
test
Dependencies: BLE_API mbed nRF51822
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ble/BLE.h" 00019 00020 Ticker timer; 00021 00022 DigitalIn myInputPin(P0_10); 00023 00024 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00025 00026 /* Optional: Device Name, add for human read-ability */ 00027 00028 const static char DEVICE_NAME[] = "BLE_BEACON_TE"; 00029 00030 00031 /* You have up to 26 bytes of advertising data to use. */ 00032 uint8_t AdvData[] = {0x01,0x02,0x00,0x04,0x12}; /* Example of hex data */ 00033 //const static uint8_t AdvData[] = {"ChangeThisData"}; /* Example of character data */ 00034 00035 00036 class AdvertisementPacket { 00037 public: 00038 AdvertisementPacket(BLE&); 00039 void updateAdvertisementPacket(){ 00040 00041 AdvData[1] = myInputPin; 00042 00043 ble.gap().clearAdvertisingPayload(); 00044 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE ); 00045 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, AdvData, sizeof(AdvData)); 00046 }; 00047 private: 00048 BLE &ble; 00049 }; 00050 00051 AdvertisementPacket::AdvertisementPacket(BLE &bleIn): ble(bleIn){ 00052 00053 }; 00054 /* 00055 00056 /* Optional: Restart advertising when peer disconnects */ 00057 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00058 { 00059 BLE::Instance().gap().startAdvertising(); 00060 } 00061 /** 00062 * This function is called when the ble initialization process has failed 00063 */ 00064 void onBleInitError(BLE &ble, ble_error_t error) 00065 { 00066 /* Avoid compiler warnings */ 00067 (void) ble; 00068 (void) error; 00069 00070 /* Initialization error handling should go here */ 00071 } 00072 00073 /** 00074 * Callback triggered when the ble initialization process has finished 00075 */ 00076 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00077 { 00078 BLE& ble = params->ble; 00079 ble_error_t error = params->error; 00080 00081 if (error != BLE_ERROR_NONE) { 00082 /* In case of error, forward the error handling to onBleInitError */ 00083 onBleInitError(ble, error); 00084 return; 00085 } 00086 00087 /* Ensure that it is the default instance of BLE */ 00088 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00089 return; 00090 } 00091 00092 /* Set device name characteristic data */ 00093 ble.gap().setDeviceName((const uint8_t *) DEVICE_NAME); 00094 00095 /* Optional: add callback for disconnection */ 00096 ble.gap().onDisconnection(disconnectionCallback); 00097 00098 /* Sacrifice 3B of 31B to Advertising Flags */ 00099 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE ); 00100 //ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00101 00102 00103 /* Configure advertisements */ 00104 //ble.gap().setTxPower(radioPowerLevels[txPowerMode]); 00105 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00106 //ble.gap().setAdvertisingInterval(beaconPeriod); 00107 //ble.gap().onRadioNotification(this, &EddystoneService::radioNotificationCallback); 00108 //ble.gap().initRadioNotification(); 00109 00110 00111 /* Sacrifice 2B of 31B to AdvType overhead, rest goes to AdvData array you define */ 00112 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, AdvData, sizeof(AdvData)); 00113 00114 /* Optional: Add name to device */ 00115 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00116 00117 /* Set advertising interval. Longer interval == longer battery life */ 00118 ble.gap().setAdvertisingInterval(1000); /* 100ms */ 00119 00120 ble.gap().clearAdvertisingPayload(); 00121 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE ); 00122 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, AdvData, sizeof(AdvData)); 00123 00124 00125 00126 /* Start advertising */ 00127 ble.gap().startAdvertising(); 00128 } 00129 00130 int main(void) 00131 { 00132 00133 00134 00135 00136 /* Initialize BLE baselayer, always do this first! */ 00137 ble.init(bleInitComplete); 00138 00139 /* SpinWait for initialization to complete. This is necessary because the 00140 * BLE object is used in the main loop below. */ 00141 while (!ble.hasInitialized()) { /* spin loop */ } 00142 00143 AdvertisementPacket f(ble); 00144 00145 timer.attach( &f, &AdvertisementPacket::updateAdvertisementPacket, 1.0); 00146 00147 while (true) { 00148 ble.waitForEvent(); 00149 } 00150 }
Generated on Tue Jul 19 2022 23:07:34 by
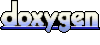