
Controlador da Panificador
Dependencies: BLE_API mbed nRF51822
Fork of BLENano_SimpleControls by
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 #include "mbed.h" 00021 #include "ble/BLE.h" 00022 #include "Servo.h" 00023 00024 00025 #define TXRX_BUF_LEN 20 00026 00027 #define PIN_TIME_MORE P0_4 00028 #define PIN_TIME_LESS P0_5 00029 #define PIN_DOUGH_QNT P0_8 00030 #define PIN_OPTIONS P0_9 00031 #define PIN_COLOR P0_10 00032 #define PIN_INIT_STOP P0_11 00033 #define PIN_TEST_LED P0_19 // only used for debuggin (visual check) 00034 00035 #define OPERATION 0x00 00036 #define COMMAND 0x01 00037 #define PROGRAM 0x02 00038 00039 #define TIME_MORE 0x04 00040 #define TIME_LESS 0x05 00041 #define DOUGH_QNT 0x02 00042 #define INIT_STOP 0x06 00043 #define OPTIONS 0x01 00044 #define COLOR 0x03 00045 #define TEST_LED 0x07 00046 00047 #define DELAY 250 // 250 ms holding the up state for button clicking 00048 00049 BLE ble; 00050 00051 DigitalOut time_more(PIN_TIME_MORE,0); 00052 DigitalOut time_less(PIN_TIME_LESS,0); 00053 DigitalOut dough_qnt(PIN_DOUGH_QNT,0); 00054 DigitalOut init_stop(PIN_INIT_STOP,0); 00055 DigitalOut options(PIN_OPTIONS,0); 00056 DigitalOut color(PIN_COLOR,0); 00057 DigitalOut test_led(PIN_TEST_LED,1); 00058 00059 /* The Nordic UART Service */ 00060 00061 // RX_SERVICE_UUID do APP Android 00062 static const uint8_t uart_base_uuid[] = {0x6E, 0x40, 0x00, 0x01, 0xB5, 0xA3, 0xF3, 0x93, 0xE0, 0xA9, 0xE5, 0x0E, 0x24, 0xDC, 0xCA, 0x9E}; 00063 //TX_CHAR_UUID do APP Android 00064 static const uint8_t uart_tx_uuid[] = {0x6E, 0x40, 0x00, 0x02, 0xB5, 0xA3, 0xF3, 0x93, 0xE0, 0xA9, 0xE5, 0x0E, 0x24, 0xDC, 0xCA, 0x9E}; 00065 //RX_CHAR_UUID do APP Android 00066 static const uint8_t uart_rx_uuid[] = {0x6E, 0x40, 0x00, 0x03, 0xB5, 0xA3, 0xF3, 0x93, 0xE0, 0xA9, 0xE5, 0x0E, 0x24, 0xDC, 0xCA, 0x9E}; 00067 // RX_SERVICE_UUID do APP Android 00068 static const uint8_t uart_base_uuid_rev[] = {0x6E, 0x40, 0x00, 0x01, 0xB5, 0xA3, 0xF3, 0x93, 0xE0, 0xA9, 0xE5, 0x0E, 0x24, 0xDC, 0xCA, 0x9E}; 00069 00070 00071 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00072 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00073 00074 //static uint8_t rx_buf[TXRX_BUF_LEN]; 00075 //static uint8_t rx_len=0; 00076 00077 00078 GattCharacteristic txCharacteristic (uart_tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00079 00080 GattCharacteristic rxCharacteristic (uart_rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00081 00082 GattCharacteristic *uartChars[] = {&txCharacteristic, &rxCharacteristic}; 00083 00084 GattService uartService(uart_base_uuid, uartChars, sizeof(uartChars) / sizeof(GattCharacteristic *)); 00085 00086 00087 00088 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00089 { 00090 //pc.printf("Disconnected \r\n"); 00091 //pc.printf("Restart advertising \r\n"); 00092 //ble.gap().startAdvertising(); 00093 ble.startAdvertising(); 00094 } 00095 00096 void ClickButton(DigitalOut *button, uint8_t *click_num) 00097 { 00098 for (uint8_t i = *click_num; i > 0; i--) 00099 { 00100 *button = 0; 00101 wait_ms(DELAY); 00102 *button = 1; 00103 wait_ms(DELAY); 00104 *button = 0; 00105 } 00106 } 00107 00108 void WrittenHandler(const GattWriteCallbackParams *Handler) 00109 { 00110 uint8_t buf[TXRX_BUF_LEN]; 00111 uint16_t bytesRead; 00112 00113 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00114 { 00115 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00116 memset(txPayload, 0, TXRX_BUF_LEN); 00117 memcpy(txPayload, buf, TXRX_BUF_LEN); 00118 00119 switch (buf[OPERATION]) { 00120 case COMMAND: 00121 options = buf[OPTIONS]; 00122 dough_qnt = buf[DOUGH_QNT]; 00123 color = buf[COLOR]; 00124 time_more = buf[TIME_MORE]; 00125 time_less = buf[TIME_LESS]; 00126 init_stop = buf[INIT_STOP]; 00127 break; 00128 case PROGRAM: 00129 ClickButton(&options,&buf[OPTIONS]); 00130 ClickButton(&dough_qnt, &buf[DOUGH_QNT]); 00131 ClickButton(&color, &buf[COLOR]); 00132 ClickButton(&time_more, &buf[TIME_MORE]); 00133 ClickButton(&time_less, &buf[TIME_LESS]); 00134 ClickButton(&init_stop, &buf[INIT_STOP]); 00135 break; 00136 } 00137 } 00138 } 00139 /* 00140 void uartCB(void) 00141 { 00142 while(pc.readable()) 00143 { 00144 rx_buf[rx_len++] = pc.getc(); 00145 if(rx_len>=20 || rx_buf[rx_len-1]=='\0' || rx_buf[rx_len-1]=='\n') 00146 { 00147 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00148 pc.printf("RecHandler \r\n"); 00149 pc.printf("Length: "); 00150 pc.putc(rx_len); 00151 pc.printf("\r\n"); 00152 rx_len = 0; 00153 break; 00154 } 00155 } 00156 } 00157 */ 00158 void m_status_check_handle(void) 00159 { 00160 /* 00161 uint8_t buf[3]; 00162 if (analog_enabled) // if analog reading enabled 00163 { 00164 // Read and send out 00165 float s = ANALOG; 00166 uint16_t value = s*1024; 00167 buf[0] = (0x0B); 00168 buf[1] = (value >> 8); 00169 buf[2] = (value); 00170 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00171 } 00172 00173 If digital in changes, report the state 00174 if (BUTTON != old_state) 00175 { 00176 old_state = BUTTON; 00177 00178 if (BUTTON == 1) 00179 { 00180 buf[0] = (0x0A); 00181 buf[1] = (0x01); 00182 buf[2] = (0x00); 00183 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00184 } 00185 else 00186 { 00187 buf[0] = (0x0A); 00188 buf[1] = (0x00); 00189 buf[2] = (0x00); 00190 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 3); 00191 } 00192 } 00193 */ 00194 } 00195 00196 00197 int main(void) 00198 { 00199 Ticker ticker; 00200 ticker.attach_us(m_status_check_handle, 200000); 00201 00202 ble.init(); 00203 ble.onDisconnection(disconnectionCallback); 00204 ble.onDataWritten(WrittenHandler); 00205 00206 //pc.baud(9600); 00207 //pc.printf("SimpleChat Init \r\n"); 00208 00209 //pc.attach( uartCB , pc.RxIrq); 00210 00211 // setup advertising 00212 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00213 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00214 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00215 (const uint8_t *)"Panificadora", sizeof("Panificadora") - 1); 00216 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00217 (const uint8_t *)uart_base_uuid_rev, sizeof(uart_base_uuid)); 00218 // 100ms; in multiples of 0.625ms. 00219 ble.setAdvertisingInterval(160); 00220 00221 ble.addService(uartService); 00222 00223 ble.startAdvertising(); 00224 00225 //pc.printf("Advertising Start \r\n"); 00226 00227 while(1) 00228 { 00229 ble.waitForEvent(); 00230 } 00231 } 00232 00233 00234 00235 00236 00237 00238 00239 00240 00241 00242 00243 00244 00245 00246 00247 00248 00249 00250 00251 00252 00253 00254 00255 00256 00257 00258 00259 00260 00261 00262
Generated on Wed Jul 13 2022 17:11:43 by
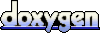