
Sample program for XBee remote API operation. This program get ADC sample data from remote XBee connected over the radio
main.cpp
00001 #include "mbed.h" 00002 #include "XBee.h" 00003 #include "TextLCD.h" 00004 00005 TextLCD lcd(p25, p26, p24, p23, p22, p21); // RS, E, DB4, DB5, DB6, DB7 00006 00007 /*-- AT command and parameters --*/ 00008 uint8_t atISCmd[] = {'I', 'S'}; // Forces a read of all enabled digital and analog input lines 00009 uint8_t atDBCmd[] = {'D', 'B'}; // Received Signal Strength 00010 uint8_t cmdVal0[] = {0}; // Clear RSSI regisger 00011 00012 /*-- Create instanse of Xbee object --*/ 00013 XBee xbee(p13, p14); 00014 XBeeAddress64 remoteAddress(0x0013A200, 0x406B7111); // Specify your XBee address 00015 00016 /*-- Create instanse of Command and Response object --*/ 00017 // Remot ATIS command to read ADC value (ADC3 is enabled by X-CTU tool) 00018 RemoteAtCommandRequest remoteSampleRequest(remoteAddress, atISCmd); 00019 // Local ATDB command to read signal strength (RSSI) 00020 AtCommandRequest atDB(atDBCmd); 00021 // Local ATDB0 command to clear RSSI 00022 AtCommandRequest atDB0(atDBCmd, cmdVal0, sizeof(cmdVal0)); 00023 // Create instanse to handle command response 00024 AtCommandResponse response = AtCommandResponse(); 00025 RemoteAtCommandResponse remoteResp = RemoteAtCommandResponse(); 00026 00027 00028 /* Receive command response packet 00029 * If OK response recieved, return pointer to the Response Data Frame 00030 */ 00031 uint8_t* GetResponse() { 00032 // Read response 00033 if (xbee.readPacket(5000)) { 00034 // Got a response! Check if response is AT command respose 00035 if (xbee.getResponse().getApiId() == AT_COMMAND_RESPONSE) { 00036 xbee.getResponse().getAtCommandResponse(response); 00037 if ( response.getStatus() == AT_OK ) 00038 return response.getValue(); 00039 } else if (xbee.getResponse().getApiId() == REMOTE_AT_COMMAND_RESPONSE) { 00040 xbee.getResponse().getRemoteAtCommandResponse(remoteResp); 00041 if ( remoteResp.getStatus() == AT_OK ) { 00042 // Debug print 00043 printf("Response Data:"); 00044 for (int i = 0; i < remoteResp.getValueLength(); i++) 00045 printf("%02X ", remoteResp.getValue()[i]); 00046 printf("\n"); 00047 00048 return remoteResp.getValue(); 00049 } else { 00050 printf("Remote Command Error:0x%X\n", response.getStatus()); 00051 } 00052 } 00053 } 00054 00055 return 0; 00056 } 00057 00058 00059 /* Get ADC data 00060 * Data frame structure of ATIS 00061 * Offset 00062 * 0 : Number of Samples (Always 1) 00063 * 1-2 : Digital Channel Mask 00064 * 3 : Analog Channel Mask 00065 * 4-5 : Digital Samples (Omit if no DIO enabled) 00066 * 6-7 : First ADC Data 00067 */ 00068 uint16_t getAnalog(uint8_t *FrameData, int ADC) { 00069 // ADC data feild starts 4 bytes offest, if no DIO enabled 00070 uint8_t start = 4; 00071 00072 // Contains Digital channel? 00073 if (FrameData[1] > 0 || FrameData[2] > 0) { 00074 // make room for digital i/o 00075 start+=2; 00076 } 00077 00078 // start depends on how many ADCs before this ADC are enabled 00079 for (int i = 0; i < ADC; i++) { 00080 // Is Analog channel Enabled ? 00081 if ( (FrameData[3] >> i) & 1 ) { 00082 start+=2; 00083 } 00084 } 00085 00086 return (uint16_t)((FrameData[start] << 8) + FrameData[start + 1]); 00087 } 00088 00089 00090 int main() { 00091 unsigned int loop = 0; 00092 00093 xbee.begin(9600); 00094 lcd.printf("RSSI:"); 00095 lcd.locate(0, 1); 00096 lcd.printf("ADC :"); 00097 printf("\nStart.\n"); 00098 00099 while (true) { 00100 uint8_t *responseVal; 00101 uint8_t rssiVal = 0; 00102 uint16_t adcVal = 0; 00103 00104 // Send ATDB command (Read RSSI register from local Xbee) 00105 xbee.send(atDB); 00106 responseVal = GetResponse(); 00107 if ( responseVal != 0 ) 00108 rssiVal = responseVal[0]; 00109 lcd.locate(5, 0); 00110 if (rssiVal == 0) 00111 lcd.printf("No Signal"); 00112 else 00113 lcd.printf("-%ddBm ", rssiVal); 00114 00115 // Clear RSSI register, because Xbee hold RSSI value of last received packet even after radio disconneded 00116 xbee.send(atDB0); 00117 GetResponse(); 00118 00119 // Read ADC3 value by sending ATIS command 00120 xbee.send(remoteSampleRequest); 00121 responseVal = GetResponse(); 00122 if ( responseVal != 0 ) { 00123 adcVal = getAnalog(responseVal, 3); // Assume ADC3 is enabled 00124 } 00125 lcd.locate(5, 1); 00126 if (adcVal == 0) 00127 lcd.printf("- "); 00128 else 00129 lcd.printf("%d", adcVal); 00130 00131 printf("Loop:%d\n", loop++); 00132 wait(1.0); 00133 } 00134 }
Generated on Fri Jul 15 2022 05:32:57 by
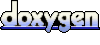