Built from datasheet for 9-DOF sensor stick from SparkFun.
Dependents: 9Dof_unit_testing sparkfun6dof Seeed_Grove_Digital_Compass_Example CompassTest ... more
HMC5883L Class Reference
The HMC5883L 3-Axis Digital Compass IC. More...
#include <HMC5883L.h>
Public Member Functions | |
HMC5883L (PinName sda, PinName scl) | |
Constructor. | |
HMC5883L (I2C &i2c) | |
Constructor that accepts external i2c interface object. | |
void | init () |
Initalize function called by all constructors. | |
void | setConfigurationA (char) |
Function for setting configuration register A. | |
char | getConfigurationA () |
Function for retrieving the contents of configuration register A. | |
void | setConfigurationB (char) |
Function for setting configuration register B. | |
char | getConfigurationB () |
Function for retrieving the contents of configuration register B. | |
void | setMode (char) |
Funciton for setting the mode register. | |
char | getMode () |
Function for retrieving the contents of mode register. | |
void | getXYZ (int16_t raw[3]) |
Function for retriaval of the raw data. | |
char | getStatus () |
Function for retrieving the contents of status register. | |
double | getHeadingXY () |
Function for getting radian heading using 2-dimensional calculation. | |
double | getHeadingXYDeg () |
Function for getting degree heading using 2-dimensional calculation. | |
Static Public Attributes | |
static const int | I2C_ADDRESS = 0x3D |
The I2C address that can be passed directly to i2c object (it's already shifted 1 bit left). |
Detailed Description
The HMC5883L 3-Axis Digital Compass IC.
Definition at line 89 of file HMC5883L.h.
Constructor & Destructor Documentation
HMC5883L | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor.
Calls init function
- Parameters:
-
sda - mbed pin to use for the SDA I2C line. scl - mbed pin to use for the SCL I2C line.
Definition at line 35 of file HMC5883L.cpp.
HMC5883L | ( | I2C & | i2c ) |
Constructor that accepts external i2c interface object.
Calls init function
- Parameters:
-
i2c The I2C interface object to use.
Definition at line 116 of file HMC5883L.h.
Member Function Documentation
char getConfigurationA | ( | ) |
Function for retrieving the contents of configuration register A.
- Returns:
- Configuration Register A
Definition at line 76 of file HMC5883L.cpp.
char getConfigurationB | ( | ) |
Function for retrieving the contents of configuration register B.
- Returns:
- Configuration Register B
Definition at line 85 of file HMC5883L.cpp.
double getHeadingXY | ( | ) |
Function for getting radian heading using 2-dimensional calculation.
Compass must be held flat and away from an magnetic field generating devices such as cell phones and speakers.
TODO: declenation angle compensation
- Returns:
- heading in radians
Definition at line 132 of file HMC5883L.cpp.
double getHeadingXYDeg | ( | ) |
Function for getting degree heading using 2-dimensional calculation.
Compass must be held flat and away from an magnetic field generating devices such as cell phones and speakers.
TODO: declenation angle compensation
- Returns:
- heading in degrees
Definition at line 232 of file HMC5883L.h.
char getMode | ( | ) |
Function for retrieving the contents of mode register.
- Returns:
- mode register
Definition at line 102 of file HMC5883L.cpp.
char getStatus | ( | ) |
Function for retrieving the contents of status register.
Bit1: LOCK, Bit0: RDY
- Returns:
- status register
Definition at line 111 of file HMC5883L.cpp.
void getXYZ | ( | int16_t | raw[3] ) |
Function for retriaval of the raw data.
- Parameters:
-
output buffer that is atleast 3 in length
Definition at line 120 of file HMC5883L.cpp.
void init | ( | ) |
Initalize function called by all constructors.
Place startup code in here.
Definition at line 50 of file HMC5883L.cpp.
void setConfigurationA | ( | char | config ) |
Function for setting configuration register A.
Defined constants should be ored together to create value. Defualt is 0x10 - 1 Sample per output, 15Hz Data output rate, normal measurement mode
Refer to datasheet for instructions for setting Configuration Register A.
- Parameters:
-
config the value to place in Configuration Register A
Definition at line 58 of file HMC5883L.cpp.
void setConfigurationB | ( | char | config ) |
Function for setting configuration register B.
Configuration Register B is for setting the device gain. Default value is 0x20
Refer to datasheet for instructions for setting Configuration Register B
- Parameters:
-
config the value to place in Configuration Register B
Definition at line 67 of file HMC5883L.cpp.
void setMode | ( | char | mode = SINGLE_MODE ) |
Funciton for setting the mode register.
Constants: CONTINUOUS_MODE, SINGLE_MODE, IDLE_MODE
When you send a the Single-Measurement Mode instruction to the mode register a single measurement is made, the RDY bit is set in the status register, and the mode is placed in idle mode.
When in Continous-Measurement Mode the device continuously performs measurements and places the results in teh data register. After being placed in this mode it takes two periods at the rate set in the data output rate before the first sample is avaliable.
Refer to datasheet for more detailed instructions for setting the mode register.
- Parameters:
-
mode the value for setting in the Mode Register
Definition at line 94 of file HMC5883L.cpp.
Field Documentation
const int I2C_ADDRESS = 0x3D [static] |
The I2C address that can be passed directly to i2c object (it's already shifted 1 bit left).
Definition at line 97 of file HMC5883L.h.
Generated on Fri Jul 15 2022 18:52:04 by
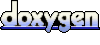