
Hangman game using qp a 16x2 LCD and joystick.
Embed:
(wiki syntax)
Show/hide line numbers
bsp.cpp
00001 #include "qp_port.h" 00002 #include "hangman.h" 00003 #include "bsp.h" 00004 #include "LPC17xx.h" 00005 #include "mbed.h" 00006 00007 Q_DEFINE_THIS_FILE 00008 00009 enum ISR_Priorities { // ISR priorities from highest urgency to lowest 00010 BUTTON_PRIO, 00011 SYSTICK_PRIO, 00012 }; 00013 00014 00015 #define LED_PORT LPC_GPIO1 00016 #define LED1_BIT (1U << 18) 00017 #define LED2_BIT (1U << 20) 00018 #define LED3_BIT (1U << 21) 00019 #define LED4_BIT (1U << 23) 00020 00021 //............................................................................ 00022 extern "C" void SysTick_Handler(void) { 00023 QK_ISR_ENTRY(); // inform the QK kernel of entering the ISR 00024 00025 #ifdef Q_SPY 00026 uint32_t volatile dummy = SysTick->CTRL; // clear the COUNTFLAG in SysTick 00027 l_tickTime += l_tickPeriod; // account for the clock rollover 00028 #endif 00029 00030 QF::TICK(&l_SysTick_Handler); // process all armed time events 00031 00032 QK_ISR_EXIT(); // inform the QK kernel of exiting the ISR 00033 } 00034 00035 //............................................................................ 00036 void BSP_init(void) { 00037 SystemInit(); // initialize the clocking system 00038 00039 // set LED port to output 00040 LED_PORT->FIODIR |= (LED1_BIT | LED2_BIT | LED3_BIT | LED4_BIT); 00041 00042 // clear the LEDs 00043 LED_PORT->FIOCLR = (LED1_BIT | LED2_BIT | LED3_BIT | LED4_BIT); 00044 00045 QS_OBJ_DICTIONARY(&l_SysTick_Handler); 00046 } 00047 00048 //............................................................................ 00049 void BSP_lcdScrollIn(char* line1, char* line2) { 00050 // scroller algorithm 00051 lcd.cls(); 00052 char output1[17]; 00053 char output2[17]; 00054 for ( int i = 0; i < 17; i++) { 00055 output1[i] = ' '; 00056 output2[i] = ' '; 00057 } 00058 for (int i = 0; i < 17; i++) { 00059 for (int col = 0; col < 17; col++) { 00060 00061 if (col >= i) { 00062 output1[col] = ' '; 00063 output2[col] = ' '; 00064 } else { 00065 output1[col] = (line1[col+(16-i)]); 00066 output2[col] = (line2[col+(16-i)]); 00067 } 00068 lcd.locate(col,0); 00069 lcd.putc(output1[col]); 00070 lcd.locate(col,1); 00071 lcd.putc(output2[col]); 00072 } 00073 wait(0.4); 00074 } 00075 } 00076 00077 //............................................................................ 00078 void BSP_lcdUpdate(char* line1,char* line2) { 00079 uint8_t length1 = strlen(line1); 00080 uint8_t length2 = strlen(line2); 00081 if (length1 > length2) 00082 length1 = length2; 00083 lcd.cls(); 00084 if (length1 < 17) 00085 for (int col = 0; col < 17 && col < length1; col++) { 00086 lcd.locate(col,0); 00087 lcd.putc(*(line1+col)); 00088 lcd.locate(col,1); 00089 lcd.putc(*(line2+col)); 00090 } 00091 else 00092 for (int col = 0; col < 17; col++) { 00093 lcd.locate(col,0); 00094 lcd.putc(*(line1+col)); 00095 lcd.locate(col,1); 00096 lcd.putc(*(line2+col)); 00097 } 00098 } 00099 00100 //............................................................................ 00101 void QF::onStartup(void) { 00102 // set up the SysTick timer to fire at BSP_TICKS_PER_SEC rate 00103 SysTick_Config(SystemCoreClock / BSP_TICKS_PER_SEC); 00104 00105 // set priorities of all interrupts in the system... 00106 NVIC_SetPriority(SysTick_IRQn, SYSTICK_PRIO); 00107 //NVIC_SetPriority(EINT0_IRQn, GPIOPORTA_PRIO); 00108 00109 //NVIC_EnableIRQ(EINT0_IRQn); 00110 } 00111 //............................................................................ 00112 void QF::onCleanup(void) { 00113 } 00114 //............................................................................ 00115 void QK::onIdle(void) { 00116 00117 QF_INT_LOCK(dummy); 00118 LED_PORT->FIOSET = LED4_BIT; // turn the LED4 on 00119 __NOP(); // delay a bit to see some light intensity 00120 __NOP(); 00121 __NOP(); 00122 __NOP(); 00123 LED_PORT->FIOCLR = LED4_BIT; // turn the LED4 off 00124 QF_INT_UNLOCK(dummy); 00125 00126 __WFI(); 00127 } 00128 00129 //............................................................................ 00130 void Q_onAssert(char const Q_ROM * const Q_ROM_VAR file, int line) { 00131 (void)file; // avoid compiler warning 00132 (void)line; // avoid compiler warning 00133 QF_INT_LOCK(dummy); // make sure that all interrupts are disabled 00134 // light up all LEDs 00135 LED_PORT->FIOSET = (LED1_BIT | LED2_BIT | LED3_BIT | LED4_BIT); 00136 00137 for (;;) { // NOTE: replace the loop with reset for final version 00138 } 00139 }
Generated on Wed Jul 13 2022 20:02:05 by
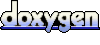