
Mamecontroller/joystick device wrapper library
Embed:
(wiki syntax)
Show/hide line numbers
DebounceIn.h
00001 #ifndef DEBOUNCEIN_H 00002 #define DEBOUNCEIN_H 00003 #include "mbed.h" 00004 00005 class DebounceIn : public DigitalIn { 00006 public: 00007 00008 /** set_debounce_us 00009 * 00010 * Sets the debounce sample period time in microseconds, default is 1000 (1ms) 00011 * 00012 * @param int i The debounce sample period time to set. 00013 */ 00014 void set_debounce_us(int i) { _ticker.attach_us(this, &DebounceIn::_callback, i); } 00015 00016 /** set_samples 00017 * 00018 * Defines the number of samples before switching the shadow 00019 * definition of the pin. 00020 * 00021 * @param int i The number of samples. 00022 */ 00023 void set_samples(int i) { _samples = i; } 00024 00025 /** read 00026 * 00027 * Read the value of the debounced pin. 00028 */ 00029 int read(void) { return _shadow; } 00030 00031 #ifdef MBED_OPERATORS 00032 /** operator int() 00033 * 00034 * Read the value of the debounced pin. 00035 */ 00036 operator int() { return read(); } 00037 #endif 00038 00039 /** Constructor 00040 * 00041 * @param PinName pin The pin to assign as an input. 00042 */ 00043 DebounceIn(PinName pin) : DigitalIn(pin) { _counter = 0; _samples = 10; set_debounce_us(1000); }; 00044 00045 protected: 00046 void _callback(void) { 00047 if (DigitalIn::read()) { 00048 if (_counter < _samples) _counter++; 00049 if (_counter == _samples) _shadow = 1; 00050 } 00051 else { 00052 if (_counter > 0) _counter--; 00053 if (_counter == 0) _shadow = 0; 00054 } 00055 } 00056 00057 Ticker _ticker; 00058 int _shadow; 00059 int _counter; 00060 int _samples; 00061 }; 00062 #endif
Generated on Wed Jul 13 2022 17:27:58 by
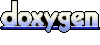