Alphanumeric LED display, 8 digits, 5x7 pattern. Supports 8x1, 8x2, 16x1 and 16x2 display configuration.
HCMS2975 Class Reference
A library for driving HCMS2975 LED matrix displays. More...
#include <HCMS2975.h>
Public Types | |
enum | LEDType { LED8x1 = (LED_T_N1 | LED_T_C8 | LED_T_R1), LED8x2 = (LED_T_N2 | LED_T_C8 | LED_T_R2), LED16x1 = (LED_T_N2 | LED_T_C16 | LED_T_R1), LED16x2 = (LED_T_N4 | LED_T_C16 | LED_T_R2) } |
LED panel format. More... | |
enum | DisplayMode { DispOff, DispOn } |
Display control. More... | |
Public Member Functions | |
HCMS2975 (SPI *spi, PinName cs, PinName rs, PinName rst=NC, LEDType type=LED8x1) | |
Create an HCMS2975 Display object connected to the proper pins. | |
virtual | ~HCMS2975 () |
Destructor for HCMS2975 Display object. | |
int | putc (int c) |
Write a character to the Display. | |
int | printf (const char *text,...) |
Write a raw string to the Display. | |
int | putc (int c) |
Write a character to the Display. | |
int | printf (const char *format,...) |
Write a formatted string to the Display. | |
void | cls () |
Clear the screen and locate to 0,0. | |
void | locate (int column=0, int row=0) |
Locate cursor to a screen column and row. | |
int | columns () |
Return the number of columns. | |
int | rows () |
Return the number of rows. | |
void | setMode (DisplayMode displayMode) |
Set the Displaymode. | |
void | setBrightness (uint8_t brightness=HCMS2975_DEF_BRIGHT) |
Set Brightness. | |
void | setUDC (unsigned char c, char *udc_data) |
Set User Defined Characters (UDC) | |
int | getVersion () |
Returns the version number of the library. | |
Protected Member Functions | |
void | _reset () |
Low level Reset method for controller. | |
void | _init () |
Low level Init method for LED controller. | |
void | _writeCommand (uint8_t command) |
Low level command byte write operation. | |
void | _pushBuffer () |
Low level _dataBuffer write operation. |
Detailed Description
A library for driving HCMS2975 LED matrix displays.
Currently supports 8x1, 8x2, 16x1, 16x2
#include "mbed.h" #include "HCMS2975.h" // SPI Communication SPI spi_led(p5, NC, p7); // MOSI, MISO, SCLK //Display HCMS2975 led(&spi_led, p8, p9, p10); // SPI bus, CS pin, RS pin, Rst pin, LEDType = default int main() { int cnt; led.locate(0, 0); // 12345678 led.printf("Hi mbed "); wait(2); cnt=0x20; while(1) { wait(0.5); led.putc(cnt); cnt++; if (cnt == 0x80) cnt=0x20; } }
Create an HCMS2975 Display object connected to the proper pins
- Parameters:
-
*spi SPI port cs PinName for Chip Select (active low) rs PinName for RS (Data = low, Command = High) rst PinName for Rst (active low, optional, default=NC) type Sets the panel size (default = LED8x1)
Definition at line 175 of file HCMS2975.h.
Member Enumeration Documentation
enum DisplayMode |
Display control.
Definition at line 195 of file HCMS2975.h.
enum LEDType |
LED panel format.
- Enumerator:
LED8x1 8x1 LED panel (default)
LED8x2 8x2 LED panel
LED16x1 16x1 LED panel
LED16x2 16x2 LED panel
Definition at line 187 of file HCMS2975.h.
Constructor & Destructor Documentation
Create an HCMS2975 Display object connected to the proper pins.
- Parameters:
-
*spi SPI port cs PinName for Chip Select (active low) rs PinName for RS () rst PinName for Rst (active low, optional, default = NC) type Sets the panel size (default = LED8x1) *spi SPI port cs PinName for Chip Select (active low) rs PinName for RS () rst PinName for Rst (active low, optional, default=NC) type Sets the panel size (default = LED8x1)
Definition at line 65 of file HCMS2975.cpp.
~HCMS2975 | ( | ) | [virtual] |
Destructor for HCMS2975 Display object.
- Parameters:
-
none
- Returns:
- none
Definition at line 97 of file HCMS2975.cpp.
Member Function Documentation
void _init | ( | ) | [protected] |
Low level Init method for LED controller.
Low level Init method for controller.
Definition at line 284 of file HCMS2975.cpp.
void _pushBuffer | ( | ) | [protected] |
Low level _dataBuffer write operation.
Definition at line 345 of file HCMS2975.cpp.
void _reset | ( | ) | [protected] |
Low level Reset method for controller.
Definition at line 272 of file HCMS2975.cpp.
void _writeCommand | ( | uint8_t | command ) | [protected] |
Low level command byte write operation.
- Parameters:
-
command commandbyte to write
Definition at line 317 of file HCMS2975.cpp.
void cls | ( | ) |
Clear the screen and locate to 0,0.
Definition at line 146 of file HCMS2975.cpp.
int columns | ( | ) |
Return the number of columns.
- Returns:
- int The number of columns
Definition at line 199 of file HCMS2975.cpp.
int getVersion | ( | ) |
Returns the version number of the library.
- Returns:
- int version number
Definition at line 440 of file HCMS2975.cpp.
void locate | ( | int | column = 0 , |
int | row = 0 |
||
) |
Locate cursor to a screen column and row.
- Parameters:
-
column The horizontal position from the left, indexed from 0 row The vertical position from the top, indexed from 0
Definition at line 166 of file HCMS2975.cpp.
int printf | ( | const char * | format, |
... | |||
) |
Write a raw string to the Display.
Write a formatted string to the Display.
- Parameters:
-
string text, may be followed by variables to emulate formatting the string. However, when (HCMS2975_PRINTF != 1) then printf formatting is NOT supported and variables will be ignored! string text, may be followed by variables to emulate formatting the string. However, printf formatting is NOT supported and variables will be ignored! format A printf-style format string, followed by the variables to use in formatting the string.
Definition at line 115 of file HCMS2975.cpp.
int printf | ( | const char * | format, |
... | |||
) |
Write a formatted string to the Display.
- Parameters:
-
format A printf-style format string, followed by the variables to use in formatting the string.
int putc | ( | int | c ) |
Write a character to the Display.
- Parameters:
-
c The character to write to the display
Definition at line 106 of file HCMS2975.cpp.
int putc | ( | int | c ) |
Write a character to the Display.
- Parameters:
-
c The character to write to the display
int rows | ( | ) |
Return the number of rows.
- Returns:
- int The number of rows
Definition at line 210 of file HCMS2975.cpp.
void setBrightness | ( | uint8_t | brightness = HCMS2975_DEF_BRIGHT ) |
Set Brightness.
- Parameters:
-
brightness The brightness level (valid range 0..15)
Definition at line 222 of file HCMS2975.cpp.
void setMode | ( | DisplayMode | displayMode ) |
Set the Displaymode.
- Parameters:
-
displayMode The Display mode (DispOff, DispOn)
Definition at line 235 of file HCMS2975.cpp.
void setUDC | ( | unsigned char | c, |
char * | udc_data | ||
) |
Set User Defined Characters (UDC)
- Parameters:
-
unsigned char c The Index of the UDC (0..7) char *udc_data The bitpatterns for the UDC (5 bytes of 7 significant bits for bitpattern)
Definition at line 255 of file HCMS2975.cpp.
Generated on Fri Jul 15 2022 04:36:08 by
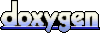