
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
pm.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __PM_H__ 00010 #define __PM_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief PyMite Header 00016 * 00017 * Include things that are needed by nearly everything. 00018 */ 00019 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #ifdef HAVE_SNPRINTF_FORMAT 00026 #include <stdio.h> 00027 #endif 00028 #include <stdint.h> 00029 00030 /** 00031 * Value indicating the release of PyMite 00032 * 00033 * This value should be incremented for every public release. 00034 * It helps locate a defect when used in conjunction with a fileID 00035 * and line number. 00036 */ 00037 #define PM_RELEASE 8 00038 00039 00040 /** null for C code */ 00041 #define C_NULL 0 00042 00043 /** false for C code */ 00044 #define C_FALSE (uint8_t)0 00045 00046 /** true for C code */ 00047 #define C_TRUE (uint8_t)1 00048 00049 /** Comparison result is that items are the same */ 00050 #define C_SAME (int8_t)0 00051 00052 /** Comparison result is that items differ */ 00053 #define C_DIFFER (int8_t)-1 00054 00055 /** PORT inline for C code */ 00056 #define INLINE __inline__ 00057 00058 00059 /** 00060 * Returns an exception error code and stores debug data 00061 * 00062 * This macro must be used as an rval statement. That is, it must 00063 * be used after an assignment such as "retval = " or a return statement 00064 */ 00065 #if __DEBUG__ 00066 #define PM_RAISE(retexn, exn) \ 00067 do \ 00068 { \ 00069 retexn = (exn); \ 00070 gVmGlobal.errFileId = __FILE_ID__; \ 00071 gVmGlobal.errLineNum = (uint16_t)__LINE__; \ 00072 } while (0) 00073 #else 00074 #define PM_RAISE(retexn, exn) \ 00075 retexn = (exn) 00076 #endif 00077 00078 /** if retval is not OK, break from the block */ 00079 #define PM_BREAK_IF_ERROR(retval) if ((retval) != PM_RET_OK) break 00080 00081 /** return an error code if it is not PM_RET_OK */ 00082 #define PM_RETURN_IF_ERROR(retval) if ((retval) != PM_RET_OK) return (retval) 00083 00084 /** print an error message if argument is not PM_RET_OK */ 00085 #define PM_REPORT_IF_ERROR(retval) if ((retval) != PM_RET_OK) \ 00086 plat_reportError(retval) 00087 00088 /** Jumps to a label if argument is not PM_RET_OK */ 00089 #define PM_GOTO_IF_ERROR(retval, target) if ((retval) != PM_RET_OK) \ 00090 goto target 00091 00092 #if __DEBUG__ 00093 /** If the boolean expression fails, return the ASSERT error code */ 00094 #define C_ASSERT(boolexpr) \ 00095 do \ 00096 { \ 00097 if (!((boolexpr))) \ 00098 { \ 00099 gVmGlobal.errFileId = __FILE_ID__; \ 00100 gVmGlobal.errLineNum = (uint16_t)__LINE__; \ 00101 return PM_RET_ASSERT_FAIL; \ 00102 } \ 00103 } \ 00104 while (0) 00105 00106 #else 00107 /** Assert statements are removed from production code */ 00108 #define C_ASSERT(boolexpr) 00109 #endif 00110 00111 /** Use as the first argument to C_DEBUG_PRINT for low volume messages */ 00112 #define VERBOSITY_LOW 1 00113 00114 /** Use as the first argument to C_DEBUG_PRINT for medium volume messages */ 00115 #define VERBOSITY_MEDIUM 2 00116 00117 /** Use as the first argument to C_DEBUG_PRINT for high volume messages */ 00118 #define VERBOSITY_HIGH 3 00119 00120 #if __DEBUG__ 00121 #include <stdio.h> 00122 00123 /** To be used to set DEBUG_PRINT_VERBOSITY to a value so no prints occur */ 00124 #define VERBOSITY_OFF 0 00125 00126 /** Sets the level of verbosity to allow in debug prints */ 00127 #define DEBUG_PRINT_VERBOSITY VERBOSITY_OFF 00128 00129 /** Prints a debug message when the verbosity is within the set value */ 00130 #define C_DEBUG_PRINT(v, f, ...) \ 00131 do \ 00132 { \ 00133 if (DEBUG_PRINT_VERBOSITY >= (v)) \ 00134 { \ 00135 printf("PM_DEBUG: " f, ## __VA_ARGS__); \ 00136 } \ 00137 } \ 00138 while (0) 00139 00140 #else 00141 #define C_DEBUG_PRINT(...) 00142 #endif 00143 00144 00145 /** 00146 * Return values for system functions 00147 * to report status, errors, exceptions, etc. 00148 * Normally, functions which use these values 00149 * should propagate the same return value 00150 * up the call tree to the interpreter. 00151 */ 00152 typedef enum PmReturn_e 00153 { 00154 /* general status return values */ 00155 PM_RET_OK = 0, /**< Everything is ok */ 00156 PM_RET_NO = 0xFF, /**< General "no result" */ 00157 PM_RET_ERR = 0xFE, /**< General failure */ 00158 PM_RET_STUB = 0xFD, /**< Return val for stub fxn */ 00159 PM_RET_ASSERT_FAIL = 0xFC, /**< Assertion failure */ 00160 PM_RET_FRAME_SWITCH = 0xFB, /**< Frame pointer was modified */ 00161 PM_RET_ALIGNMENT = 0xFA, /**< Heap is not aligned */ 00162 00163 /* return vals that indicate an exception occured */ 00164 PM_RET_EX = 0xE0, /**< General exception */ 00165 PM_RET_EX_EXIT = 0xE1, /**< System exit */ 00166 PM_RET_EX_IO = 0xE2, /**< Input/output error */ 00167 PM_RET_EX_ZDIV = 0xE3, /**< Zero division error */ 00168 PM_RET_EX_ASSRT = 0xE4, /**< Assertion error */ 00169 PM_RET_EX_ATTR = 0xE5, /**< Attribute error */ 00170 PM_RET_EX_IMPRT = 0xE6, /**< Import error */ 00171 PM_RET_EX_INDX = 0xE7, /**< Index error */ 00172 PM_RET_EX_KEY = 0xE8, /**< Key error */ 00173 PM_RET_EX_MEM = 0xE9, /**< Memory error */ 00174 PM_RET_EX_NAME = 0xEA, /**< Name error */ 00175 PM_RET_EX_SYNTAX = 0xEB, /**< Syntax error */ 00176 PM_RET_EX_SYS = 0xEC, /**< System error */ 00177 PM_RET_EX_TYPE = 0xED, /**< Type error */ 00178 PM_RET_EX_VAL = 0xEE, /**< Value error */ 00179 PM_RET_EX_STOP = 0xEF, /**< Stop iteration */ 00180 PM_RET_EX_WARN = 0xF0, /**< Warning */ 00181 PM_RET_EX_OFLOW = 0xF1, /**< Overflow */ 00182 } PmReturn_t; 00183 00184 00185 extern volatile uint32_t pm_timerMsTicks; 00186 00187 00188 /* WARNING: The order of the following includes is critical */ 00189 #include "plat.h" 00190 #include "pmfeatures.h" 00191 #include "pmEmptyPlatformDefs.h" 00192 #include "sli.h" 00193 #include "mem.h" 00194 #include "obj.h" 00195 #include "seq.h" 00196 #include "tuple.h" 00197 #include "strobj.h" 00198 #include "heap.h" 00199 #include "int.h" 00200 #include "seglist.h" 00201 #include "list.h" 00202 #include "dict.h" 00203 #include "codeobj.h" 00204 #include "func.h" 00205 #include "module.h" 00206 #include "frame.h" 00207 #include "class.h" 00208 #include "interp.h" 00209 #include "img.h" 00210 #include "global.h" 00211 #include "thread.h" 00212 #include "float.h" 00213 #include "plat_interface.h" 00214 #include "bytearray.h" 00215 00216 00217 /** Pointer to a native function used for lookup tables in interp.c */ 00218 typedef PmReturn_t (* pPmNativeFxn_t)(pPmFrame_t *); 00219 extern pPmNativeFxn_t const std_nat_fxn_table[]; 00220 extern pPmNativeFxn_t const usr_nat_fxn_table[]; 00221 00222 00223 /** 00224 * Initializes the PyMite virtual machine and indexes the user's application 00225 * image. The VM heap and globals are reset. The argument, pusrimg, may be 00226 * null for interactive sessions. 00227 * 00228 * @param heap_base The address where the contiguous heap begins 00229 * @param heap_size The size in bytes (octets) of the given heap. 00230 * Must be a multiple of four. 00231 * @param memspace Memory space in which the user image is located 00232 * @param pusrimg Address of the user image in the memory space 00233 * @return Return status 00234 */ 00235 PmReturn_t pm_init(uint8_t *heap_base, uint32_t heap_size, 00236 PmMemSpace_t memspace, uint8_t const * const pusrimg); 00237 00238 /** 00239 * Executes the named module 00240 * 00241 * @param modstr Name of module to run 00242 * @return Return status 00243 */ 00244 PmReturn_t pm_run(uint8_t const *modstr); 00245 00246 /** 00247 * Needs to be called periodically by the host program. 00248 * For the desktop target, it is periodically called using a signal. 00249 * For embedded targets, it needs to be called periodically. It should 00250 * be called from a timer interrupt. 00251 * 00252 * @param usecsSinceLastCall Microseconds (not less than those) that passed 00253 * since last call. This must be <64535. 00254 * @return Return status 00255 */ 00256 PmReturn_t pm_vmPeriodic(uint16_t usecsSinceLastCall); 00257 00258 #ifdef __cplusplus 00259 } 00260 #endif 00261 00262 #endif /* __PM_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
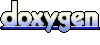