This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
RSA encrypt/decrypt functions
[RayCryptoApi]
Typedefs | |
typedef U8 * | SharkSslRSAKey |
SharkSslRSAKey is an alias for the SharkSslCert type and is a private/public key converted by sharkssl_PEM_to_RSAKey or the command line tool [SharkSslParseKey](SharkSslParseKey). | |
Functions | |
SHARKSSL_API SharkSslRSAKey | sharkssl_PEM_to_RSAKey (const char *PEMKey, const char *passphrase) |
Convert an RSA private or public key in PEM format to the SharkSslRSAKey format. | |
SHARKSSL_API SharkSslRSAKey | sharkssl_PEM_extractPublicKey (const char *certPEM) |
Extract the public key form a certificate in PEM format. | |
SHARKSSL_API void | SharkSslRSAKey_free (SharkSslRSAKey key) |
Release a SharkSslRSAKey allocated by functions sharkssl_PEM_to_RSAKey and sharkssl_PEM_extractPublicKey. | |
SHARKSSL_API U16 | SharkSslRSAKey_size (SharkSslRSAKey key) |
Returns the private or public key's modulus size in bytes. | |
SHARKSSL_API sharkssl_RSA_RetVal | sharkssl_RSA_public_encrypt (U16 len, U8 *in, U8 *out, SharkSslRSAKey key, U8 padding) |
Encrypt data using the public key or private key. | |
SHARKSSL_API sharkssl_RSA_RetVal | sharkssl_RSA_private_decrypt (U16 len, U8 *in, U8 *out, SharkSslRSAKey privkey, U8 padding) |
Decrypt ciphertext using the private key. | |
SHARKSSL_API sharkssl_RSA_RetVal | sharkssl_RSA_private_encrypt (U16 len, U8 *in, U8 *out, SharkSslRSAKey privkey, U8 padding) |
Sign a message digest using the private key. | |
SHARKSSL_API sharkssl_RSA_RetVal | sharkssl_RSA_public_decrypt (U16 len, U8 *in, U8 *out, SharkSslRSAKey key, U8 padding) |
Bring back a message digest using the public key or private key. |
Typedef Documentation
typedef U8* SharkSslRSAKey |
SharkSslRSAKey is an alias for the SharkSslCert type and is a private/public key converted by sharkssl_PEM_to_RSAKey or the command line tool [SharkSslParseKey](SharkSslParseKey).
Definition at line 1637 of file SharkSSL.h.
Function Documentation
SHARKSSL_API SharkSslRSAKey sharkssl_PEM_extractPublicKey | ( | const char * | certPEM ) |
Extract the public key form a certificate in PEM format.
Note: the converted value must be released by calling SharkSslRSAKey_free, when no longer needed.
example:
{ SharkSslRSAKey RSAKey; ... RSAKey = sharkssl_PEM_extractPublicKey(cert); if (RSAKey) { ... void SharkSslRSAKey_free(RSAKey); } }
- Returns:
- the certificate's public key in SharkSslRSAKey format or NULL if the conversion fails.
SHARKSSL_API SharkSslRSAKey sharkssl_PEM_to_RSAKey | ( | const char * | PEMKey, |
const char * | passphrase | ||
) |
Convert an RSA private or public key in PEM format to the SharkSslRSAKey format.
Note: the converted value must be released by calling SharkSslRSAKey_free, when no longer needed.
example:
{ SharkSslRSAKey RSAKey; ... RSAKey = sharksslPEM_to_RSAKey(key, pass); if (RSAKey) { ... void SharkSslRSAKey_free(RSAKey); } }
- Returns:
- the private/public key in SharkSslRSAKey format or NULL if the conversion fails.
SHARKSSL_API sharkssl_RSA_RetVal sharkssl_RSA_private_decrypt | ( | U16 | len, |
U8 * | in, | ||
U8 * | out, | ||
SharkSslRSAKey | privkey, | ||
U8 | padding | ||
) |
Decrypt ciphertext using the private key.
- Parameters:
-
len is the length/size of parameter 'in'. This length must be exactly SharkSslRSAKey_size (key). in the ciphertext out the decrypted ciphertext is copied to this buffer. The size of this buffer must be no less than SharkSslRSAKey_size (key) privkey is the private key in SharkSslRSAKey format. padding is one of SHARKSSL_RSA_PKCS1_PADDING or SHARKSSL_RSA_NO_PADDING
- Returns:
- the size of the decrypted ciphertext, or -1 if any error occurs
SHARKSSL_API sharkssl_RSA_RetVal sharkssl_RSA_private_encrypt | ( | U16 | len, |
U8 * | in, | ||
U8 * | out, | ||
SharkSslRSAKey | privkey, | ||
U8 | padding | ||
) |
Sign a message digest using the private key.
- Parameters:
-
in commonly, an algorithm identifier followed by a message digest len is the length/size of parameter 'in'. This length must be exactly SharkSslRSAKey_size (key) when selecting SHARKSSL_RSA_NO_PADDING or a value between 1 and (SharkSslRSAKey_size (key) - 11) when selecting SHARKSSL_RSA_PKCS1_PADDING. out the signature is copied to this buffer. The size of this buffer must be no less than SharkSslRSAKey_size (key) privkey is the private key in SharkSslRSAKey format. padding is one of SHARKSSL_RSA_PKCS1_PADDING or SHARKSSL_RSA_NO_PADDING
- Returns:
- the size of the signature, or -1 if any error occurs
SHARKSSL_API sharkssl_RSA_RetVal sharkssl_RSA_public_decrypt | ( | U16 | len, |
U8 * | in, | ||
U8 * | out, | ||
SharkSslRSAKey | key, | ||
U8 | padding | ||
) |
Bring back a message digest using the public key or private key.
The private key includes the public key an can for this reason be used for this operation.
- Parameters:
-
in the RSA signature len is the length/size of parameter 'in'. This length must be exactly SharkSslRSAKey_size (key). out the message digest is copied to this buffer. The size of this buffer must be no less than SharkSslRSAKey_size (key) key is the public key in SharkSslRSAKey format. padding is one of SHARKSSL_RSA_PKCS1_PADDING or SHARKSSL_RSA_NO_PADDING
- Returns:
- the size of the obtained message digest, or -1 if any error occurs
SHARKSSL_API sharkssl_RSA_RetVal sharkssl_RSA_public_encrypt | ( | U16 | len, |
U8 * | in, | ||
U8 * | out, | ||
SharkSslRSAKey | key, | ||
U8 | padding | ||
) |
Encrypt data using the public key or private key.
The private key includes the public key an can for this reason be used for encrypting the data.
- Parameters:
-
in the plaintext len is the length/size of parameter 'in'. This length must be exactly SharkSslRSAKey_size (key) when selecting SHARKSSL_RSA_NO_PADDING or a value between 1 and (SharkSslRSAKey_size (key) - 11) when selecting SHARKSSL_RSA_PKCS1_PADDING. out the encrypted ciphertext is copied to this buffer. The size of this buffer must be no less than SharkSslRSAKey_size (key) key is the public key in SharkSslRSAKey format. padding is one of SHARKSSL_RSA_PKCS1_PADDING or SHARKSSL_RSA_NO_PADDING
- Returns:
- the size of the encrypted ciphertext, or -1 if any error occurs
SHARKSSL_API void SharkSslRSAKey_free | ( | SharkSslRSAKey | key ) |
Release a SharkSslRSAKey allocated by functions sharkssl_PEM_to_RSAKey and sharkssl_PEM_extractPublicKey.
SHARKSSL_API U16 SharkSslRSAKey_size | ( | SharkSslRSAKey | key ) |
Returns the private or public key's modulus size in bytes.
Generated on Wed Jul 13 2022 10:54:53 by
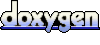