame, NULL means data not avaliable. 00206 */ 00207 RemoteCommandIndicator * getRemoteCommand(); 00208 00209 /** Read the next avaliable API frame. 00210 * 00211 * @returns a API frame, NULL means data not avaliable. 00212 */ 00213 RouteRecordIndicator * getRouteRecord(); 00214 00215 /** Read the next avaliable API frame. 00216 * 00217 * @returns a API frame, NULL means data not avaliable. 00218 */ 00219 ManyToOneRouteIndicator * getManyToOneRoute(); 00220 00221 XBeeTxStatusIndicator * sendXBeeTx16(Address * remoteAddress, OptionsBase * option, const unsigned char * payload, int offset, int length); 00222 00223 XBeeTxStatusIndicator * sendXBeeTx64(Address * remoteAddress, OptionsBase * option, const unsigned char * payload, int offset, int length); 00224 00225 ATCommandIndicator * sendATCommand(const char * command, bool applyChange, const unsigned char * parameter = NULL, int offset = 0, int length = 0); 00226 00227 RemoteCommandIndicator * sendRemoteATCommand(Address * remoteAddress, const char * command, OptionsBase * transmitOptions, const unsigned char * parameter = NULL, int parameterOffset = 0, int parameterLength = 0); 00228 00229 ZigBeeTxStatusIndicator * sendZigBeeTx(Address * remoteAddress, OptionsBase * option, const unsigned char * payload, int offset, int length); 00230 00231 ZigBeeTxStatusIndicator * sendZigBeeExplicitTx(ExplicitAddress * remoteAddress, OptionsBase * option, const unsigned char * payload, int offset, int length); 00232 00233 /** 00234 * @param function 00235 * DISABLED = 0x00, 00236 * RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, 00237 * ANALOG_INPUT_SINGLE_ENDED = 0x02, 00238 * DIGITAL_INPUT_MONITORED = 0x03, 00239 * DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, 00240 * DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, 00241 * ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09 00242 */ 00243 ATCommandIndicator * setPinFunction (Pin * pin, unsigned char function); 00244 00245 ATCommandIndicator * setIODetection(Pin ** pins, int size); 00246 00247 /** 00248 * @param function 00249 * DISABLED = 0x00, 00250 * RESERVED_FOR_PIN_SPECIFIC_ALTERNATE_FUNCTIONALITIES = 0x01, 00251 * ANALOG_INPUT_SINGLE_ENDED = 0x02, 00252 * DIGITAL_INPUT_MONITORED = 0x03, 00253 * DIGITAL_OUTPUT_DEFAULT_LOW = 0x04, 00254 * DIGITAL_OUTPUT_DEFAULT_HIGH = 0x05, 00255 * ALTERNATE_FUNCTIONALITIES_WHERE_APPLICABLE = 0x06//0x06~0x09 00256 */ 00257 RemoteCommandIndicator * setRemotePinFunction (Address * remoteAddress, Pin * pin, unsigned char function); 00258 00259 RemoteCommandIndicator * setRemoteIODetection(Address * remoteAddress, Pin ** pins, int size); 00260 00261 /// <summary> 00262 /// The command will immediately return an "OK" response. The data will follow in the normal API format for DIO data event. 00263 /// </summary> 00264 /// <returns>true if the command is "OK", false if no IO is enabled.</returns> 00265 bool forceXBeeLocalIOSample(); 00266 00267 /// <summary> 00268 /// Return 1 IO sample from the local module. 00269 /// </summary> 00270 /// <returns></returns> 00271 IOSamples * forceZigBeeLocalIOSample(); 00272 00273 /// <summary> 00274 /// Return 1 IO sample only, Samples before TX (IT) does not affect. 00275 /// </summary> 00276 /// <param name="remote"Remote address of the device></param> 00277 /// <returns></returns> 00278 IOSamples * forceXBeeRemoteIOSample(Address * remote); 00279 00280 /// <summary> 00281 /// Return 1 IO sample only. 00282 /// </summary> 00283 /// <param name="remote">Remote address of the device</param> 00284 /// <returns></returns> 00285 IOSamples * forceZigBeeRemoteIOSample(Address * remote); 00286 }; 00287 00288 #endif
Generated on Tue Jul 12 2022 18:56:10 by
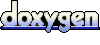