A GPS serial interrupt service routine that has an on the fly nmea parser. Works with a STM32F411RE and a Adafruit GPS logger.
Dependents: Bicycl_Computer_NUCLEO-F411RE Bicycl_Computer_NUCLEO-L476RG
Fork of GPS by
nmea.h
00001 /* 00002 File: nmea.h 00003 Version: 0.1.0 00004 Date: Feb. 23, 2013 00005 License: GPL v2 00006 00007 NMEA GPS content parser 00008 00009 **************************************************************************** 00010 Copyright (C) 2013 Radu Motisan <radu.motisan@gmail.com> 00011 00012 http://www.pocketmagic.net 00013 00014 This program is free software; you can redistribute it and/or modify 00015 it under the terms of the GNU General Public License as published by 00016 the Free Software Foundation; either version 2 of the License, or 00017 (at your option) any later version. 00018 00019 This program is distributed in the hope that it will be useful, 00020 but WITHOUT ANY WARRANTY; without even the implied warranty of 00021 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00022 GNU General Public License for more details. 00023 00024 You should have received a copy of the GNU General Public License 00025 along with this program; if not, write to the Free Software 00026 Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00027 **************************************************************************** 00028 */ 00029 //#include "../timeout.h" 00030 00031 00032 00033 class NMEA { 00034 00035 private: 00036 bool m_bFlagRead, // flag used by the parser, when a valid sentence has begun 00037 m_bFlagDataReady; // valid GPS fix and data available, user can call reader functions 00038 char tmp_words[20][15], // hold parsed words for one given NMEA sentence 00039 tmp_szChecksum[15]; // hold the received checksum for one given NMEA sentence 00040 00041 // will be set to true for characters between $ and * only 00042 bool m_bFlagComputedCks ; // used to compute checksum and indicate valid checksum interval (between $ and * in a given sentence) 00043 int m_nChecksum ; // numeric checksum, computed for a given sentence 00044 bool m_bFlagReceivedCks ; // after getting * we start cuttings the received checksum 00045 int index_received_checksum ; // used to parse received checksum 00046 00047 // word cutting variables 00048 int m_nWordIdx , // the current word in a sentence 00049 m_nPrevIdx, // last character index where we did a cut 00050 m_nNowIdx ; // current character index 00051 00052 // globals to store parser results 00053 float res_fLongitude; // GPRMC and GPGGA 00054 char res_clon; // E or W 00055 float res_fLatitude; // GPRMC and GPGGA 00056 char res_clat; // N or S 00057 unsigned char res_nUTCHour, res_nUTCMin, res_nUTCSec, // GPRMC and GPGGA 00058 res_nUTCDay, res_nUTCMonth, res_nUTCYear; // GPRMC 00059 int res_nSatellitesUsed; // GPGGA 00060 float res_fAltitude; // GPGGA 00061 float res_fSpeed; // GPRMC 00062 float res_fBearing; // GPRMC 00063 00064 // the parser, currently handling GPRMC and GPGGA, but easy to add any new sentences 00065 void parsedata(); 00066 // aux functions 00067 int digit2dec(char hexdigit); 00068 float string2float(char* s); 00069 int mstrcmp(const char *s1, const char *s2); 00070 float trunc(float v); 00071 00072 public: 00073 // constructor, initing a few variables 00074 NMEA() { 00075 m_bFlagRead = false; //are we in a sentence? 00076 m_bFlagDataReady = false; //is data available? 00077 } 00078 00079 /* 00080 * The serial data is assembled on the fly, without using any redundant buffers. 00081 * When a sentence is complete (one that starts with $, ending in EOL), all processing is done on 00082 * this temporary buffer that we've built: checksum computation, extracting sentence "words" (the CSV values), 00083 * and so on. 00084 * When a new sentence is fully assembled using the fusedata function, the code calls parsedata. 00085 * This function in turn, splits the sentences and interprets the data. Here is part of the parser function, 00086 * handling both the $GPRMC NMEA sentence: 00087 */ 00088 int fusedata(char c); 00089 00090 00091 // READER functions: retrieving results, call isdataready() first 00092 bool isdataready(); 00093 int getHour(); 00094 int getMinute(); 00095 int getSecond(); 00096 int getDay(); 00097 int getMonth(); 00098 int getYear(); 00099 float getLatitude(); 00100 char getlatc(); 00101 float getLongitude(); 00102 char getlonc(); 00103 int getSatellites(); 00104 float getAltitude(); 00105 float getSpeed(); 00106 float getBearing(); 00107 }; 00108
Generated on Tue Jul 12 2022 18:50:37 by
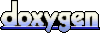