This is the open source Pawn interpreter ported to mbed. See here: http://www.compuphase.com/pawn/pawn.htm and here: http://code.google.com/p/pawnscript/
amxaux.c
00001 /* Support routines for the Pawn Abstract Machine 00002 * 00003 * Copyright (c) ITB CompuPhase, 2003-2011 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 * 00017 * Version: $Id: amxaux.c 4523 2011-06-21 15:03:47Z thiadmer $ 00018 */ 00019 #include <stdio.h> 00020 #include <stdlib.h> 00021 #include <string.h> 00022 #include "amx.h" 00023 #include "amxaux.h" 00024 00025 size_t AMXAPI aux_ProgramSize(const char *filename) 00026 { 00027 FILE *fp; 00028 AMX_HEADER hdr; 00029 00030 if ((fp=fopen(filename,"rb")) == NULL) 00031 return 0; 00032 fread(&hdr, sizeof hdr, 1, fp); 00033 fclose(fp); 00034 00035 amx_Align16(&hdr.magic); 00036 amx_Align32((uint32_t *)&hdr.stp); 00037 return (hdr.magic==AMX_MAGIC) ? (size_t)hdr.stp : 0; 00038 } 00039 00040 int AMXAPI aux_LoadProgram(AMX *amx, const char *filename, void *memblock) 00041 { 00042 FILE *fp; 00043 AMX_HEADER hdr; 00044 int result, didalloc; 00045 00046 /* open the file, read and check the header */ 00047 if ((fp = fopen(filename, "rb")) == NULL) 00048 return AMX_ERR_NOTFOUND; 00049 fread(&hdr, sizeof hdr, 1, fp); 00050 amx_Align16(&hdr.magic); 00051 amx_Align32((uint32_t *)&hdr.size); 00052 amx_Align32((uint32_t *)&hdr.stp); 00053 if (hdr.magic != AMX_MAGIC) { 00054 fclose(fp); 00055 return AMX_ERR_FORMAT; 00056 } /* if */ 00057 00058 /* allocate the memblock if it is NULL */ 00059 didalloc = 0; 00060 if (memblock == NULL) { 00061 if ((memblock = malloc(hdr.stp)) == NULL) { 00062 fclose(fp); 00063 return AMX_ERR_MEMORY; 00064 } /* if */ 00065 didalloc = 1; 00066 /* after amx_Init(), amx->base points to the memory block */ 00067 } /* if */ 00068 00069 /* read in the file */ 00070 rewind(fp); 00071 fread(memblock, 1, (size_t)hdr.size, fp); 00072 fclose(fp); 00073 00074 /* initialize the abstract machine */ 00075 memset(amx, 0, sizeof *amx); 00076 result = amx_Init(amx, memblock); 00077 00078 /* free the memory block on error, if it was allocated here */ 00079 if (result != AMX_ERR_NONE && didalloc) { 00080 free(memblock); 00081 amx->base = NULL; /* avoid a double free */ 00082 } /* if */ 00083 00084 return result; 00085 } 00086 00087 int AMXAPI aux_FreeProgram(AMX *amx) 00088 { 00089 if (amx->base!=NULL) { 00090 amx_Cleanup(amx); 00091 free(amx->base); 00092 memset(amx, 0, sizeof(AMX)); 00093 } /* if */ 00094 return AMX_ERR_NONE; 00095 } 00096 00097 char * AMXAPI aux_StrError(int errnum) 00098 { 00099 static char *messages[] = { 00100 /* AMX_ERR_NONE */ "(none)", 00101 /* AMX_ERR_EXIT */ "Forced exit", 00102 /* AMX_ERR_ASSERT */ "Assertion failed", 00103 /* AMX_ERR_STACKERR */ "Stack/heap collision (insufficient stack size)", 00104 /* AMX_ERR_BOUNDS */ "Array index out of bounds", 00105 /* AMX_ERR_MEMACCESS */ "Invalid memory access", 00106 /* AMX_ERR_INVINSTR */ "Invalid instruction", 00107 /* AMX_ERR_STACKLOW */ "Stack underflow", 00108 /* AMX_ERR_HEAPLOW */ "Heap underflow", 00109 /* AMX_ERR_CALLBACK */ "No (valid) native function callback", 00110 /* AMX_ERR_NATIVE */ "Native function failed", 00111 /* AMX_ERR_DIVIDE */ "Divide by zero", 00112 /* AMX_ERR_SLEEP */ "(sleep mode)", 00113 /* AMX_ERR_INVSTATE */ "Invalid state", 00114 /* 14 */ "(reserved)", 00115 /* 15 */ "(reserved)", 00116 /* AMX_ERR_MEMORY */ "Out of memory", 00117 /* AMX_ERR_FORMAT */ "Invalid/unsupported P-code file format", 00118 /* AMX_ERR_VERSION */ "File is for a newer version of the AMX", 00119 /* AMX_ERR_NOTFOUND */ "File or function is not found", 00120 /* AMX_ERR_INDEX */ "Invalid index parameter (bad entry point)", 00121 /* AMX_ERR_DEBUG */ "Debugger cannot run", 00122 /* AMX_ERR_INIT */ "AMX not initialized (or doubly initialized)", 00123 /* AMX_ERR_USERDATA */ "Unable to set user data field (table full)", 00124 /* AMX_ERR_INIT_JIT */ "Cannot initialize the JIT", 00125 /* AMX_ERR_PARAMS */ "Parameter error", 00126 /* AMX_ERR_DOMAIN */ "Domain error, expression result does not fit in range", 00127 /* AMX_ERR_GENERAL */ "General error (unknown or unspecific error)", 00128 /* AMX_ERR_OVERLAY */ "Overlays are unsupported (JIT) or uninitialized", 00129 }; 00130 if (errnum < 0 || errnum >= sizeof messages / sizeof messages[0]) 00131 return "(unknown)"; 00132 return messages[errnum]; 00133 } 00134 00135 int AMXAPI aux_GetSection(const AMX *amx, int section, cell **start, size_t *size) 00136 { 00137 AMX_HEADER *hdr; 00138 00139 if (amx == NULL || start == NULL || size == NULL) 00140 return AMX_ERR_PARAMS; 00141 00142 hdr = (AMX_HEADER*)amx->base; 00143 switch(section) { 00144 case CODE_SECTION: 00145 *start = (cell *)(amx->base + hdr->cod); 00146 *size = hdr->dat - hdr->cod; 00147 break; 00148 case DATA_SECTION: 00149 *start = (cell *)(amx->data); 00150 *size = hdr->hea - hdr->dat; 00151 break; 00152 case HEAP_SECTION: 00153 *start = (cell *)(amx->data + hdr->hea); 00154 *size = amx->hea - hdr->hea; 00155 break; 00156 case STACK_SECTION: 00157 *start = (cell *)(amx->data + amx->stk); 00158 *size = amx->stp - amx->stk; 00159 break; 00160 default: 00161 return AMX_ERR_PARAMS; 00162 } /* switch */ 00163 return AMX_ERR_NONE; 00164 }
Generated on Sat Jul 16 2022 16:09:35 by
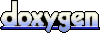