
SimpleBle-Example on mbed-os 5
Dependencies: SimpleBLE mbed nRF51822
Fork of SimpleBLE-Example by
main.cpp
00001 #include "mbed.h" 00002 #include "SimpleBLE.h" 00003 00004 DigitalOut led(LED1); 00005 00006 // The first thing we need to do is create a SimpleBLE instance: 00007 // * first argument is the device name 00008 // * second is the advertisement interval in ms. (default 1000 ms.) 00009 SimpleBLE ble("DEVICE_NAME"); 00010 00011 // Now we can declare some variables that we want to expose. 00012 // After you created the variable you can use it like any other var, 00013 // but it's value will be automatically updated over Bluetooth! 00014 00015 // F.e. here we declare service 0x180d (heartrate), char 0x2a37 (curr. value) as uint8_t 00016 SimpleChar<uint8_t> heartrate = ble.readOnly_u8(0x180D, 0x2A37, true /* notify */, 100 /* default value */); 00017 00018 // now we can use this variable everywhere in our code like a normal uint8_t 00019 void updateHeartrate() { 00020 led = !led; // keep-alive LED 00021 // we just loop between 100 and 180 00022 heartrate = heartrate + 1; 00023 if (heartrate > 180) { 00024 heartrate = 100; 00025 } 00026 } 00027 00028 // And here we create a custom service (0x9310) and char (0x9311) with a callback 00029 void callback(uint32_t newValue) { 00030 // whenever someone updates this var over Bluetooth, this function will be called 00031 printf("My value was updated to %d\n", newValue); 00032 } 00033 // FYI, you can also use long UUID strings here instead of short services :-) 00034 SimpleChar<uint32_t> writeMe = ble.readWrite_u32(0x9310, 0x9311, &callback); 00035 00036 int main(int, char**) { 00037 // update the heart rate every second 00038 Ticker t; 00039 t.attach(updateHeartrate, 1.0f); 00040 00041 // here's how we kick off our loop 00042 ble.start(); 00043 while (1) { 00044 ble.waitForEvent(); 00045 } 00046 00047 }
Generated on Thu Jul 14 2022 18:15:32 by
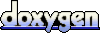