
Error 230
Dependencies: BLE_API mbed nRF51822
Fork of BLE_HeartRate by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLEDevice.h" 00019 #include "ble_hrs.h" 00020 #include "ble_hts.h" 00021 00022 BLEDevice ble; 00023 DigitalOut led1(LED1); 00024 00025 #define NEED_CONSOLE_OUTPUT 0 /* Set this if you need debug messages on the console; 00026 * it will have an impact on code-size and power consumption. */ 00027 00028 #if NEED_CONSOLE_OUTPUT 00029 Serial pc(USBTX, USBRX); 00030 #define DEBUG(...) { pc.printf(__VA_ARGS__); } 00031 #else 00032 #define DEBUG(...) /* nothing */ 00033 #endif /* #if NEED_CONSOLE_OUTPUT */ 00034 00035 const static char DEVICE_NAME[] = "Rob's Tool"; 00036 00037 /* Heart Rate Service */ 00038 /* Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.heart_rate.xml */ 00039 /* HRM Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml */ 00040 /* Location: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.body_sensor_location.xml */ 00041 00042 /* Temperature Service */ 00043 /* Service: https://developer.bluetooth.org/gatt/services/Pages/ServiceViewer.aspx?u=org.bluetooth.service.health_thermometer.xml */ 00044 /* Temp Char: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.temperature_measurement.xml */ 00045 /* Location: https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.temperature_type.xml */ 00046 00047 /* Heart Rate 1 */ 00048 static uint8_t bpm1[2] = {0x00, 80}; 00049 GattCharacteristic hrmRate1(GattCharacteristic::UUID_HEART_RATE_MEASUREMENT_CHAR, bpm1, sizeof(bpm1), sizeof(bpm1), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00050 00051 static uint8_t location1 = BLE_HRS_BODY_SENSOR_LOCATION_EAR_LOBE; 00052 GattCharacteristic hrmLocation1(GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, (uint8_t *)&location1, sizeof(location1), sizeof(location1), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00053 00054 GattCharacteristic *hrmChars1[] = {&hrmRate1, &hrmLocation1, }; 00055 GattService hrmService1(GattService::UUID_HEART_RATE_SERVICE, hrmChars1, sizeof(hrmChars1) / sizeof(GattCharacteristic *)); 00056 00057 /* Heart Rate 2 */ 00058 static uint8_t bpm2[2] = {0x00, 80}; 00059 GattCharacteristic hrmRate2(GattCharacteristic::UUID_HEART_RATE_MEASUREMENT_CHAR, bpm2, sizeof(bpm2), sizeof(bpm2), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00060 00061 static uint8_t location2 = BLE_HRS_BODY_SENSOR_LOCATION_HAND; 00062 GattCharacteristic hrmLocation2(GattCharacteristic::UUID_BODY_SENSOR_LOCATION_CHAR, (uint8_t *)&location2, sizeof(location2), sizeof(location2), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00063 00064 GattCharacteristic *hrmChars2[] = {&hrmRate2, &hrmLocation2, }; 00065 GattService hrmService2(GattService::UUID_HEART_RATE_SERVICE, hrmChars2, sizeof(hrmChars2) / sizeof(GattCharacteristic *)); 00066 00067 00068 /* Temperature */ 00069 static uint8_t temp[5] = {0, 0, 0, 0, 0}; 00070 GattCharacteristic recTemp(GattCharacteristic::UUID_TEMPERATURE_MEASUREMENT_CHAR, temp, sizeof(temp), sizeof(temp), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE); 00071 00072 static uint8_t type = BLE_HTS_TEMP_TYPE_RECTUM; 00073 GattCharacteristic recType(GattCharacteristic::UUID_TEMPERATURE_TYPE_CHAR, (uint8_t *)&type, sizeof(type), sizeof(type), GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00074 00075 GattCharacteristic *recChars[] = {&recTemp, &recType, }; 00076 GattService recService(GattService::UUID_HEALTH_THERMOMETER_SERVICE, recChars, sizeof(recChars) / sizeof(GattCharacteristic *)); 00077 00078 /* List */ 00079 static const uint16_t uuid16_list[] = {GattService::UUID_HEART_RATE_SERVICE, GattService::UUID_HEART_RATE_SERVICE, GattService::UUID_HEALTH_THERMOMETER_SERVICE}; 00080 00081 static volatile bool triggerSensorPolling = false; /* set to high periodically to indicate to the main thread that 00082 * polling is necessary. */ 00083 static Gap::ConnectionParams_t connectionParams; 00084 00085 void disconnectionCallback(Gap::Handle_t handle) 00086 { 00087 DEBUG("Disconnected handle %u!\n\r", handle); 00088 DEBUG("Restarting the advertising process\n\r"); 00089 ble.startAdvertising(); 00090 } 00091 00092 void onConnectionCallback(Gap::Handle_t handle) 00093 { 00094 DEBUG("connected. Got handle %u\r\n", handle); 00095 00096 connectionParams.slaveLatency = 1; 00097 if (ble.updateConnectionParams(handle, &connectionParams) != BLE_ERROR_NONE) { 00098 DEBUG("failed to update connection paramter\r\n"); 00099 } 00100 } 00101 00102 /** 00103 * Triggered periodically by the 'ticker' interrupt. 00104 */ 00105 void periodicCallback(void) 00106 { 00107 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00108 triggerSensorPolling = true; /* Note that the periodicCallback() executes in 00109 * interrupt context, so it is safer to do 00110 * heavy-weight sensor polling from the main 00111 * thread.*/ 00112 } 00113 00114 uint32_t quick_ieee11073_from_float(float temperature) 00115 { 00116 uint8_t exponent = 0xFF; //exponent is -1 00117 uint32_t mantissa = (uint32_t)(temperature*10); 00118 00119 return ( ((uint32_t)exponent) << 24) | mantissa; 00120 } 00121 00122 int main(void) 00123 { 00124 led1 = 1; 00125 Ticker ticker; 00126 ticker.attach(periodicCallback, 1); 00127 00128 DEBUG("Initialising the nRF51822\n\r"); 00129 ble.init(); 00130 ble.onDisconnection(disconnectionCallback); 00131 ble.onConnection(onConnectionCallback); 00132 00133 ble.getPreferredConnectionParams(&connectionParams); 00134 00135 /* setup advertising */ 00136 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00137 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t*)uuid16_list, sizeof(uuid16_list)); 00138 ble.accumulateAdvertisingPayload(GapAdvertisingData::HEART_RATE_SENSOR_HEART_RATE_BELT); 00139 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00140 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00141 ble.setAdvertisingInterval(80); /* 100ms; in multiples of 0.625ms. */ 00142 ble.startAdvertising(); 00143 00144 ble.addService(hrmService1); 00145 ble.addService(hrmService2); 00146 ble.addService(recService); 00147 00148 while (true) { 00149 if (triggerSensorPolling) { 00150 triggerSensorPolling = false; 00151 00152 /* Do blocking calls or whatever is necessary for sensor polling. */ 00153 /* In our case, we simply update the dummy HRM measurement. */ 00154 if (ble.getGapState().connected) { 00155 /* First byte = 8-bit values, no extra info, Second byte = uint8_t HRM value */ 00156 /* See --> https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.heart_rate_measurement.xml */ 00157 bpm1[1] = rand() % 40 + 60; 00158 ble.updateCharacteristicValue(hrmRate1.getHandle(), bpm1, sizeof(bpm1)); 00159 00160 bpm2[1] = rand() % 40 + 60; 00161 ble.updateCharacteristicValue(hrmRate2.getHandle(), bpm2, sizeof(bpm2)); 00162 00163 float f = (rand() % 20 + 360) / 10.0f; 00164 uint32_t tem = quick_ieee11073_from_float(f); 00165 memcpy(temp+1, &tem, 4); 00166 ble.updateCharacteristicValue(recTemp.getHandle(), temp, sizeof(temp)); 00167 } 00168 } else { 00169 ble.waitForEvent(); 00170 } 00171 } 00172 }
Generated on Wed Jul 13 2022 08:05:49 by
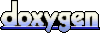