
Mutex Example
Fork of rtos_mutex by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 00004 DigitalOut led1(LED1); 00005 DigitalOut led2(LED2); 00006 00007 Mutex led_mutex; 00008 00009 void delay(void){ 00010 for(int i=0;i<65000;i++){ 00011 for(int j=0;j<100;j++) 00012 { 00013 } 00014 } 00015 } 00016 00017 void test_thread1(void const *args) { 00018 while (true) { 00019 led_mutex.lock(); 00020 printf("thd1 toogling\n"); 00021 led1 = !led1; 00022 delay(); 00023 led_mutex.unlock(); 00024 Thread::wait(1000); 00025 } 00026 } 00027 00028 void test_thread2(void const *args) { 00029 while (true) { 00030 led_mutex.lock(); 00031 printf("thd2 toogling\n"); 00032 led1 = !led1; 00033 delay(); 00034 led_mutex.unlock(); 00035 Thread::wait(1000); 00036 } 00037 } 00038 void test_thread(void const *args) { 00039 while (true) { 00040 led_mutex.lock(); 00041 printf("main toogling\n"); 00042 led1 = !led1; 00043 delay(); 00044 led_mutex.unlock(); 00045 Thread::wait(1000); 00046 } 00047 } 00048 00049 int main() { 00050 Thread t2(test_thread1, (void *)"Th 1"); 00051 Thread t3(test_thread2, (void *)"Th 2"); 00052 00053 test_thread((void *)"main"); 00054 }
Generated on Sat Aug 6 2022 09:07:47 by
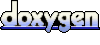