Library to handle a quadrature encoder with interrupts
Embed:
(wiki syntax)
Show/hide line numbers
Encoder.h
00001 #ifndef ENCODER_H_INCLUDED 00002 #define ENCODER_H_INCLUDED 00003 00004 /** Class to handle a rotary encoder 00005 * The lib is interrupt based, therefore no update functions have to be called. 00006 * Example usage: 00007 * @code 00008 * 00009 * #include "mbed.h" 00010 * #include "Encoder.h" 00011 * 00012 * RotaryEncoder encoder(D3, D4); 00013 * 00014 * int main() 00015 * { 00016 * while(1) 00017 * { 00018 * int pos = encoder.position(); 00019 * wait(1.0f); 00020 * } 00021 * } 00022 * @endcode 00023 */ 00024 class RotaryEncoder { 00025 public: 00026 RotaryEncoder(PinName outa, PinName outb) 00027 : position_(0), delta_(0), 00028 inta_(outa), intb_(outb), outa_(outa), outb_(outb) { 00029 outa_.mode(PullUp); 00030 outb_.mode(PullUp); 00031 inta_.rise(this, &RotaryEncoder::handleIntA); 00032 inta_.fall(this, &RotaryEncoder::handleIntA); 00033 intb_.rise(this, &RotaryEncoder::handleIntB); 00034 intb_.fall(this, &RotaryEncoder::handleIntB); 00035 } 00036 00037 /** Returns the overall position of the encoder 00038 */ 00039 int position() { 00040 return position_; 00041 } 00042 00043 /** Returns the encoders change since the last call to this function 00044 */ 00045 int delta() { 00046 int d = delta_; 00047 delta_ = 0; 00048 return d; 00049 } 00050 00051 private: 00052 00053 // A = B --> Clockwise 00054 // A /= B --> Counter clockwise 00055 void handleIntA() { 00056 if (outa_ == outb_) { 00057 increment(1); 00058 } else { 00059 increment(-1); 00060 } 00061 } 00062 00063 // A = B --> Counter clockwise 00064 // A /= B --> Clockwise 00065 void handleIntB() { 00066 if (outa_ == outb_) { 00067 increment(-1); 00068 } else { 00069 increment(1); 00070 } 00071 } 00072 00073 void increment(int i) { 00074 position_ += i; 00075 delta_ += i; 00076 } 00077 00078 int position_; 00079 int delta_; 00080 00081 InterruptIn inta_; 00082 InterruptIn intb_; 00083 DigitalIn outa_; 00084 DigitalIn outb_; 00085 }; 00086 00087 #endif /* ENCODER_H_INCLUDED */
Generated on Mon Jul 18 2022 21:55:59 by
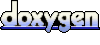