This is the open source Pawn interpreter ported to mbed. See here: http://www.compuphase.com/pawn/pawn.htm and here: http://code.google.com/p/pawnscript/
amx.h
00001 /* Pawn Abstract Machine (for the Pawn language) 00002 * 00003 * Copyright (c) ITB CompuPhase, 1997-2012 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 * 00017 * Version: $Id: amx.h 4731 2012-06-21 11:11:18Z thiadmer $ 00018 */ 00019 00020 #ifndef AMX_H_INCLUDED 00021 #define AMX_H_INCLUDED 00022 00023 #include <stdlib.h> /* for size_t */ 00024 #include <limits.h> 00025 00026 #if defined __linux || defined __linux__ 00027 #define __LINUX__ 00028 #endif 00029 #if defined FREEBSD && !defined __FreeBSD__ 00030 #define __FreeBSD__ 00031 #endif 00032 #if defined __LINUX__ || defined __FreeBSD__ || defined __OpenBSD__ 00033 #include <sclinux.h> 00034 #endif 00035 00036 #if !defined HAVE_STDINT_H 00037 #if (defined __STDC_VERSION__ && __STDC_VERSION__ >= 199901L) \ 00038 || defined __GNUC__ || defined __LCC__ || defined __DMC__ \ 00039 || (defined __WATCOMC__ && __WATCOMC__ >= 1200) 00040 #define HAVE_STDINT_H 1 00041 #endif 00042 #endif 00043 #if !defined HAVE_INTTYPES_H 00044 #if defined __FreeBSD__ 00045 #define HAVE_INTTYPES_H 1 00046 #endif 00047 #endif 00048 #if defined HAVE_STDINT_H 00049 #include <stdint.h> 00050 #elif defined HAVE_INTTYPES_H 00051 #include <inttypes.h> 00052 #else 00053 #if defined __MACH__ 00054 #include <ppc/types.h> 00055 #endif 00056 typedef short int int16_t; 00057 typedef unsigned short int uint16_t; 00058 #if defined SN_TARGET_PS2 00059 typedef int int32_t; 00060 typedef unsigned int uint32_t; 00061 #else 00062 typedef long int int32_t; 00063 typedef unsigned long int uint32_t; 00064 #endif 00065 #if defined __WIN32__ || defined _WIN32 || defined WIN32 00066 typedef __int64 int64_t; 00067 typedef unsigned __int64 uint64_t; 00068 #define HAVE_I64 00069 #endif 00070 #if !defined _INTPTR_T_DEFINED 00071 #if defined _LP64 || defined WIN64 || defined _WIN64 00072 typedef __int64 intptr_t; 00073 #else 00074 typedef int32_t intptr_t; 00075 #endif 00076 #endif 00077 #endif 00078 #if defined _LP64 || defined WIN64 || defined _WIN64 00079 #if !defined __64BIT__ 00080 #define __64BIT__ 00081 #endif 00082 #endif 00083 00084 #if !defined HAVE_ALLOCA_H 00085 #if defined __GNUC__ || defined __LCC__ || defined __DMC__ || defined __ARMCC_VERSION 00086 #define HAVE_ALLOCA_H 1 00087 #elif defined __WATCOMC__ && __WATCOMC__ >= 1200 00088 #define HAVE_ALLOCA_H 1 00089 #endif 00090 #endif 00091 #if defined HAVE_ALLOCA_H && HAVE_ALLOCA_H 00092 #include <alloca.h> 00093 #elif defined __BORLANDC__ 00094 #include <malloc.h> 00095 #endif 00096 #if defined __WIN32__ || defined _WIN32 || defined WIN32 /* || defined __MSDOS__ */ 00097 #if !defined alloca 00098 #define alloca(n) _alloca(n) 00099 #endif 00100 #endif 00101 00102 #if !defined assert_static 00103 /* see "Compile-Time Assertions" by Greg Miller, 00104 * (with modifications to port it to C) 00105 */ 00106 #define _ASSERT_STATIC_SYMBOL_INNER(line) __ASSERT_STATIC_ ## line 00107 #define _ASSERT_STATIC_SYMBOL(line) _ASSERT_STATIC_SYMBOL_INNER(line) 00108 #define assert_static(test) \ 00109 do { \ 00110 typedef char _ASSERT_STATIC_SYMBOL(__LINE__)[ ((test) ? 1 : -1) ]; \ 00111 } while (0) 00112 #endif 00113 00114 #if defined __cplusplus 00115 extern "C" { 00116 #endif 00117 00118 #if defined PAWN_DLL 00119 #if !defined AMX_NATIVE_CALL 00120 #define AMX_NATIVE_CALL __stdcall 00121 #endif 00122 #if !defined AMXAPI 00123 #define AMXAPI __stdcall 00124 #endif 00125 #if !defined AMXEXPORT 00126 #define AMXEXPORT __declspec(dllexport) 00127 #endif 00128 #endif 00129 00130 /* calling convention for native functions */ 00131 #if !defined AMX_NATIVE_CALL 00132 #define AMX_NATIVE_CALL 00133 #endif 00134 /* calling convention for all interface functions and callback functions */ 00135 #if !defined AMXAPI 00136 #if defined STDECL 00137 #define AMXAPI __stdcall 00138 #elif defined CDECL 00139 #define AMXAPI __cdecl 00140 #elif defined GCC_HASCLASSVISIBILITY 00141 #define AMXAPI __attribute__((visibility("default"))) 00142 #else 00143 #define AMXAPI 00144 #endif 00145 #endif 00146 #if !defined AMXEXPORT 00147 #define AMXEXPORT 00148 #endif 00149 00150 /* File format version (in CUR_FILE_VERSION) 00151 * 0 original version 00152 * 1 opcodes JUMP.pri, SWITCH and CASETBL 00153 * 2 compressed files 00154 * 3 public variables 00155 * 4 opcodes SWAP.pri/alt and PUSHADDR 00156 * 5 tagnames table 00157 * 6 reformatted header 00158 * 7 name table, opcodes SYMTAG & SYSREQ.D 00159 * 8 opcode BREAK, renewed debug interface 00160 * 9 macro opcodes 00161 * 10 position-independent code, overlays, packed instructions 00162 * 11 relocating instructions for the native interface, reorganized instruction set 00163 * MIN_FILE_VERSION is the lowest file version number that the current AMX 00164 * implementation supports. If the AMX file header gets new fields, this number 00165 * often needs to be incremented. MIN_AMX_VERSION is the lowest AMX version that 00166 * is needed to support the current file version. When there are new opcodes, 00167 * this number needs to be incremented. 00168 * The file version supported by the JIT may run behind MIN_AMX_VERSION. So 00169 * there is an extra constant for it: MAX_FILE_VER_JIT. 00170 */ 00171 #define CUR_FILE_VERSION 11 /* current file version; also the current AMX version */ 00172 #define MIN_FILE_VERSION 11 /* lowest supported file format version for the current AMX version */ 00173 #define MIN_AMX_VERSION 11 /* minimum AMX version needed to support the current file format */ 00174 #define MAX_FILE_VER_JIT 11 /* file version supported by the JIT */ 00175 #define MIN_AMX_VER_JIT 11 /* AMX version supported by the JIT */ 00176 00177 #if !defined PAWN_CELL_SIZE 00178 #define PAWN_CELL_SIZE 32 /* by default, use 32-bit cells */ 00179 #endif 00180 #if PAWN_CELL_SIZE==16 00181 typedef uint16_t ucell; 00182 typedef int16_t cell; 00183 #elif PAWN_CELL_SIZE==32 00184 typedef uint32_t ucell; 00185 typedef int32_t cell; 00186 #elif PAWN_CELL_SIZE==64 00187 typedef uint64_t ucell; 00188 typedef int64_t cell; 00189 #else 00190 #error Unsupported cell size (PAWN_CELL_SIZE) 00191 #endif 00192 00193 #define UNPACKEDMAX (((cell)1 << (sizeof(cell)-1)*8) - 1) 00194 #define UNLIMITED (~1u >> 1) 00195 00196 struct tagAMX; 00197 typedef cell (AMX_NATIVE_CALL *AMX_NATIVE)(struct tagAMX *amx, const cell *params); 00198 typedef int (AMXAPI *AMX_CALLBACK)(struct tagAMX *amx, cell index, 00199 cell *result, const cell *params); 00200 typedef int (AMXAPI *AMX_DEBUG)(struct tagAMX *amx); 00201 typedef int (AMXAPI *AMX_OVERLAY)(struct tagAMX *amx, int index); 00202 typedef int (AMXAPI *AMX_IDLE)(struct tagAMX *amx, int AMXAPI Exec(struct tagAMX *, cell *, int)); 00203 #if !defined _FAR 00204 #define _FAR 00205 #endif 00206 00207 #if defined _MSC_VER 00208 #pragma warning(disable:4103) /* disable warning message 4103 that complains 00209 * about pragma pack in a header file */ 00210 #pragma warning(disable:4100) /* "'%$S' : unreferenced formal parameter" */ 00211 #pragma warning(disable:4996) /* POSIX name is deprecated */ 00212 #endif 00213 00214 /* Some compilers do not support the #pragma align, which should be fine. Some 00215 * compilers give a warning on unknown #pragmas, which is not so fine... 00216 */ 00217 #if (defined SN_TARGET_PS2 || defined __GNUC__) && !defined AMX_NO_ALIGN 00218 #define AMX_NO_ALIGN 00219 #endif 00220 00221 #if defined __GNUC__ 00222 #define PACKED __attribute__((packed)) 00223 #else 00224 #define PACKED 00225 #endif 00226 00227 #if !defined AMX_NO_ALIGN 00228 #if defined __LINUX__ || defined __FreeBSD__ 00229 #pragma pack(1) /* structures must be packed (byte-aligned) */ 00230 #elif defined MACOS && defined __MWERKS__ 00231 #pragma options align=mac68k 00232 #else 00233 #pragma pack(push) 00234 #pragma pack(1) /* structures must be packed (byte-aligned) */ 00235 #if defined __TURBOC__ 00236 #pragma option -a- /* "pack" pragma for older Borland compilers */ 00237 #endif 00238 #endif 00239 #endif 00240 00241 typedef struct tagAMX_NATIVE_INFO { 00242 const char _FAR *name; 00243 AMX_NATIVE func; 00244 } PACKED AMX_NATIVE_INFO; 00245 00246 #if !defined AMX_USERNUM 00247 #define AMX_USERNUM 4 00248 #endif 00249 #define sEXPMAX 19 /* maximum name length for file version <= 6 */ 00250 #define sNAMEMAX 31 /* maximum name length of symbol name */ 00251 00252 typedef struct tagFUNCSTUB { 00253 uint32_t address; 00254 uint32_t nameofs; 00255 } PACKED AMX_FUNCSTUB; 00256 00257 typedef struct tagOVERLAYINFO { 00258 int32_t offset; /* offset relative to the start of the code block */ 00259 int32_t size; /* size in bytes */ 00260 } PACKED AMX_OVERLAYINFO; 00261 00262 /* The AMX structure is the internal structure for many functions. Not all 00263 * fields are valid at all times; many fields are cached in local variables. 00264 */ 00265 typedef struct tagAMX { 00266 unsigned char _FAR *base; /* points to the AMX header, perhaps followed by P-code and data */ 00267 unsigned char _FAR *code; /* points to P-code block, possibly in ROM or in an overlay pool */ 00268 unsigned char _FAR *data; /* points to separate data+stack+heap, may be NULL */ 00269 AMX_CALLBACK callback; /* native function callback */ 00270 AMX_DEBUG debug; /* debug callback */ 00271 AMX_OVERLAY overlay; /* overlay reader callback */ 00272 /* for external functions a few registers must be accessible from the outside */ 00273 cell cip; /* instruction pointer: relative to base + amxhdr->cod */ 00274 cell frm; /* stack frame base: relative to base + amxhdr->dat */ 00275 cell hea; /* top of the heap: relative to base + amxhdr->dat */ 00276 cell hlw; /* bottom of the heap: relative to base + amxhdr->dat */ 00277 cell stk; /* stack pointer: relative to base + amxhdr->dat */ 00278 cell stp; /* top of the stack: relative to base + amxhdr->dat */ 00279 int flags; /* current status, see amx_Flags() */ 00280 /* user data */ 00281 #if AMX_USERNUM > 0 00282 long usertags[AMX_USERNUM]; 00283 void _FAR *userdata[AMX_USERNUM]; 00284 #endif 00285 /* native functions can raise an error */ 00286 int error; 00287 /* passing parameters requires a "count" field */ 00288 int paramcount; 00289 /* the sleep opcode needs to store the full AMX status */ 00290 cell pri; 00291 cell alt; 00292 cell reset_stk; 00293 cell reset_hea; 00294 /* extra fields for increased performance */ 00295 cell sysreq_d; /* relocated address/value for the SYSREQ.D opcode */ 00296 /* fields for overlay support and JIT support */ 00297 int ovl_index; /* current overlay index */ 00298 long codesize; /* size of the overlay, or estimated memory footprint of the native code */ 00299 #if defined AMX_JIT 00300 /* support variables for the JIT */ 00301 int reloc_size; /* required temporary buffer for relocations */ 00302 #endif 00303 } PACKED AMX; 00304 00305 /* The AMX_HEADER structure is both the memory format as the file format. The 00306 * structure is used internaly. 00307 */ 00308 typedef struct tagAMX_HEADER { 00309 int32_t size; /* size of the "file" */ 00310 uint16_t magic; /* signature */ 00311 char file_version; /* file format version */ 00312 char amx_version; /* required version of the AMX */ 00313 int16_t flags; 00314 int16_t defsize; /* size of a definition record */ 00315 int32_t cod; /* initial value of COD - code block */ 00316 int32_t dat; /* initial value of DAT - data block */ 00317 int32_t hea; /* initial value of HEA - start of the heap */ 00318 int32_t stp; /* initial value of STP - stack top */ 00319 int32_t cip; /* initial value of CIP - the instruction pointer */ 00320 int32_t publics; /* offset to the "public functions" table */ 00321 int32_t natives; /* offset to the "native functions" table */ 00322 int32_t libraries; /* offset to the table of libraries */ 00323 int32_t pubvars; /* offset to the "public variables" table */ 00324 int32_t tags; /* offset to the "public tagnames" table */ 00325 int32_t nametable; /* offset to the name table */ 00326 int32_t overlays; /* offset to the overlay table */ 00327 } PACKED AMX_HEADER; 00328 00329 #define AMX_MAGIC_16 0xf1e2 00330 #define AMX_MAGIC_32 0xf1e0 00331 #define AMX_MAGIC_64 0xf1e1 00332 #if PAWN_CELL_SIZE==16 00333 #define AMX_MAGIC AMX_MAGIC_16 00334 #elif PAWN_CELL_SIZE==32 00335 #define AMX_MAGIC AMX_MAGIC_32 00336 #elif PAWN_CELL_SIZE==64 00337 #define AMX_MAGIC AMX_MAGIC_64 00338 #endif 00339 00340 enum { 00341 AMX_ERR_NONE, 00342 /* reserve the first 15 error codes for exit codes of the abstract machine */ 00343 AMX_ERR_EXIT, /* forced exit */ 00344 AMX_ERR_ASSERT, /* assertion failed */ 00345 AMX_ERR_STACKERR, /* stack/heap collision */ 00346 AMX_ERR_BOUNDS, /* index out of bounds */ 00347 AMX_ERR_MEMACCESS, /* invalid memory access */ 00348 AMX_ERR_INVINSTR, /* invalid instruction */ 00349 AMX_ERR_STACKLOW, /* stack underflow */ 00350 AMX_ERR_HEAPLOW, /* heap underflow */ 00351 AMX_ERR_CALLBACK, /* no callback, or invalid callback */ 00352 AMX_ERR_NATIVE, /* native function failed */ 00353 AMX_ERR_DIVIDE, /* divide by zero */ 00354 AMX_ERR_SLEEP, /* go into sleepmode - code can be restarted */ 00355 AMX_ERR_INVSTATE, /* no implementation for this state, no fall-back */ 00356 00357 AMX_ERR_MEMORY = 16, /* out of memory */ 00358 AMX_ERR_FORMAT, /* invalid file format */ 00359 AMX_ERR_VERSION, /* file is for a newer version of the AMX */ 00360 AMX_ERR_NOTFOUND, /* function not found */ 00361 AMX_ERR_INDEX, /* invalid index parameter (bad entry point) */ 00362 AMX_ERR_DEBUG, /* debugger cannot run */ 00363 AMX_ERR_INIT, /* AMX not initialized (or doubly initialized) */ 00364 AMX_ERR_USERDATA, /* unable to set user data field (table full) */ 00365 AMX_ERR_INIT_JIT, /* cannot initialize the JIT */ 00366 AMX_ERR_PARAMS, /* parameter error */ 00367 AMX_ERR_DOMAIN, /* domain error, expression result does not fit in range */ 00368 AMX_ERR_GENERAL, /* general error (unknown or unspecific error) */ 00369 AMX_ERR_OVERLAY, /* overlays are unsupported (JIT) or uninitialized */ 00370 }; 00371 00372 #define AMX_FLAG_OVERLAY 0x01 /* all function calls use overlays */ 00373 #define AMX_FLAG_DEBUG 0x02 /* symbolic info. available */ 00374 #define AMX_FLAG_NOCHECKS 0x04 /* no array bounds checking; no BREAK opcodes */ 00375 #define AMX_FLAG_SLEEP 0x08 /* script uses the sleep instruction (possible re-entry or power-down mode) */ 00376 #define AMX_FLAG_CRYPT 0x10 /* file is encrypted */ 00377 #define AMX_FLAG_DSEG_INIT 0x20 /* data section is explicitly initialized */ 00378 #define AMX_FLAG_SYSREQN 0x800 /* script uses new (optimized) version of SYSREQ opcode */ 00379 #define AMX_FLAG_NTVREG 0x1000 /* all native functions are registered */ 00380 #define AMX_FLAG_JITC 0x2000 /* abstract machine is JIT compiled */ 00381 #define AMX_FLAG_VERIFY 0x4000 /* busy verifying P-code */ 00382 #define AMX_FLAG_INIT 0x8000 /* AMX has been initialized */ 00383 00384 #define AMX_EXEC_MAIN (-1) /* start at program entry point */ 00385 #define AMX_EXEC_CONT (-2) /* continue from last address */ 00386 00387 #define AMX_USERTAG(a,b,c,d) ((a) | ((b)<<8) | ((long)(c)<<16) | ((long)(d)<<24)) 00388 00389 /* for native functions that use floating point parameters, the following 00390 * two macros are convenient for casting a "cell" into a "float" type _without_ 00391 * changing the bit pattern 00392 */ 00393 #if PAWN_CELL_SIZE==32 00394 #define amx_ftoc(f) ( * ((cell*)&f) ) /* float to cell */ 00395 #define amx_ctof(c) ( * ((float*)&c) ) /* cell to float */ 00396 #elif PAWN_CELL_SIZE==64 00397 #define amx_ftoc(f) ( * ((cell*)&f) ) /* float to cell */ 00398 #define amx_ctof(c) ( * ((double*)&c) ) /* cell to float */ 00399 #else 00400 // amx_ftoc() and amx_ctof() cannot be used 00401 #endif 00402 00403 /* when a pointer cannot be stored in a cell, cells that hold relocated 00404 * addresses need to be expanded 00405 */ 00406 #if defined __64BIT__ && PAWN_CELL_SIZE<64 00407 #define CELLMASK (((int64_t)1 << PAWN_CELL_SIZE) - 1) 00408 #define amx_Address(amx,addr) \ 00409 (cell*)(((int64_t)((amx)->data ? (amx)->data : (amx)->code) & ~CELLMASK) | ((int64_t)(addr) & CELLMASK)) 00410 #elif defined __32BIT__ && PAWN_CELL_SIZE<32 00411 #define CELLMASK ((1L << PAWN_CELL_SIZE) - 1) 00412 #define amx_Address(amx,addr) \ 00413 (cell*)(((int32_t)((amx)->data ? (amx)->data : (amx)->code) & ~CELLMASK) | ((int32_t)(addr) & CELLMASK)) 00414 #else 00415 #define amx_Address(amx,addr) ((void)(amx),(cell*)(addr)) 00416 #endif 00417 00418 #if defined __STDC_VERSION__ && __STDC_VERSION__ >= 199901L 00419 /* C99: use variable-length arrays */ 00420 #define amx_StrParam_Type(amx,param,result,type) \ 00421 int result##_length_; \ 00422 amx_StrLen(amx_Address(amx,param),&result##_length_); \ 00423 char result##_vla_[(result##_length_+1)*sizeof(*(result))]; \ 00424 (result)=(type)result##_vla_; \ 00425 amx_GetString((char*)(result),amx_Address(amx,param), \ 00426 sizeof(*(result))>1,result##_length_+1) 00427 #define amx_StrParam(amx,param,result) \ 00428 amx_StrParam_Type(amx,param,result,void*) 00429 #else 00430 /* macro using alloca() */ 00431 #define amx_StrParam_Type(amx,param,result,type) \ 00432 do { \ 00433 int result##_length_; \ 00434 amx_StrLen(amx_Address(amx,param),&result##_length_); \ 00435 if (result##_length_>0 && \ 00436 ((result)=(type)alloca((result##_length_+1)*sizeof(*(result))))!=NULL) \ 00437 amx_GetString((char*)(result),amx_Address(amx,param), \ 00438 sizeof(*(result))>1,result##_length_+1); \ 00439 else (result) = NULL; \ 00440 } while (0) 00441 #define amx_StrParam(amx,param,result) \ 00442 amx_StrParam_Type(amx,param,result,void*) 00443 #endif 00444 00445 uint16_t * AMXAPI amx_Align16(uint16_t *v); 00446 uint32_t * AMXAPI amx_Align32(uint32_t *v); 00447 #if defined _I64_MAX || defined INT64_MAX || defined HAVE_I64 00448 uint64_t * AMXAPI amx_Align64(uint64_t *v); 00449 #endif 00450 int AMXAPI amx_Allot(AMX *amx, int cells, cell **address); 00451 int AMXAPI amx_Callback(AMX *amx, cell index, cell *result, const cell *params); 00452 int AMXAPI amx_Cleanup(AMX *amx); 00453 int AMXAPI amx_Clone(AMX *amxClone, AMX *amxSource, void *data); 00454 int AMXAPI amx_Exec(AMX *amx, cell *retval, int index); 00455 int AMXAPI amx_FindNative(AMX *amx, const char *name, int *index); 00456 int AMXAPI amx_FindPublic(AMX *amx, const char *name, int *index); 00457 int AMXAPI amx_FindPubVar(AMX *amx, const char *name, cell **address); 00458 int AMXAPI amx_FindTagId(AMX *amx, cell tag_id, char *tagname); 00459 int AMXAPI amx_Flags(AMX *amx,uint16_t *flags); 00460 int AMXAPI amx_GetNative(AMX *amx, int index, char *name); 00461 int AMXAPI amx_GetPublic(AMX *amx, int index, char *name, ucell *address); 00462 int AMXAPI amx_GetPubVar(AMX *amx, int index, char *name, cell **address); 00463 int AMXAPI amx_GetString(char *dest,const cell *source, int use_wchar, size_t size); 00464 int AMXAPI amx_GetTag(AMX *amx, int index, char *tagname, cell *tag_id); 00465 int AMXAPI amx_GetUserData(AMX *amx, long tag, void **ptr); 00466 int AMXAPI amx_Init(AMX *amx, void *program); 00467 int AMXAPI amx_InitJIT(AMX *amx, void *reloc_table, void *native_code); 00468 int AMXAPI amx_MemInfo(AMX *amx, long *codesize, long *datasize, long *stackheap); 00469 int AMXAPI amx_NameLength(AMX *amx, int *length); 00470 AMX_NATIVE_INFO * AMXAPI amx_NativeInfo(const char *name, AMX_NATIVE func); 00471 int AMXAPI amx_NumNatives(AMX *amx, int *number); 00472 int AMXAPI amx_NumPublics(AMX *amx, int *number); 00473 int AMXAPI amx_NumPubVars(AMX *amx, int *number); 00474 int AMXAPI amx_NumTags(AMX *amx, int *number); 00475 int AMXAPI amx_Push(AMX *amx, cell value); 00476 int AMXAPI amx_PushAddress(AMX *amx, cell *address); 00477 int AMXAPI amx_PushArray(AMX *amx, cell **address, const cell array[], int numcells); 00478 int AMXAPI amx_PushString(AMX *amx, cell **address, const char *string, int pack, int use_wchar); 00479 int AMXAPI amx_RaiseError(AMX *amx, int error); 00480 int AMXAPI amx_Register(AMX *amx, const AMX_NATIVE_INFO *nativelist, int number); 00481 int AMXAPI amx_Release(AMX *amx, cell *address); 00482 int AMXAPI amx_SetCallback(AMX *amx, AMX_CALLBACK callback); 00483 int AMXAPI amx_SetDebugHook(AMX *amx, AMX_DEBUG debug); 00484 int AMXAPI amx_SetString(cell *dest, const char *source, int pack, int use_wchar, size_t size); 00485 int AMXAPI amx_SetUserData(AMX *amx, long tag, void *ptr); 00486 int AMXAPI amx_StrLen(const cell *cstring, int *length); 00487 int AMXAPI amx_UTF8Check(const char *string, int *length); 00488 int AMXAPI amx_UTF8Get(const char *string, const char **endptr, cell *value); 00489 int AMXAPI amx_UTF8Len(const cell *cstr, int *length); 00490 int AMXAPI amx_UTF8Put(char *string, char **endptr, int maxchars, cell value); 00491 00492 #if PAWN_CELL_SIZE==16 00493 #define amx_AlignCell(v) amx_Align16(v) 00494 #elif PAWN_CELL_SIZE==32 00495 #define amx_AlignCell(v) amx_Align32(v) 00496 #elif PAWN_CELL_SIZE==64 && (defined _I64_MAX || defined INT64_MAX || defined HAVE_I64) 00497 #define amx_AlignCell(v) amx_Align64(v) 00498 #else 00499 #error Unsupported cell size 00500 #endif 00501 00502 #define amx_RegisterFunc(amx, name, func) \ 00503 amx_Register((amx), amx_NativeInfo((name),(func)), 1); 00504 00505 #if !defined AMX_NO_ALIGN 00506 #if defined __LINUX__ || defined __FreeBSD__ 00507 #pragma pack() /* reset default packing */ 00508 #elif defined MACOS && defined __MWERKS__ 00509 #pragma options align=reset 00510 #else 00511 #pragma pack(pop) /* reset previous packing */ 00512 #endif 00513 #endif 00514 00515 #ifdef __cplusplus 00516 } 00517 #endif 00518 00519 #endif /* AMX_H_INCLUDED */
Generated on Sat Jul 16 2022 16:09:35 by
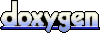