SPKDisplay - A mbed display class and processing imaging tools for 128x64 OLEDs using the SSD1305 driver, connected via SPI.
Dependents: SPK-DVIMXR SPK-DMXer
SPKDisplay Class Reference
Display class for 128x64 OLEDs using the SSD1305 driver, connected via SPI. More...
#include <spk_oled_ssd1305.h>
Public Member Functions | |
SPKDisplay (PinName mosi, PinName clk, PinName cs, PinName dc, PinName res, Serial *debugSerial=NULL) | |
Create a display object connected via SPI. | |
void | clearBuffer () |
Completely clear the object's display representation. | |
void | clearBufferRow (int row) |
Clear a row of the object's display representation. | |
void | imageToBuffer (const uint8_t *image) |
Replace the object display representation with the contents of image. | |
void | horizLineToBuffer (int y) |
Draw a horizontal line in the object's display representation. | |
void | textToBuffer (std::string message, int row) |
Write a line of text in the object's display representation Requires the font to have been set. | |
void | characterToBuffer (char character, int x, int row) |
Write a single character in the object's display representation Requires the font to have been set. | |
void | sendBuffer () |
Send the object's display representation to the OLED. | |
Data Fields | |
const int * | fontStartCharacter |
Font - Assign the ASCII value of the character at the start of the implemented range. | |
const int * | fontEndCharacter |
Font - Assign the ASCII value of the character at the end of the implemented range. | |
uint8_t const ** | fontCharacters |
Font - Assign the font, an array of 8x8px characters. |
Detailed Description
Display class for 128x64 OLEDs using the SSD1305 driver, connected via SPI.
Display ie. DENSITRON - DD-12864YO-3A
This is a ground-up, minimal library. Further functionality as and when its needed or anybody wants to contribute.
This library includes two processing sketches to create a font and full-screen image in the required byte representations. Without creating your font and any images, all this library will do is blank the screen and draw horizontal lines. But at least you'll know its working!
Terminology: 'rows' are 8 pixel high rows across the display, 0 being the topmost and 7 the bottom. 'lines' are single pixel lines, origin top left.
Example:
// Create object and load font SPKDisplay screen(p5, p7, p8, p10, p9) screen.fontStartCharacter = &myStartChar; screen.fontEndCharacter = &myEndChar; screen.fontCharacters = myFontArray; // Draw screen.imageToBuffer(myImageByteArray); screen.textToBuffer("*spark OLED SSD1305",0); screen.textToBuffer("v01",1); screen.sendBuffer
Definition at line 62 of file spk_oled_ssd1305.h.
Constructor & Destructor Documentation
SPKDisplay | ( | PinName | mosi, |
PinName | clk, | ||
PinName | cs, | ||
PinName | dc, | ||
PinName | res, | ||
Serial * | debugSerial = NULL |
||
) |
Create a display object connected via SPI.
- Parameters:
-
mosi SPI MOSI clk SPI SCK cs Chip Select - a digital out pin dc Data/Command - a digital out pin res Reset - a digital out pin debugSerial An optional serial object to log to
Definition at line 25 of file spk_oled_ssd1305.cpp.
Member Function Documentation
void characterToBuffer | ( | char | character, |
int | x, | ||
int | row | ||
) |
Write a single character in the object's display representation Requires the font to have been set.
- Parameters:
-
character The character to write. x The x position to draw the character row The row in which to write the character
Definition at line 160 of file spk_oled_ssd1305.cpp.
void clearBuffer | ( | ) |
Completely clear the object's display representation.
Definition at line 52 of file spk_oled_ssd1305.cpp.
void clearBufferRow | ( | int | row ) |
Clear a row of the object's display representation.
- Parameters:
-
row The row to clear.
Definition at line 64 of file spk_oled_ssd1305.cpp.
void horizLineToBuffer | ( | int | y ) |
Draw a horizontal line in the object's display representation.
- Parameters:
-
y The y position of the line to draw
Definition at line 83 of file spk_oled_ssd1305.cpp.
void imageToBuffer | ( | const uint8_t * | image ) |
Replace the object display representation with the contents of image.
- Parameters:
-
image An array of 1056 bytes representing an image.
- Note:
- The processing sketch spk_oled_screenByteMaker--processing takes 132x64 images and creates the code to declare such arrays
Definition at line 58 of file spk_oled_ssd1305.cpp.
void sendBuffer | ( | ) |
Send the object's display representation to the OLED.
You can safely call this once per main loop, it will only transmit the buffer contents if there has been an update
Definition at line 198 of file spk_oled_ssd1305.cpp.
void textToBuffer | ( | std::string | message, |
int | row | ||
) |
Write a line of text in the object's display representation Requires the font to have been set.
- Parameters:
-
message The text to write. The text will be truncated if longer than the screen's width. row The row in which to write the text
Definition at line 106 of file spk_oled_ssd1305.cpp.
Field Documentation
uint8_t const** fontCharacters |
Font - Assign the font, an array of 8x8px characters.
- Note:
- The processing sketch spk_oled_fontByteMaker--processing takes characterCount*8px x 8px images and creates the code to declare the font array needed by this method
Definition at line 86 of file spk_oled_ssd1305.h.
const int* fontEndCharacter |
Font - Assign the ASCII value of the character at the end of the implemented range.
Definition at line 80 of file spk_oled_ssd1305.h.
const int* fontStartCharacter |
Font - Assign the ASCII value of the character at the start of the implemented range.
Definition at line 77 of file spk_oled_ssd1305.h.
Generated on Fri Jul 15 2022 01:46:06 by
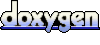