
An embedded server sending sensor information over the network to a remote client side process parsing the data
Dependencies: EthernetInterface NTPClient TimeInterface WebSocketClient mbed-rtos mbed ST_Events-old
data_logger.cpp
00001 //An embedded server sending sensor data to a client side process running on a PC for logging and analysis 00002 //TODO: Add in error handling and timeouts to deal with dropped connections and compile flags for testing 00003 00004 #include "data_logger.h" 00005 00006 EthernetInterface eth; 00007 NTPClient ntp; 00008 TimeInterface time_b; 00009 Timer t; 00010 time_t timestamp; //Built-in time class instance 00011 TCPSocketServer server; 00012 TCPSocketConnection client; 00013 EventQueue queue1; 00014 EventQueue queue2; 00015 Thread t1; 00016 Thread t2; 00017 Mutex m; 00018 00019 //setup 00020 DigitalOut led1(LED1); 00021 AnalogIn strainIn(A0); 00022 InterruptIn sig(p5); 00023 00024 //RtosTimer Timer(send_payload,osTimerPeriodic); 00025 00026 // main() runs in its own thread in the OS 00027 // (note the calls to Thread::wait below for delays) 00028 00029 int main(void) 00030 { 00031 eth.init(); //Use DHCP 00032 eth.setName("DL-test"); //set a hostname 00033 eth_cxn_status = eth.connect(); //Attempt an ethernet connection and save the status, add retries here in the future 00034 if(eth_cxn_status == 0) { 00035 //Bring up the ethernet interface 00036 printf("Detected Ethernet connection, entering network logging mode \n Server IP Address is %s\n", eth.getIPAddress()); 00037 server.bind(SERVER_PORT); 00038 server.listen(); //Wait for the client to connect 00039 server.accept(client); 00040 printf("Connection from: %s\n", client.get_address()); 00041 if (ntp.setTime("0.pool.ntp.org") == 0) //If time retrieval is successful 00042 { 00043 printf("Set time successfully\r\n"); 00044 00045 } 00046 else 00047 { 00048 printf("Unable to set time, error\r\n"); 00049 } 00050 } 00051 else { 00052 printf("Entering USB logging mode"); 00053 } 00054 00055 timestamp = time(NULL); 00056 queue1.call_every(RATE,&idle_report); 00057 t1.start(callback(&queue1, &EventQueue::dispatch_forever)); //Start the thread for periodic basline value reporting 00058 sig.rise(queue2.event(&cycle_time_isr_rise)); 00059 sig.fall(queue2.event(&cycle_time_isr_fall)); 00060 t2.start(callback(&queue2, &EventQueue::dispatch_forever)); //Start the cycle time and peak value calculation thread 00061 00062 } 00063 00064 void cycle_time_isr_rise(void) { 00065 t.start(); //Start the timer 00066 m.lock(); //Lock the mutex to prevent the baseline reporting thread from writing to the application buffer 00067 /*for(i=0;i<1023;i++) { 00068 samples[i] = pressureIn.read(); 00069 //add a mean and peak pressure calculation here and you may want to slow down the sample rate 00070 i++; 00071 }*/ 00072 //p_press = pressIn.read(); 00073 p_strain = strainCalc(); //Calculate strain while the machine is crushing 00074 } 00075 00076 void cycle_time_isr_fall(void) { 00077 t.stop(); //Stop the timer 00078 cycle_time = t.read(); 00079 t.reset(); //reset the timer 00080 if(eth_cxn_status == 0) { 00081 sprintf(appbuffer,"<payload><time>%s</time><cy_time>%f</cy_time><p_strain>%f</p_strain></payload>",time_b.ctime(×tamp), cycle_time, p_strain); 00082 } 00083 else { 00084 printf("Cycle time is: %f \n Strain detected during cycle is: %f% \r", cycle_time, p_strain); 00085 } 00086 sprintf(appbuffer,"\0"); //Nullify the string 00087 m.unlock(); //Unlock the mutex 00088 }; 00089 00090 void idle_report(void) { 00091 m.lock(); //Attempt to lock the mutex here 00092 id_strain = strainCalc(); //Calculate strain while the machine is idle 00093 if (eth_cxn_status == 0) { //Log data based on the available connection 00094 sprintf(appbuffer,"<payload><time>%s</time><id_strain>%f</id_strain></payload>",time_b.ctime(×tamp), id_strain); 00095 } 00096 else { 00097 printf("Strain while machine is idle: %f%",id_strain ); 00098 } 00099 00100 sprintf(appbuffer,"\0"); //Nullify the buffer 00101 m.unlock(); //Unlock the mutex 00102 } 00103 00104 float strainCalc(void) { 00105 float deltaR = strainIn/CURRENT; //Calculate Delta R 00106 float strain = (1/GAUGE_FACTOR)*(deltaR/NOMINAL_R); //Calculate strain, see equation reference in docs 00107 return strain; 00108 } 00109
Generated on Wed Jul 20 2022 12:19:43 by
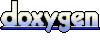