Opencv 3.1 project on GR-PEACH board
Fork of gr-peach-opencv-project by
Utility and system functions and macros
[Core functionality]
Data Structures | |
class | Allocator< _Tp > |
class | AutoBuffer< _Tp, fixed_size > |
Automatically Allocated Buffer Class. More... | |
class | ParallelLoopBody |
Base class for parallel data processors. More... | |
class | CommandLineParser |
Designed for command line parsing. More... | |
class | Exception |
Class passed to an error. More... | |
Modules | |
SSE utilities | |
NEON utilities | |
Enumerations | |
enum | CpuFeatures |
Available CPU features. More... | |
enum | SortFlags { SORT_EVERY_ROW = 0, SORT_EVERY_COLUMN = 1, SORT_ASCENDING = 0, SORT_DESCENDING = 16 } |
Functions | |
CV_EXPORTS void | error (int _code, const String &_err, const char *_func, const char *_file, int _line) |
Signals an error and raises the exception. | |
CV_INLINE CV_NORETURN void | errorNoReturn (int _code, const String &_err, const char *_func, const char *_file, int _line) |
same as cv::error, but does not return | |
CV_EXPORTS_W float | cubeRoot (float val) |
Computes the cube root of an argument. | |
CV_EXPORTS_W float | fastAtan2 (float y, float x) |
Calculates the angle of a 2D vector in degrees. | |
CV_EXPORTS int | LU (float *A, size_t astep, int m, float *b, size_t bstep, int n) |
proxy for hal::LU | |
CV_EXPORTS int | LU (double *A, size_t astep, int m, double *b, size_t bstep, int n) |
proxy for hal::LU | |
CV_EXPORTS bool | Cholesky (float *A, size_t astep, int m, float *b, size_t bstep, int n) |
proxy for hal::Cholesky | |
CV_EXPORTS bool | Cholesky (double *A, size_t astep, int m, double *b, size_t bstep, int n) |
proxy for hal::Cholesky | |
CV_EXPORTS void * | fastMalloc (size_t bufSize) |
Allocates an aligned memory buffer. | |
CV_EXPORTS void | fastFree (void *ptr) |
Deallocates a memory buffer. | |
CV_INLINE int | cvRound (double value) |
Rounds floating-point number to the nearest integer. | |
CV_INLINE int | cvFloor (double value) |
Rounds floating-point number to the nearest integer not larger than the original. | |
CV_INLINE int | cvCeil (double value) |
Rounds floating-point number to the nearest integer not smaller than the original. | |
CV_INLINE int | cvIsNaN (double value) |
Determines if the argument is Not A Number. | |
CV_INLINE int | cvIsInf (double value) |
Determines if the argument is Infinity. | |
CV_INLINE int | cvRound (float value) |
CV_INLINE int | cvRound (int value) |
CV_INLINE int | cvFloor (float value) |
CV_INLINE int | cvFloor (int value) |
CV_INLINE int | cvCeil (float value) |
CV_INLINE int | cvCeil (int value) |
CV_INLINE int | cvIsNaN (float value) |
CV_INLINE int | cvIsInf (float value) |
template<typename _Tp > | |
static _Tp | saturate_cast (uchar v) |
Template function for accurate conversion from one primitive type to another. | |
template<typename _Tp > | |
static _Tp | saturate_cast (schar v) |
template<typename _Tp > | |
static _Tp | saturate_cast (ushort v) |
template<typename _Tp > | |
static _Tp | saturate_cast (short v) |
template<typename _Tp > | |
static _Tp | saturate_cast (unsigned v) |
template<typename _Tp > | |
static _Tp | saturate_cast (int v) |
template<typename _Tp > | |
static _Tp | saturate_cast (float v) |
template<typename _Tp > | |
static _Tp | saturate_cast (double v) |
template<typename _Tp > | |
static _Tp | saturate_cast (int64 v) |
template<typename _Tp > | |
static _Tp | saturate_cast (uint64 v) |
bool | setBreakOnError (bool flag) |
Sets/resets the break-on-error mode. | |
CV_EXPORTS ErrorCallback | redirectError (ErrorCallback errCallback, void *userdata=0, void **prevUserdata=0) |
Sets the new error handler and the optional user data. | |
String | format (const char *fmt,...) |
Returns a text string formatted using the printf-like expression. | |
CV_EXPORTS_W void | setNumThreads (int nthreads) |
OpenCV will try to set the number of threads for the next parallel region. | |
CV_EXPORTS_W int | getNumThreads () |
Returns the number of threads used by OpenCV for parallel regions. | |
CV_EXPORTS_W int | getThreadNum () |
Returns the index of the currently executed thread within the current parallel region. | |
const String & | getBuildInformation () |
Returns full configuration time cmake output. | |
int64 | getTickCount () |
Returns the number of ticks. | |
double | getTickFrequency () |
Returns the number of ticks per second. | |
int64 | getCPUTickCount () |
Returns the number of CPU ticks. | |
bool | checkHardwareSupport (int feature) |
Returns true if the specified feature is supported by the host hardware. | |
CV_EXPORTS_W int | getNumberOfCPUs () |
Returns the number of logical CPUs available for the process. | |
template<typename _Tp > | |
static _Tp * | alignPtr (_Tp *ptr, int n=(int) sizeof(_Tp)) |
Aligns a pointer to the specified number of bytes. | |
static size_t | alignSize (size_t sz, int n) |
Aligns a buffer size to the specified number of bytes. | |
void | setUseOptimized (bool onoff) |
Enables or disables the optimized code. | |
bool | useOptimized () |
Returns the status of optimized code usage. | |
CV_EXPORTS void | parallel_for_ (const Range &range, const ParallelLoopBody &body, double nstripes=-1.) |
Parallel data processor. | |
void | error (const Exception &exc) |
Signals an error and raises the exception. | |
template<typename _Tp , typename Functor > | |
void | forEach_impl (const Functor &operation) |
Enumeration Type Documentation
enum CpuFeatures |
enum SortFlags |
- Enumerator:
Function Documentation
static _Tp* cv::alignPtr | ( | _Tp * | ptr, |
int | n = (int)sizeof(_Tp) |
||
) | [static] |
Aligns a pointer to the specified number of bytes.
The function returns the aligned pointer of the same type as the input pointer:
- Parameters:
-
ptr Aligned pointer. n Alignment size that must be a power of two.
Definition at line 302 of file utility.hpp.
static size_t cv::alignSize | ( | size_t | sz, |
int | n | ||
) | [static] |
Aligns a buffer size to the specified number of bytes.
The function returns the minimum number that is greater or equal to sz and is divisible by n :
- Parameters:
-
sz Buffer size to align. n Alignment size that must be a power of two.
Definition at line 314 of file utility.hpp.
CV_EXPORTS_W bool checkHardwareSupport | ( | int | feature ) |
Returns true if the specified feature is supported by the host hardware.
The function returns true if the host hardware supports the specified feature. When user calls setUseOptimized(false), the subsequent calls to checkHardwareSupport() will return false until setUseOptimized(true) is called. This way user can dynamically switch on and off the optimized code in OpenCV.
- Parameters:
-
feature The feature of interest, one of cv::CpuFeatures
Definition at line 376 of file system.cpp.
bool Cholesky | ( | double * | A, |
size_t | astep, | ||
int | m, | ||
double * | b, | ||
size_t | bstep, | ||
int | n | ||
) |
proxy for hal::Cholesky
Definition at line 68 of file lapack.cpp.
bool Cholesky | ( | float * | A, |
size_t | astep, | ||
int | m, | ||
float * | b, | ||
size_t | bstep, | ||
int | n | ||
) |
proxy for hal::Cholesky
Definition at line 63 of file lapack.cpp.
float cubeRoot | ( | float | val ) |
Computes the cube root of an argument.
The function cubeRoot computes . Negative arguments are handled correctly. NaN and Inf are not handled. The accuracy approaches the maximum possible accuracy for single-precision data.
- Parameters:
-
val A function argument.
Definition at line 130 of file mathfuncs.cpp.
CV_INLINE int cvCeil | ( | float | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 269 of file fast_math.hpp.
CV_INLINE int cvCeil | ( | int | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 286 of file fast_math.hpp.
CV_INLINE int cvCeil | ( | double | value ) |
Rounds floating-point number to the nearest integer not smaller than the original.
The function computes an integer i such that:
- Parameters:
-
value floating-point number. If the value is outside of INT_MIN ... INT_MAX range, the result is not defined.
Definition at line 158 of file fast_math.hpp.
CV_INLINE int cvFloor | ( | float | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 246 of file fast_math.hpp.
CV_INLINE int cvFloor | ( | int | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 263 of file fast_math.hpp.
CV_INLINE int cvFloor | ( | double | value ) |
Rounds floating-point number to the nearest integer not larger than the original.
The function computes an integer i such that:
- Parameters:
-
value floating-point number. If the value is outside of INT_MIN ... INT_MAX range, the result is not defined.
Definition at line 135 of file fast_math.hpp.
CV_INLINE int cvIsInf | ( | float | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 300 of file fast_math.hpp.
CV_INLINE int cvIsInf | ( | double | value ) |
Determines if the argument is Infinity.
- Parameters:
-
value The input floating-point value
The function returns 1 if the argument is a plus or minus infinity (as defined by IEEE754 standard) and 0 otherwise.
Definition at line 194 of file fast_math.hpp.
CV_INLINE int cvIsNaN | ( | float | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 292 of file fast_math.hpp.
CV_INLINE int cvIsNaN | ( | double | value ) |
Determines if the argument is Not A Number.
- Parameters:
-
value The input floating-point value
The function returns 1 if the argument is Not A Number (as defined by IEEE754 standard), 0 otherwise.
Definition at line 180 of file fast_math.hpp.
CV_INLINE int cvRound | ( | int | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 240 of file fast_math.hpp.
CV_INLINE int cvRound | ( | double | value ) |
Rounds floating-point number to the nearest integer.
- Parameters:
-
value floating-point number. If the value is outside of INT_MIN ... INT_MAX range, the result is not defined.
Definition at line 93 of file fast_math.hpp.
CV_INLINE int cvRound | ( | float | value ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 205 of file fast_math.hpp.
CV_EXPORTS void error | ( | const Exception & | exc ) |
Signals an error and raises the exception.
By default the function prints information about the error to stderr, then it either stops if cv::setBreakOnError() had been called before or raises the exception. It is possible to alternate error processing by using cv::redirectError().
- Parameters:
-
exc the exception raisen.
Definition at line 636 of file system.cpp.
void error | ( | int | _code, |
const String & | _err, | ||
const char * | _func, | ||
const char * | _file, | ||
int | _line | ||
) |
Signals an error and raises the exception.
By default the function prints information about the error to stderr, then it either stops if setBreakOnError() had been called before or raises the exception. It is possible to alternate error processing by using redirectError().
- Parameters:
-
_code - error code (Error::Code) _err - error description _func - function name. Available only when the compiler supports getting it _file - source file name where the error has occured _line - line number in the source file where the error has occured
- See also:
- CV_Error, CV_Error_, CV_ErrorNoReturn, CV_ErrorNoReturn_, CV_Assert, CV_DbgAssert
Definition at line 665 of file system.cpp.
CV_INLINE CV_NORETURN void cv::errorNoReturn | ( | int | _code, |
const String & | _err, | ||
const char * | _func, | ||
const char * | _file, | ||
int | _line | ||
) |
float fastAtan2 | ( | float | y, |
float | x | ||
) |
Calculates the angle of a 2D vector in degrees.
The function fastAtan2 calculates the full-range angle of an input 2D vector. The angle is measured in degrees and varies from 0 to 360 degrees. The accuracy is about 0.3 degrees.
- Parameters:
-
x x-coordinate of the vector. y y-coordinate of the vector.
Definition at line 103 of file mathfuncs.cpp.
void fastFree | ( | void * | ptr ) |
Deallocates a memory buffer.
The function deallocates the buffer allocated with fastMalloc . If NULL pointer is passed, the function does nothing. C version of the function clears the pointer *pptr* to avoid problems with double memory deallocation.
- Parameters:
-
ptr Pointer to the allocated buffer.
void * fastMalloc | ( | size_t | bufSize ) |
void forEach_impl | ( | const Functor & | operation ) | [protected, inherited] |
idx is modified in this->rowCall
Definition at line 360 of file utility.hpp.
CV_EXPORTS String format | ( | const char * | fmt, |
... | |||
) |
Returns a text string formatted using the printf-like expression.
The function acts like sprintf but forms and returns an STL string. It can be used to form an error message in the Exception constructor.
- Parameters:
-
fmt printf-compatible formatting specifiers.
Definition at line 527 of file system.cpp.
CV_EXPORTS_W const String & getBuildInformation | ( | ) |
Returns full configuration time cmake output.
Returned value is raw cmake output including version control system revision, compiler version, compiler flags, enabled modules and third party libraries, etc. Output format depends on target architecture.
Definition at line 519 of file system.cpp.
CV_EXPORTS_W int64 getCPUTickCount | ( | ) |
Returns the number of CPU ticks.
The function returns the current number of CPU ticks on some architectures (such as x86, x64, PowerPC). On other platforms the function is equivalent to getTickCount. It can also be used for very accurate time measurements, as well as for RNG initialization. Note that in case of multi-CPU systems a thread, from which getCPUTickCount is called, can be suspended and resumed at another CPU with its own counter. So, theoretically (and practically) the subsequent calls to the function do not necessary return the monotonously increasing values. Also, since a modern CPU varies the CPU frequency depending on the load, the number of CPU clocks spent in some code cannot be directly converted to time units. Therefore, getTickCount is generally a preferable solution for measuring execution time.
Definition at line 456 of file system.cpp.
int getNumberOfCPUs | ( | void | ) |
Returns the number of logical CPUs available for the process.
Definition at line 503 of file parallel.cpp.
int getNumThreads | ( | void | ) |
Returns the number of threads used by OpenCV for parallel regions.
Always returns 1 if OpenCV is built without threading support.
The exact meaning of return value depends on the threading framework used by OpenCV library:
- `TBB` – The number of threads, that OpenCV will try to use for parallel regions. If there is any tbb::thread_scheduler_init in user code conflicting with OpenCV, then function returns default number of threads used by TBB library.
- `OpenMP` – An upper bound on the number of threads that could be used to form a new team.
- `Concurrency` – The number of threads, that OpenCV will try to use for parallel regions.
- `GCD` – Unsupported; returns the GCD thread pool limit (512) for compatibility.
- `C=` – The number of threads, that OpenCV will try to use for parallel regions, if before called setNumThreads with threads > 0, otherwise returns the number of logical CPUs, available for the process.
- See also:
- setNumThreads, getThreadNum
Definition at line 330 of file parallel.cpp.
int getThreadNum | ( | void | ) |
Returns the index of the currently executed thread within the current parallel region.
Always returns 0 if called outside of parallel region.
The exact meaning of return value depends on the threading framework used by OpenCV library:
- `TBB` – Unsupported with current 4.1 TBB release. May be will be supported in future.
- `OpenMP` – The thread number, within the current team, of the calling thread.
- `Concurrency` – An ID for the virtual processor that the current context is executing on (0 for master thread and unique number for others, but not necessary 1,2,3,...).
- `GCD` – System calling thread's ID. Never returns 0 inside parallel region.
- `C=` – The index of the current parallel task.
- See also:
- setNumThreads, getNumThreads
Definition at line 438 of file parallel.cpp.
CV_EXPORTS_W int64 getTickCount | ( | ) |
Returns the number of ticks.
The function returns the number of ticks after the certain event (for example, when the machine was turned on). It can be used to initialize RNG or to measure a function execution time by reading the tick count before and after the function call. See also the tick frequency.
Definition at line 411 of file system.cpp.
CV_EXPORTS_W double getTickFrequency | ( | ) |
Returns the number of ticks per second.
The function returns the number of ticks per second. That is, the following code computes the execution time in seconds:
double t = (double)getTickCount(); // do something ... t = ((double)getTickCount() - t)/getTickFrequency();
Definition at line 431 of file system.cpp.
int LU | ( | float * | A, |
size_t | astep, | ||
int | m, | ||
float * | b, | ||
size_t | bstep, | ||
int | n | ||
) |
proxy for hal::LU
Definition at line 53 of file lapack.cpp.
int LU | ( | double * | A, |
size_t | astep, | ||
int | m, | ||
double * | b, | ||
size_t | bstep, | ||
int | n | ||
) |
proxy for hal::LU
Definition at line 58 of file lapack.cpp.
void parallel_for_ | ( | const Range & | range, |
const ParallelLoopBody & | body, | ||
double | nstripes = -1. |
||
) |
Parallel data processor.
Definition at line 253 of file parallel.cpp.
CV_EXPORTS ErrorCallback cv::redirectError | ( | ErrorCallback | errCallback, |
void * | userdata = 0 , |
||
void ** | prevUserdata = 0 |
||
) |
Sets the new error handler and the optional user data.
The function sets the new error handler, called from cv::error().
- Parameters:
-
errCallback the new error handler. If NULL, the default error handler is used. userdata the optional user data pointer, passed to the callback. prevUserdata the optional output parameter where the previous user data pointer is stored
- Returns:
- the previous error handler
static _Tp cv::saturate_cast | ( | int | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 92 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | uint64 | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 100 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | double | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 96 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | uchar | v ) | [static] |
Template function for accurate conversion from one primitive type to another.
The functions saturate_cast resemble the standard C++ cast operations, such as static_cast<T>() and others. They perform an efficient and accurate conversion from one primitive type to another (see the introduction chapter). saturate in the name means that when the input value v is out of the range of the target type, the result is not formed just by taking low bits of the input, but instead the value is clipped. For example:
uchar a = saturate_cast<uchar>(-100); // a = 0 (UCHAR_MIN) short b = saturate_cast<short>(33333.33333); // b = 32767 (SHRT_MAX)
Such clipping is done when the target type is unsigned char , signed char , unsigned short or signed short . For 32-bit integers, no clipping is done.
When the parameter is a floating-point value and the target type is an integer (8-, 16- or 32-bit), the floating-point value is first rounded to the nearest integer and then clipped if needed (when the target type is 8- or 16-bit).
This operation is used in the simplest or most complex image processing functions in OpenCV.
- Parameters:
-
v Function parameter.
- See also:
- add, subtract, multiply, divide, Mat::convertTo
Definition at line 82 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | schar | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 84 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | ushort | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 86 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | short | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 88 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | float | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 94 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | int64 | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 98 of file saturate.hpp.
static _Tp cv::saturate_cast | ( | unsigned | v ) | [static] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 90 of file saturate.hpp.
CV_EXPORTS bool setBreakOnError | ( | bool | flag ) |
Sets/resets the break-on-error mode.
When the break-on-error mode is set, the default error handler issues a hardware exception, which can make debugging more convenient.
- Returns:
- the previous state
Definition at line 629 of file system.cpp.
void setNumThreads | ( | int | nthreads ) |
OpenCV will try to set the number of threads for the next parallel region.
If threads == 0, OpenCV will disable threading optimizations and run all it's functions sequentially. Passing threads < 0 will reset threads number to system default. This function must be called outside of parallel region.
OpenCV will try to run it's functions with specified threads number, but some behaviour differs from framework:
- `TBB` – User-defined parallel constructions will run with the same threads number, if another does not specified. If late on user creates own scheduler, OpenCV will be use it.
- `OpenMP` – No special defined behaviour.
- `Concurrency` – If threads == 1, OpenCV will disable threading optimizations and run it's functions sequentially.
- `GCD` – Supports only values <= 0.
- `C=` – No special defined behaviour.
- Parameters:
-
nthreads Number of threads used by OpenCV.
- See also:
- getNumThreads, getThreadNum
Definition at line 380 of file parallel.cpp.
CV_EXPORTS_W void setUseOptimized | ( | bool | onoff ) |
Enables or disables the optimized code.
The function can be used to dynamically turn on and off optimized code (code that uses SSE2, AVX, and other instructions on the platforms that support it). It sets a global flag that is further checked by OpenCV functions. Since the flag is not checked in the inner OpenCV loops, it is only safe to call the function on the very top level in your application where you can be sure that no other OpenCV function is currently executed.
By default, the optimized code is enabled unless you disable it in CMake. The current status can be retrieved using useOptimized.
- Parameters:
-
onoff The boolean flag specifying whether the optimized code should be used (onoff=true) or not (onoff=false).
Definition at line 385 of file system.cpp.
CV_EXPORTS_W bool useOptimized | ( | ) |
Returns the status of optimized code usage.
The function returns true if the optimized code is enabled. Otherwise, it returns false.
Definition at line 406 of file system.cpp.
Generated on Tue Jul 12 2022 15:17:34 by
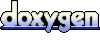