
AM2321 Temperature and Humidity Sensor mbed library Example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 AM2321 Temperature and Humidity Sensor 00003 mbed Sample code 00004 00005 Copyright (c) 2014 tomozh <tomozh@gmail.com> 00006 00007 This software is released under the MIT License. 00008 http://opensource.org/licenses/mit-license.php 00009 00010 Last update : 2014/05/06 00011 */ 00012 00013 #include "mbed.h" 00014 #include "AM2321.h" 00015 00016 Serial pc(USBTX, USBRX); // Tx, Rx 00017 AM2321 am2321(p28, p27); // SDA, SCL 00018 DigitalOut led1(LED1); 00019 00020 int main() 00021 { 00022 uint16_t count = 0; 00023 00024 while(1) 00025 { 00026 led1 = !led1; 00027 00028 if(am2321.poll()) 00029 { 00030 pc.printf( 00031 ":%05u,%.1f,%.1f\n" 00032 , count++ 00033 , am2321.getTemperature() 00034 , am2321.getHumidity() 00035 ); 00036 } 00037 00038 wait(0.5); 00039 } 00040 00041 /* 00042 output 00043 ----------------------- 00044 :01100,23.7,38.1 00045 :01101,23.6,38.0 00046 :01102,23.7,38.1 00047 :01103,23.6,38.0 00048 :01104,23.7,38.0 00049 :01105,23.7,38.1 00050 :01106,23.6,38.0 00051 :01107,23.7,38.0 00052 :01108,23.7,38.0 00053 :01109,23.6,38.0 00054 :01110,23.7,38.0 00055 */ 00056 }
Generated on Sat Jul 16 2022 05:24:43 by
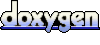