UART1 buffered serial driver, requires RTOS
Dependents: Serial_interrupts_buffered HARP2 HARP3
buffered_serial.h
00001 /* 00002 * @file buffered_serial.h 00003 * @author Tyler Weaver 00004 * 00005 * @section LICENSE 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00008 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00009 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00010 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in all copies or 00014 * substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00017 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00018 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00019 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * @section DESCRIPTION 00023 * 00024 * Buffered serial UART1 - Setup to work with UART1 (p13,p14) 00025 * 00026 * Uses RTOS to block current thread. 00027 */ 00028 00029 #ifndef BUFFERED_SERIAL_H 00030 #define BUFFERED_SERIAL_H 00031 00032 #define BUFFER_SIZE 255 00033 #define LINE_SIZE 80 00034 #define NEXT(x) ((x+1)&BUFFER_SIZE) 00035 #define IS_TX_FULL (((tx_in_ + 1) & BUFFER_SIZE) == tx_out_) 00036 #define IS_RX_FULL (((rx_in_ + 1) & BUFFER_SIZE) == rx_out_) 00037 #define IS_RX_EMPTY (rx_in_ == rx_out_) 00038 00039 #include "mbed.h" 00040 #include "rtos.h" 00041 /** Buffered serial UART1 - Setup to work with UART1 (p13,p14) 00042 * 00043 * Uses RTOS to block current thread. 00044 */ 00045 class BufferedSerial : public Serial 00046 { 00047 public: 00048 /** Default Constructor 00049 * Initialize UART1 - Serial(p13,p14) 00050 * Initialize Semaphores 00051 * Attach Serial Interrupts 00052 */ 00053 BufferedSerial(); 00054 00055 /** Put cstring in buffer/output 00056 * 00057 * @param cstring to put in buffer for printing (max length = 80 characters) 00058 */ 00059 void put_line(char*); 00060 00061 /** Gets a cstring from the buffer/input 00062 * 00063 * @param buffer cstring to put line from buffer in (ends at '\n' or 80 characters) 00064 */ 00065 void get_line(char*); 00066 00067 private: 00068 void Tx_interrupt(); 00069 void Rx_interrupt(); 00070 00071 // for disabling the irq 00072 IRQn device_irqn; 00073 00074 // Circular buffers for serial TX and RX data - used by interrupt routines 00075 // might need to increase buffer size for high baud rates 00076 char tx_buffer_[BUFFER_SIZE]; 00077 char rx_buffer_[BUFFER_SIZE]; 00078 // Circular buffer pointers 00079 // volatile makes read-modify-write atomic 00080 volatile int tx_in_; 00081 volatile int tx_out_; 00082 volatile int rx_in_; 00083 volatile int rx_out_; 00084 // Line buffers for sprintf and sscanf 00085 char tx_line_[LINE_SIZE]; 00086 char rx_line_[LINE_SIZE]; 00087 00088 //DigitalOut led1; // debug 00089 //DigitalOut led2; 00090 00091 Semaphore rx_sem_; 00092 Semaphore tx_sem_; 00093 }; 00094 00095 #endif
Generated on Sat Jul 16 2022 17:21:01 by
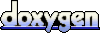