Mbed driver for adafruit trellis.
Dependents: Adafruit_Trellis_Hello_World
Adafruit_Trellis Class Reference
A class for Adafruit trellis. More...
#include <Adafruit_Trellis.h>
Public Member Functions | |
Adafruit_Trellis (void) | |
Create a new trellis object. | |
void | begin (I2C *_wire, uint8_t _addr) |
Initialize the trellis controller. | |
void | setBrightness (uint8_t b) |
Set brightness(PWM) of LEDs. | |
void | blinkRate (uint8_t b) |
Set blink rate of LEDs. | |
void | writeDisplay (void) |
Write to LEDs after set and clear status of LEDs. | |
void | clear (void) |
Set all LEDs to off. | |
bool | isLED (uint8_t x) |
Check status of LED. | |
void | setLED (uint8_t x) |
Set LED status to on. | |
void | clrLED (uint8_t x) |
Set LED status to off. | |
bool | isKeyPressed (uint8_t k) |
Check if button is pressed. | |
bool | wasKeyPressed (uint8_t k) |
Check if button was pressed. | |
bool | readSwitches (void) |
Update all buttons' status, need to be called before checking buttons' status. | |
bool | justPressed (uint8_t k) |
Check if button was just pressed. | |
bool | justReleased (uint8_t k) |
Check if button was just released. |
Detailed Description
A class for Adafruit trellis.
Definition at line 18 of file Adafruit_Trellis.h.
Constructor & Destructor Documentation
Adafruit_Trellis | ( | void | ) |
Create a new trellis object.
Definition at line 24 of file Adafruit_Trellis.cpp.
Member Function Documentation
void begin | ( | I2C * | _wire, |
uint8_t | _addr = 0x70 |
||
) |
Initialize the trellis controller.
- Parameters:
-
_wire Address of an I2C master object _addr Slave address of the trellis controller
Definition at line 27 of file Adafruit_Trellis.cpp.
void blinkRate | ( | uint8_t | b ) |
Set blink rate of LEDs.
- Parameters:
-
b Blink rate defined as macros
Definition at line 122 of file Adafruit_Trellis.cpp.
void clear | ( | void | ) |
Set all LEDs to off.
Definition at line 139 of file Adafruit_Trellis.cpp.
void clrLED | ( | uint8_t | x ) |
Set LED status to off.
- Parameters:
-
x LED index (1-16)
Definition at line 83 of file Adafruit_Trellis.cpp.
bool isKeyPressed | ( | uint8_t | k ) |
Check if button is pressed.
- Parameters:
-
k button index (1-16)
- Returns:
- 1 for pressed, 0 for not pressed
Definition at line 50 of file Adafruit_Trellis.cpp.
bool isLED | ( | uint8_t | x ) |
Check status of LED.
- Parameters:
-
x LED index (1-16)
- Returns:
- 1 for on, 0 for off
Definition at line 73 of file Adafruit_Trellis.cpp.
bool justPressed | ( | uint8_t | k ) |
Check if button was just pressed.
- Parameters:
-
k button index (1-16)
- Returns:
- 1 for pressed, 0 for not pressed
Definition at line 61 of file Adafruit_Trellis.cpp.
bool justReleased | ( | uint8_t | k ) |
Check if button was just released.
- Parameters:
-
k button index (1-16)
- Returns:
- 1 for released, 0 for not released
Definition at line 64 of file Adafruit_Trellis.cpp.
bool readSwitches | ( | void | ) |
Update all buttons' status, need to be called before checking buttons' status.
- Returns:
- 1 for any button has been switched, 0 otherwise
Definition at line 94 of file Adafruit_Trellis.cpp.
void setBrightness | ( | uint8_t | b ) |
Set brightness(PWM) of LEDs.
- Parameters:
-
b Brightness level from 1 to 16 with 16 being the brightest
Definition at line 116 of file Adafruit_Trellis.cpp.
void setLED | ( | uint8_t | x ) |
Set LED status to on.
- Parameters:
-
x LED index (1-16)
Definition at line 78 of file Adafruit_Trellis.cpp.
bool wasKeyPressed | ( | uint8_t | k ) |
Check if button was pressed.
- Parameters:
-
k button index (1-16)
- Returns:
- 1 for pressed, 0 for not pressed
Definition at line 55 of file Adafruit_Trellis.cpp.
void writeDisplay | ( | void | ) |
Write to LEDs after set and clear status of LEDs.
Definition at line 129 of file Adafruit_Trellis.cpp.
Generated on Wed Jul 13 2022 18:58:39 by
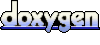