This is the open source Pawn interpreter ported to mbed. See here: http://www.compuphase.com/pawn/pawn.htm and here: http://code.google.com/p/pawnscript/
amxmbed.cpp
00001 /** 00002 * Interface between Pawn interpreter and mbed platform. 00003 * 00004 * Copyright 2011 Pulse-Robotics, Inc. 00005 * Author: Tyler Wilson 00006 */ 00007 00008 #include <assert.h> 00009 00010 #include "_amxmbed.h" 00011 #include "amxmbed.h" 00012 #include "amx.h" 00013 #include "mbed.h" 00014 00015 Serial* port = 0; 00016 00017 void mbed_set_serial(Serial* serial) 00018 { 00019 port = serial; 00020 } 00021 00022 int amx_putstr(const char *s) 00023 { 00024 return port?port->printf(s):0; 00025 } 00026 00027 int amx_putchar(int c) 00028 { 00029 return port?port->putc(c):0; 00030 } 00031 00032 int amx_fflush(void) 00033 { 00034 return 0; 00035 } 00036 00037 int amx_getch(void) 00038 { 00039 return port?port->getc():0; 00040 } 00041 00042 char *amx_gets(char* s, int i) 00043 { 00044 return port?port->gets(s,i):0; 00045 } 00046 00047 int amx_termctl(int,int) 00048 { 00049 return 0; 00050 } 00051 00052 void amx_clrscr(void) 00053 { 00054 } 00055 00056 void amx_clreol(void) 00057 { 00058 } 00059 00060 int amx_gotoxy(int x,int y) 00061 { 00062 return 0; 00063 } 00064 00065 void amx_wherexy(int *x,int *y) 00066 { 00067 } 00068 00069 unsigned int amx_setattr(int foregr,int backgr,int highlight) 00070 { 00071 return 0; 00072 } 00073 00074 void amx_console(int columns, int lines, int flags) 00075 { 00076 } 00077 00078 void amx_viewsize(int *width,int *height) 00079 { 00080 } 00081 00082 int amx_kbhit(void) 00083 { 00084 return port?port->readable():0; 00085 } 00086 00087 static cell AMX_NATIVE_CALL n_digitalOpen(AMX *amx, const cell *params) 00088 { 00089 (void)amx; 00090 DigitalOut* self = new DigitalOut((PinName)params[1]); 00091 // port->printf("digitalOpen(0x%x) returns 0x%x\n\r", (PinName)params[1], self); 00092 return (cell)self; 00093 } 00094 00095 static cell AMX_NATIVE_CALL n_digitalRead(AMX *amx, const cell *params) 00096 { 00097 // port->printf("digitalRead\n\r"); 00098 (void)amx; 00099 DigitalOut* obj = (DigitalOut*)params[1]; 00100 if (obj) 00101 { 00102 return obj->read() != 0; 00103 } 00104 00105 return 0; 00106 } 00107 00108 static cell AMX_NATIVE_CALL n_digitalWrite(AMX *amx, const cell *params) 00109 { 00110 DigitalOut* obj = (DigitalOut*)params[1]; 00111 // port->printf("digitalWrite(0x%x, %d)\n\r", obj, params[2]); 00112 if (obj) 00113 { 00114 obj->write(params[2]); 00115 } 00116 00117 return 0; 00118 } 00119 00120 static cell AMX_NATIVE_CALL n_digitalClose(AMX *amx, const cell *params) 00121 { 00122 // port->printf("digitalClose\n\r"); 00123 //(void)amx; 00124 DigitalOut* obj = (DigitalOut*)params[1]; 00125 if (obj) 00126 { 00127 delete obj; 00128 } 00129 00130 return 0; 00131 } 00132 00133 static cell AMX_NATIVE_CALL n_analogInOpen(AMX * amx, const cell *params) 00134 { 00135 (void)amx; 00136 AnalogIn* self = new AnalogIn((PinName)params[1]); 00137 00138 return (cell)self; 00139 } 00140 00141 static cell AMX_NATIVE_CALL n_analogInClose(AMX *amx, const cell params[]) 00142 { 00143 (void)amx; 00144 AnalogIn* obj = (AnalogIn*)params[1]; 00145 00146 if(obj) 00147 { 00148 return(obj->read()); 00149 } 00150 00151 return 0; 00152 } 00153 00154 static cell AMX_NATIVE_CALL n_analogInRead(AMX *amx, const cell params[]) 00155 { 00156 (void)amx; 00157 AnalogIn* obj = (AnalogIn*)params[1]; 00158 00159 if(obj) 00160 { 00161 float voltage = obj->read(); 00162 00163 port->printf("%f read from analog in pin \n\r",voltage); 00164 return(amx_ftoc(voltage)); 00165 } 00166 00167 return 0; 00168 } 00169 00170 static cell AMX_NATIVE_CALL n_analogOutOpen(AMX *amx, const cell params[]) 00171 { 00172 (void)amx; 00173 AnalogOut* self = new AnalogOut((PinName)params[1]); 00174 00175 return (cell)self; 00176 } 00177 00178 static cell AMX_NATIVE_CALL n_analogOutRead(AMX *amx, const cell params[]) 00179 { 00180 (void)amx; 00181 AnalogOut* obj = (AnalogOut*)params[1]; 00182 00183 if(obj) 00184 { 00185 float voltage = obj->read(); 00186 port->printf("reading voltage of %f in analog out pin\n\r",voltage); 00187 00188 return(amx_ftoc(voltage)); 00189 } 00190 00191 return 0; 00192 } 00193 00194 static cell AMX_NATIVE_CALL n_analogOutWrite(AMX *amx, const cell params[]) 00195 { 00196 (void)amx; 00197 AnalogOut* obj = (AnalogOut*)params[1]; 00198 00199 if(obj) 00200 { 00201 float voltage = amx_ctof(params[2]); 00202 port->printf("writing voltage of %f to analog out pin\n\r",voltage); 00203 obj->write(voltage); 00204 } 00205 00206 return(0); 00207 } 00208 00209 static cell AMX_NATIVE_CALL n_analogOutClose(AMX* amx, const cell params[]) 00210 { 00211 (void)amx; 00212 AnalogOut* obj = (AnalogOut*)params[1]; 00213 00214 if(obj) 00215 { 00216 delete obj; 00217 } 00218 return 0; 00219 } 00220 00221 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00222 static cell AMX_NATIVE_CALL n_wait(AMX *amx, const cell *params) 00223 { 00224 float amount = amx_ctof(params[1]); 00225 00226 wait(amount); 00227 00228 return 0; 00229 } 00230 #endif 00231 00232 static cell AMX_NATIVE_CALL n_wait_ms(AMX *amx, const cell *params) 00233 { 00234 int amount = (int)params[1]; 00235 // port->printf("waiting %d ms\n\r", amount); 00236 wait_ms(amount); 00237 00238 return 0; 00239 } 00240 00241 static cell AMX_NATIVE_CALL n_wait_us(AMX *amx, const cell *params) 00242 { 00243 int amount = (int)params[1]; 00244 wait_us(amount); 00245 return 0; 00246 } 00247 00248 static cell AMX_NATIVE_CALL n_kbhit(AMX *amx, const cell *params) 00249 { 00250 return amx_kbhit() != 0; 00251 } 00252 00253 00254 const AMX_NATIVE_INFO mbed_Natives[] = { 00255 { "digitalOpen", n_digitalOpen }, 00256 { "digitalRead", n_digitalRead }, 00257 { "digitalWrite", n_digitalWrite }, 00258 { "digitalClose", n_digitalClose }, 00259 { "analogInOpen", n_analogInOpen }, 00260 { "analogInClose", n_analogInClose }, 00261 { "analogInRead", n_analogInRead }, 00262 { "analogOutOpen", n_analogOutOpen }, 00263 { "analogOutRead", n_analogOutRead }, 00264 { "analogOutWrite", n_analogOutWrite }, 00265 { "analogOutClose", n_analogOutClose }, 00266 00267 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00268 { "wait", n_wait }, // uses a float, which we do not support on LPC11U24 version 00269 #endif 00270 { "wait_ms", n_wait_ms }, 00271 { "wait_us", n_wait_us }, 00272 00273 { "kbhit", n_kbhit }, 00274 { NULL, NULL } /* terminator */ 00275 }; 00276 00277 int AMXEXPORT AMXAPI amx_mbedInit(AMX *amx) 00278 { 00279 return amx_Register(amx, mbed_Natives, -1); 00280 } 00281 00282 int AMXEXPORT AMXAPI amx_mbedCleanup(AMX *amx) 00283 { 00284 (void)amx; 00285 return AMX_ERR_NONE; 00286 }
Generated on Sat Jul 16 2022 16:09:35 by
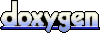