forked from weirdhome
Fork of HTTPClient by
HTTPJson Class Reference
A data endpoint to store JSON text. More...
#include <HTTPJson.h>
Inherits HTTPText.
Public Member Functions | |
HTTPJson (char *json_str) | |
Create an HTTPJson instance for output. | |
HTTPJson (char *json_str, size_t size) | |
Create an HTTPText instance for input. | |
Protected Member Functions | |
virtual int | getDataType (char *type, size_t maxTypeLen) |
Get MIME type. | |
virtual void | readReset () |
Reset stream to its beginning Called by the HTTPClient on each new request. | |
virtual int | read (char *buf, size_t len, size_t *pReadLen) |
Read a piece of data to be transmitted. | |
virtual bool | getIsChunked () |
Determine whether the HTTP client should chunk the data Used for Transfer-Encoding header. | |
virtual size_t | getDataLen () |
If the data is not chunked, get its size Used for Content-Length header. | |
virtual void | writeReset () |
Reset stream to its beginning Called by the HTTPClient on each new request. | |
virtual int | write (const char *buf, size_t len) |
Write a piece of data transmitted by the server. | |
virtual void | setDataType (const char *type) |
Set MIME type. | |
virtual void | setIsChunked (bool chunked) |
Determine whether the data is chunked Recovered from Transfer-Encoding header. | |
virtual void | setDataLen (size_t len) |
If the data is not chunked, set its size From Content-Length header. | |
Friends | |
class | HTTPClient |
class | HTTPClient |
Detailed Description
A data endpoint to store JSON text.
Definition at line 28 of file HTTPJson.h.
Constructor & Destructor Documentation
HTTPJson | ( | char * | json_str ) |
Create an HTTPJson instance for output.
- Parameters:
-
json_str JSON string to be transmitted
Definition at line 26 of file HTTPJson.cpp.
HTTPJson | ( | char * | json_str, |
size_t | size | ||
) |
Create an HTTPText instance for input.
- Parameters:
-
json_str Buffer to store the incoming JSON string size Size of the buffer
Definition at line 31 of file HTTPJson.cpp.
Member Function Documentation
size_t getDataLen | ( | ) | [protected, virtual, inherited] |
If the data is not chunked, get its size Used for Content-Length header.
Implements IHTTPDataOut.
Definition at line 68 of file HTTPText.cpp.
int getDataType | ( | char * | type, |
size_t | maxTypeLen | ||
) | [protected, virtual] |
Get MIME type.
- Parameters:
-
[out] type Internet media type from Content-Type header [in] maxTypeLen is the size of the type buffer to write to
Reimplemented from HTTPText.
Definition at line 36 of file HTTPJson.cpp.
bool getIsChunked | ( | ) | [protected, virtual, inherited] |
Determine whether the HTTP client should chunk the data Used for Transfer-Encoding header.
Implements IHTTPDataOut.
Definition at line 63 of file HTTPText.cpp.
int read | ( | char * | buf, |
size_t | len, | ||
size_t * | pReadLen | ||
) | [protected, virtual, inherited] |
Read a piece of data to be transmitted.
- Parameters:
-
[out] buf Pointer to the buffer on which to copy the data [in] len Length of the buffer [out] pReadLen Pointer to the variable on which the actual copied data length will be stored
Implements IHTTPDataOut.
Definition at line 48 of file HTTPText.cpp.
void readReset | ( | ) | [protected, virtual, inherited] |
Reset stream to its beginning Called by the HTTPClient on each new request.
Implements IHTTPDataOut.
Definition at line 43 of file HTTPText.cpp.
void setDataLen | ( | size_t | len ) | [protected, virtual, inherited] |
If the data is not chunked, set its size From Content-Length header.
Implements IHTTPDataIn.
Definition at line 98 of file HTTPText.cpp.
void setDataType | ( | const char * | type ) | [protected, virtual, inherited] |
Set MIME type.
- Parameters:
-
type Internet media type from Content-Type header
Implements IHTTPDataIn.
Definition at line 88 of file HTTPText.cpp.
void setIsChunked | ( | bool | chunked ) | [protected, virtual, inherited] |
Determine whether the data is chunked Recovered from Transfer-Encoding header.
Implements IHTTPDataIn.
Definition at line 93 of file HTTPText.cpp.
int write | ( | const char * | buf, |
size_t | len | ||
) | [protected, virtual, inherited] |
Write a piece of data transmitted by the server.
- Parameters:
-
buf Pointer to the buffer from which to copy the data len Length of the buffer
Implements IHTTPDataIn.
Definition at line 79 of file HTTPText.cpp.
void writeReset | ( | ) | [protected, virtual, inherited] |
Reset stream to its beginning Called by the HTTPClient on each new request.
Implements IHTTPDataIn.
Definition at line 74 of file HTTPText.cpp.
Generated on Thu Jul 14 2022 02:08:43 by
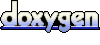