Alphanumeric LED display, 8 digits, 5x7 pattern. Supports 8x1, 8x2, 16x1 and 16x2 display configuration.
Embed:
(wiki syntax)
Show/hide line numbers
HCMS2975.h
Go to the documentation of this file.
00001 /** 00002 * @file HCMS2975.h 00003 * @brief mbed Avago/HP HCSM2975 LED matrix display Library. 00004 * @author WH 00005 * @date Copyright (c) 2014 00006 * v01: WH, Initial release, 00007 * Info available at http://playground.arduino.cc/Main/LedDisplay and http://www.pjrc.com/teensy/td_libs_LedDisplay.html 00008 * v02: WH, added getVersion(), 00009 * v03: WH, fixed setBrightness at init() when there is no HW reset. 00010 * 00011 * Permission is hereby granted, free of charge, to any person obtaining a copy 00012 * of this software and associated documentation files (the "Software"), to deal 00013 * in the Software without restriction, including without limitation the rights 00014 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00015 * copies of the Software, and to permit persons to whom the Software is 00016 * furnished to do so, subject to the following conditions: 00017 * 00018 * The above copyright notice and this permission notice shall be included in 00019 * all copies or substantial portions of the Software. 00020 * 00021 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00022 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00023 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00024 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00025 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00026 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00027 * THE SOFTWARE. 00028 */ 00029 #ifndef _HCMS2975_H 00030 #define _HCMS2975_H 00031 00032 #include "mbed.h" 00033 00034 //Enable Stream printf or minimalistic printf 00035 #define HCMS2975_PRINTF 1 00036 00037 #define HCMS2975_VERSION 2 00038 00039 //Max supported display is 16x2 00040 #define HCMS2975_BUFFER_SIZE 32 00041 //#define HCMS2975_BUFFER_SIZE 8 00042 00043 /* LED Type information on Rows and Columns. This information is encoded in 00044 * an int and used for the LEDType enumerators in order to simplify code maintenance */ 00045 // Columns encoded in b7..b0 00046 #define LED_T_COL_MSK 0x000000FF 00047 #define LED_T_C8 0x00000008 00048 #define LED_T_C16 0x00000010 00049 00050 // Rows encoded in b15..b8 00051 #define LED_T_ROW_MSK 0x0000FF00 00052 #define LED_T_R1 0x00000100 00053 #define LED_T_R2 0x00000200 00054 #define LED_T_R3 0x00000300 00055 #define LED_T_R4 0x00000400 00056 00057 /* LED number of devices. This information is encoded in 00058 * an int and used for the command and databyte transmission counter in order to simplify code maintenance */ 00059 // Number of Devices encoded in b23..16 00060 #define LED_T_CNT_MSK 0x00FF0000 00061 #define LED_T_N1 0x00010000 00062 #define LED_T_N2 0x00020000 00063 #define LED_T_N4 0x00040000 00064 00065 00066 // Controlword 0 00067 #define HCMS2975_CONTROL0 0x00 00068 00069 // D6 is /Sleep 00070 #define HCMS2975_SLP 0x00 00071 #define HCMS2975_NORMAL 0x40 00072 00073 // D4.D5 selects max peak current 00074 #define HCMS2975_PEAK_12_8 0x30 00075 #define HCMS2975_PEAK_9_3 0x00 00076 #define HCMS2975_PEAK_6_4 0x10 00077 #define HCMS2975_PEAK_4_0 0x20 00078 00079 // display brightness definitions, indicating percentage brightness 00080 // D3..D0 select brightness 00081 #define HCMS2975_BRIGHT_100 0x0F 00082 #define HCMS2975_BRIGHT_80 0x0E 00083 #define HCMS2975_BRIGHT_60 0x0D 00084 #define HCMS2975_BRIGHT_47 0x0C 00085 #define HCMS2975_BRIGHT_37 0x0B 00086 #define HCMS2975_BRIGHT_30 0x0A 00087 #define HCMS2975_BRIGHT_23 0x09 00088 #define HCMS2975_BRIGHT_18 0x08 00089 #define HCMS2975_BRIGHT_15 0x07 00090 #define HCMS2975_BRIGHT_11_7 0x06 00091 #define HCMS2975_BRIGHT_8_3 0x05 00092 #define HCMS2975_BRIGHT_6_7 0x04 00093 #define HCMS2975_BRIGHT_5 0x03 00094 #define HCMS2975_BRIGHT_3_3 0x02 00095 #define HCMS2975_BRIGHT_1_7 0x01 00096 #define HCMS2975_BRIGHT_0 0x00 00097 00098 // default display brightness 00099 #define HCMS2975_DEF_BRIGHT HCMS2975_BRIGHT_30 00100 #define HCMS2975_DEF_PEAK HCMS2975_PEAK_6_4 00101 00102 // Controlword 1 00103 #define HCMS2975_CONTROL1 0x80 00104 00105 //Select Prescaler mode 00106 #define HCMS2975_PRE_1 0x00 00107 #define HCMS2975_PRE_8 0x02 00108 00109 //Select Serial/Simultaneous mode 00110 #define HCMS2975_SERIAL 0x00 00111 #define HCMS2975_SIMUL 0x01 00112 00113 // Write Digit columns data as 5 sequential bytes. 00114 // First byte defines left column. D7 always 0, D6..D0 define bitpattern. D6 is downmost, D0 is topmost pixel. 00115 // 00116 extern const char udc_0[]; /* |> */ 00117 extern const char udc_1[]; /* <| */ 00118 extern const char udc_2[]; /* | */ 00119 extern const char udc_3[]; /* || */ 00120 extern const char udc_4[]; /* ||| */ 00121 extern const char udc_5[]; /* = */ 00122 extern const char udc_6[]; /* checkerboard */ 00123 extern const char udc_7[]; /* \ */ 00124 00125 extern const char udc_Bat_Hi[]; /* Battery Full */ 00126 extern const char udc_Bat_Ha[]; /* Battery Half */ 00127 extern const char udc_Bat_Lo[]; /* Battery Low */ 00128 extern const char udc_AC[]; /* AC Power */ 00129 extern const char udc_smiley[]; /* Smiley */ 00130 00131 /** A library for driving HCMS2975 LED matrix displays 00132 * @brief Currently supports 8x1, 8x2, 16x1, 16x2 00133 * 00134 * 00135 * @code 00136 * #include "mbed.h" 00137 * #include "HCMS2975.h" 00138 * 00139 * // SPI Communication 00140 * SPI spi_led(p5, NC, p7); // MOSI, MISO, SCLK 00141 * 00142 * //Display 00143 * HCMS2975 led(&spi_led, p8, p9, p10); // SPI bus, CS pin, RS pin, Rst pin, LEDType = default 00144 * 00145 * int main() { 00146 * int cnt; 00147 * 00148 * led.locate(0, 0); 00149 * 00150 * // 12345678 00151 * led.printf("Hi mbed "); 00152 * wait(2); 00153 * 00154 * cnt=0x20; 00155 * while(1) { 00156 * wait(0.5); 00157 * 00158 * led.putc(cnt); 00159 * cnt++; 00160 * if (cnt == 0x80) cnt=0x20; 00161 * } 00162 * } 00163 * @endcode 00164 */ 00165 00166 00167 /** Create an HCMS2975 Display object connected to the proper pins 00168 * 00169 * @param *spi SPI port 00170 * @param cs PinName for Chip Select (active low) 00171 * @param rs PinName for RS (Data = low, Command = High) 00172 * @param rst PinName for Rst (active low, optional, default=NC) 00173 * @param type Sets the panel size (default = LED8x1) 00174 */ 00175 class HCMS2975 : public Stream { 00176 //class HCMS2975 { 00177 00178 //Unfortunately this #define selection breaks Doxygen !!! 00179 //#if (HCMS2975_PRINTF == 1) 00180 //class HCMS2975 : public Stream { 00181 //#else 00182 //class HCMS2975 { 00183 //#endif 00184 00185 public: 00186 /** LED panel format */ 00187 enum LEDType { 00188 LED8x1 = (LED_T_N1 | LED_T_C8 | LED_T_R1), /**< 8x1 LED panel (default) */ 00189 LED8x2 = (LED_T_N2 | LED_T_C8 | LED_T_R2), /**< 8x2 LED panel */ 00190 LED16x1 = (LED_T_N2 | LED_T_C16 | LED_T_R1), /**< 16x1 LED panel */ 00191 LED16x2 = (LED_T_N4 | LED_T_C16 | LED_T_R2), /**< 16x2 LED panel */ 00192 }; 00193 00194 /** Display control */ 00195 enum DisplayMode { 00196 DispOff, /**< Display Off */ 00197 DispOn /**< Display On */ 00198 }; 00199 00200 /** Create an HCMS2975 Display object connected to the proper pins 00201 * 00202 * @param *spi SPI port 00203 * @param cs PinName for Chip Select (active low) 00204 * @param rs PinName for RS () 00205 * @param rst PinName for Rst (active low, optional, default = NC) 00206 * @param type Sets the panel size (default = LED8x1) 00207 */ 00208 HCMS2975(SPI *spi, PinName cs, PinName rs, PinName rst = NC, LEDType type = LED8x1); 00209 00210 00211 /** Destructor for HCMS2975 Display object 00212 * 00213 * @param none 00214 * @return none 00215 */ 00216 virtual ~HCMS2975(); 00217 00218 #if (HCMS2975_PRINTF != 1) 00219 /** Write a character to the Display 00220 * 00221 * @param c The character to write to the display 00222 */ 00223 int putc(int c); 00224 00225 /** Write a raw string to the Display 00226 * 00227 * @param string text, may be followed by variables to emulate formatting the string. 00228 * However, when (HCMS2975_PRINTF != 1) then printf formatting is NOT supported and variables will be ignored! 00229 */ 00230 int printf(const char* text, ...); 00231 #else 00232 #if DOXYGEN_ONLY 00233 /** Write a character to the Display 00234 * 00235 * @param c The character to write to the display 00236 */ 00237 int putc(int c); 00238 00239 /** Write a formatted string to the Display 00240 * 00241 * @param format A printf-style format string, followed by the 00242 * variables to use in formatting the string. 00243 */ 00244 int printf(const char* format, ...); 00245 #endif 00246 00247 #endif 00248 00249 /** Clear the screen and locate to 0,0 00250 */ 00251 void cls(); 00252 00253 /** Locate cursor to a screen column and row 00254 * 00255 * @param column The horizontal position from the left, indexed from 0 00256 * @param row The vertical position from the top, indexed from 0 00257 */ 00258 void locate(int column=0, int row=0); 00259 00260 /** Return the number of columns 00261 * 00262 * @return int The number of columns 00263 */ 00264 int columns(); 00265 00266 /** Return the number of rows 00267 * 00268 * @return int The number of rows 00269 */ 00270 int rows(); 00271 00272 /** Set the Displaymode 00273 * 00274 * @param displayMode The Display mode (DispOff, DispOn) 00275 */ 00276 void setMode(DisplayMode displayMode); 00277 00278 /** Set Brightness 00279 * 00280 * @param brightness The brightness level (valid range 0..15) 00281 */ 00282 void setBrightness(uint8_t brightness = HCMS2975_DEF_BRIGHT); 00283 00284 /** Set User Defined Characters (UDC) 00285 * 00286 * @param unsigned char c The Index of the UDC (0..7) 00287 * @param char *udc_data The bitpatterns for the UDC (5 bytes of 7 significant bits for bitpattern) 00288 */ 00289 void setUDC(unsigned char c, char *udc_data); 00290 00291 00292 /** Returns the version number of the library 00293 * @return int version number 00294 */ 00295 int getVersion(); 00296 00297 protected: 00298 /** Low level Reset method for controller 00299 */ 00300 void _reset(); 00301 00302 /** Low level Init method for LED controller 00303 */ 00304 void _init(); 00305 00306 /** Low level command byte write operation. 00307 * @param command commandbyte to write 00308 */ 00309 void _writeCommand(uint8_t command); 00310 00311 /** Low level _dataBuffer write operation. 00312 */ 00313 void _pushBuffer(); 00314 00315 // Stream implementation functions 00316 virtual int _putc(int value); 00317 virtual int _getc(); 00318 00319 //SPI bus 00320 SPI *_spi; 00321 DigitalOut _cs; 00322 00323 DigitalOut _rs; 00324 DigitalOut *_rst; 00325 00326 //Display type 00327 LEDType _type; 00328 int _nr_cols; 00329 int _nr_rows; 00330 00331 int _row; 00332 int _column; 00333 int _peak, _brightness; 00334 00335 // _displayBuffer 00336 char _displayBuffer[HCMS2975_BUFFER_SIZE]; 00337 int _displaySize; 00338 int _deviceCount; 00339 00340 // Local UDC memory 00341 char _udc[8][5]; 00342 }; 00343 00344 00345 #endif
Generated on Fri Jul 15 2022 04:36:08 by
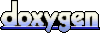