Opencv 3.1 project on GR-PEACH board
Fork of gr-peach-opencv-project by
OpenGL interoperability
[Core functionality]
This section describes OpenGL interoperability. More...
Data Structures | |
class | Buffer |
Smart pointer for OpenGL buffer object with reference counting. More... | |
class | Texture2D |
Smart pointer for OpenGL 2D texture memory with reference counting. More... | |
class | Arrays |
Wrapper for OpenGL Client-Side Vertex arrays. More... | |
Enumerations | |
enum | RenderModes |
render mode More... | |
Functions | |
Buffer () | |
The constructors. | |
Buffer (int arows, int acols, int atype, unsigned int abufId, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Buffer (Size asize, int atype, unsigned int abufId, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Buffer (int arows, int acols, int atype, Target target=ARRAY_BUFFER, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Buffer (Size asize, int atype, Target target=ARRAY_BUFFER, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Buffer (InputArray arr, Target target=ARRAY_BUFFER, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
void | create (int arows, int acols, int atype, Target target=ARRAY_BUFFER, bool autoRelease=false) |
Allocates memory for ogl::Buffer object. | |
void | create (Size asize, int atype, Target target=ARRAY_BUFFER, bool autoRelease=false) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
void | release () |
Decrements the reference counter and destroys the buffer object if needed. | |
void | setAutoRelease (bool flag) |
Sets auto release mode. | |
void | copyFrom (InputArray arr, Target target=ARRAY_BUFFER, bool autoRelease=false) |
Copies from host/device memory to OpenGL buffer. | |
void | copyFrom (InputArray arr, cuda::Stream &stream, Target target=ARRAY_BUFFER, bool autoRelease=false) |
void | copyTo (OutputArray arr) const |
Copies from OpenGL buffer to host/device memory or another OpenGL buffer object. | |
void | copyTo (OutputArray arr, cuda::Stream &stream) const |
Buffer | clone (Target target=ARRAY_BUFFER, bool autoRelease=false) const |
Creates a full copy of the buffer object and the underlying data. | |
void | bind (Target target) const |
Binds OpenGL buffer to the specified buffer binding point. | |
static void | unbind (Target target) |
Unbind any buffers from the specified binding point. | |
Mat | mapHost (Access access) |
Maps OpenGL buffer to host memory. | |
void | unmapHost () |
Unmaps OpenGL buffer. | |
cuda::GpuMat | mapDevice () |
map to device memory (blocking) | |
cuda::GpuMat | mapDevice (cuda::Stream &stream) |
Maps OpenGL buffer to CUDA device memory. | |
void | unmapDevice (cuda::Stream &stream) |
Unmaps OpenGL buffer. | |
unsigned int | bufId () const |
get OpenGL opject id | |
Texture2D () | |
The constructors. | |
Texture2D (int arows, int acols, Format aformat, unsigned int atexId, bool autoRelease=false) | |
Texture2D (Size asize, Format aformat, unsigned int atexId, bool autoRelease=false) | |
Texture2D (int arows, int acols, Format aformat, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Texture2D (Size asize, Format aformat, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
Texture2D (InputArray arr, bool autoRelease=false) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
void | create (int arows, int acols, Format aformat, bool autoRelease=false) |
Allocates memory for ogl::Texture2D object. | |
void | create (Size asize, Format aformat, bool autoRelease=false) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
void | release () |
Decrements the reference counter and destroys the texture object if needed. | |
void | setAutoRelease (bool flag) |
Sets auto release mode. | |
void | copyFrom (InputArray arr, bool autoRelease=false) |
Copies from host/device memory to OpenGL texture. | |
void | copyTo (OutputArray arr, int ddepth=CV_32F, bool autoRelease=false) const |
Copies from OpenGL texture to host/device memory or another OpenGL texture object. | |
void | bind () const |
Binds texture to current active texture unit for GL_TEXTURE_2D target. | |
unsigned int | texId () const |
get OpenGL opject id | |
void | setVertexArray (InputArray vertex) |
Sets an array of vertex coordinates. | |
void | resetVertexArray () |
Resets vertex coordinates. | |
void | setColorArray (InputArray color) |
Sets an array of vertex colors. | |
void | resetColorArray () |
Resets vertex colors. | |
void | setNormalArray (InputArray normal) |
Sets an array of vertex normals. | |
void | resetNormalArray () |
Resets vertex normals. | |
void | setTexCoordArray (InputArray texCoord) |
Sets an array of vertex texture coordinates. | |
void | resetTexCoordArray () |
Resets vertex texture coordinates. | |
void | release () |
Releases all inner buffers. | |
void | setAutoRelease (bool flag) |
Sets auto release mode all inner buffers. | |
void | bind () const |
Binds all vertex arrays. | |
int | size () const |
Returns the vertex count. | |
CV_EXPORTS void | render (const Texture2D &tex, Rect_< double > wndRect=Rect_< double >(0.0, 0.0, 1.0, 1.0), Rect_< double > texRect=Rect_< double >(0.0, 0.0, 1.0, 1.0)) |
Render OpenGL texture or primitives. | |
CV_EXPORTS void | render (const Arrays &arr, int mode=POINTS, Scalar color=Scalar::all(255)) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_EXPORTS void | render (const Arrays &arr, InputArray indices, int mode=POINTS, Scalar color=Scalar::all(255)) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_EXPORTS Context & | initializeContextFromGL () |
Creates OpenCL context from GL. | |
CV_EXPORTS void | convertToGLTexture2D (InputArray src, Texture2D &texture) |
Converts InputArray to Texture2D object. | |
CV_EXPORTS void | convertFromGLTexture2D (const Texture2D &texture, OutputArray dst) |
Converts Texture2D object to OutputArray. | |
CV_EXPORTS UMat | mapGLBuffer (const Buffer &buffer, int accessFlags=ACCESS_READ|ACCESS_WRITE) |
Maps Buffer object to process on CL side (convert to UMat). | |
CV_EXPORTS void | unmapGLBuffer (UMat &u) |
Unmaps Buffer object (releases UMat, previously mapped from Buffer). | |
CV_EXPORTS void | setGlDevice (int device=0) |
Sets a CUDA device and initializes it for the current thread with OpenGL interoperability. |
Detailed Description
This section describes OpenGL interoperability.
To enable OpenGL support, configure OpenCV using CMake with WITH_OPENGL=ON . Currently OpenGL is supported only with WIN32, GTK and Qt backends on Windows and Linux (MacOS and Android are not supported). For GTK backend gtkglext-1.0 library is required.
To use OpenGL functionality you should first create OpenGL context (window or frame buffer). You can do this with namedWindow function or with other OpenGL toolkit (GLUT, for example).
Enumeration Type Documentation
enum RenderModes |
render mode
Definition at line 478 of file opengl.hpp.
Function Documentation
void bind | ( | Target | target ) | const [inherited] |
Binds OpenGL buffer to the specified buffer binding point.
- Parameters:
-
target Binding point. See cv::ogl::Buffer::Target .
Definition at line 719 of file opengl.cpp.
void bind | ( | ) | const [inherited] |
Binds texture to current active texture unit for GL_TEXTURE_2D target.
Definition at line 1217 of file opengl.cpp.
void bind | ( | ) | const [inherited] |
Binds all vertex arrays.
Definition at line 1333 of file opengl.cpp.
Buffer | ( | int | arows, |
int | acols, | ||
int | atype, | ||
unsigned int | abufId, | ||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arows Number of rows in a 2D array. acols Number of columns in a 2D array. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. abufId Buffer object name. autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 468 of file opengl.cpp.
Buffer | ( | int | arows, |
int | acols, | ||
int | atype, | ||
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arows Number of rows in a 2D array. acols Number of columns in a 2D array. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
Buffer | ( | ) | [inherited] |
The constructors.
Creates empty ogl::Buffer object, creates ogl::Buffer object from existed buffer ( abufId parameter), allocates memory for ogl::Buffer object or copies from host/device memory.
Definition at line 459 of file opengl.cpp.
Buffer | ( | Size | asize, |
int | atype, | ||
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
asize 2D array size. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
Buffer | ( | InputArray | arr, |
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [explicit, inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Input array (host or device memory, it can be Mat , cuda::GpuMat or std::vector ). target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 501 of file opengl.cpp.
Buffer | ( | Size | asize, |
int | atype, | ||
unsigned int | abufId, | ||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
asize 2D array size. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. abufId Buffer object name. autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 485 of file opengl.cpp.
unsigned int bufId | ( | ) | const [inherited] |
get OpenGL opject id
Definition at line 820 of file opengl.cpp.
cv::ogl::Buffer clone | ( | Target | target = ARRAY_BUFFER , |
bool | autoRelease = false |
||
) | const [inherited] |
Creates a full copy of the buffer object and the underlying data.
- Parameters:
-
target Buffer usage for destination buffer. autoRelease Auto release mode for destination buffer.
Definition at line 705 of file opengl.cpp.
void convertFromGLTexture2D | ( | const Texture2D & | texture, |
OutputArray | dst | ||
) |
Converts Texture2D object to OutputArray.
- Parameters:
-
texture - source Texture2D object. dst - destination OutputArray.
Definition at line 1749 of file opengl.cpp.
void convertToGLTexture2D | ( | InputArray | src, |
Texture2D & | texture | ||
) |
Converts InputArray to Texture2D object.
- Parameters:
-
src - source InputArray. texture - destination Texture2D object.
Definition at line 1697 of file opengl.cpp.
void copyFrom | ( | InputArray | arr, |
bool | autoRelease = false |
||
) | [inherited] |
Copies from host/device memory to OpenGL texture.
- Parameters:
-
arr Input array (host or device memory, it can be Mat , cuda::GpuMat or ogl::Buffer ). autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 1097 of file opengl.cpp.
void copyFrom | ( | InputArray | arr, |
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
Copies from host/device memory to OpenGL buffer.
- Parameters:
-
arr Input array (host or device memory, it can be Mat , cuda::GpuMat or std::vector ). target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 576 of file opengl.cpp.
void copyFrom | ( | InputArray | arr, |
cuda::Stream & | stream, | ||
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 621 of file opengl.cpp.
void copyTo | ( | OutputArray | arr, |
int | ddepth = CV_32F , |
||
bool | autoRelease = false |
||
) | const [inherited] |
Copies from OpenGL texture to host/device memory or another OpenGL texture object.
- Parameters:
-
arr Destination array (host or device memory, can be Mat , cuda::GpuMat , ogl::Buffer or ogl::Texture2D ). ddepth Destination depth. autoRelease Auto release mode for destination buffer (if arr is OpenGL buffer or texture).
Definition at line 1164 of file opengl.cpp.
void copyTo | ( | OutputArray | arr ) | const [inherited] |
Copies from OpenGL buffer to host/device memory or another OpenGL buffer object.
- Parameters:
-
arr Destination array (host or device memory, can be Mat , cuda::GpuMat , std::vector or ogl::Buffer ).
Definition at line 646 of file opengl.cpp.
void copyTo | ( | OutputArray | arr, |
cuda::Stream & | stream | ||
) | const [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 686 of file opengl.cpp.
void create | ( | Size | asize, |
int | atype, | ||
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
asize 2D array size. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
void create | ( | int | arows, |
int | acols, | ||
Format | aformat, | ||
bool | autoRelease = false |
||
) | [inherited] |
Allocates memory for ogl::Texture2D object.
- Parameters:
-
arows Number of rows. acols Number of columns. aformat Image format. See cv::ogl::Texture2D::Format . autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 1055 of file opengl.cpp.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
asize 2D array size. aformat Image format. See cv::ogl::Texture2D::Format . autoRelease Auto release mode (if true, release will be called in object's destructor).
void create | ( | int | arows, |
int | acols, | ||
int | atype, | ||
Target | target = ARRAY_BUFFER , |
||
bool | autoRelease = false |
||
) | [inherited] |
Allocates memory for ogl::Buffer object.
- Parameters:
-
arows Number of rows in a 2D array. acols Number of columns in a 2D array. atype Array type ( CV_8UC1, ..., CV_64FC4 ). See Mat for details. target Buffer usage. See cv::ogl::Buffer::Target . autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 533 of file opengl.cpp.
Context & initializeContextFromGL | ( | ) |
Creates OpenCL context from GL.
- Returns:
- Returns reference to OpenCL Context
Definition at line 1599 of file opengl.cpp.
GpuMat mapDevice | ( | ) | [inherited] |
map to device memory (blocking)
Definition at line 760 of file opengl.cpp.
cuda::GpuMat mapDevice | ( | cuda::Stream & | stream ) | [inherited] |
Maps OpenGL buffer to CUDA device memory.
This operatation doesn't copy data. Several buffer objects can be mapped to CUDA memory at a time.
A mapped data store must be unmapped with ogl::Buffer::unmapDevice before its buffer object is used.
Definition at line 788 of file opengl.cpp.
UMat mapGLBuffer | ( | const Buffer & | buffer, |
int | accessFlags = ACCESS_READ|ACCESS_WRITE |
||
) |
Maps Buffer object to process on CL side (convert to UMat).
Function creates CL buffer from GL one, and then constructs UMat that can be used to process buffer data with OpenCV functions. Note that in current implementation UMat constructed this way doesn't own corresponding GL buffer object, so it is the user responsibility to close down CL/GL buffers relationships by explicitly calling unmapGLBuffer() function.
- Parameters:
-
buffer - source Buffer object. accessFlags - data access flags (ACCESS_READ|ACCESS_WRITE).
- Returns:
- Returns UMat object
Definition at line 1808 of file opengl.cpp.
Mat mapHost | ( | Access | access ) | [inherited] |
Maps OpenGL buffer to host memory.
mapHost maps to the client's address space the entire data store of the buffer object. The data can then be directly read and/or written relative to the returned pointer, depending on the specified access policy.
A mapped data store must be unmapped with ogl::Buffer::unmapHost before its buffer object is used.
This operation can lead to memory transfers between host and device.
Only one buffer object can be mapped at a time.
- Parameters:
-
access Access policy, indicating whether it will be possible to read from, write to, or both read from and write to the buffer object's mapped data store. The symbolic constant must be ogl::Buffer::READ_ONLY , ogl::Buffer::WRITE_ONLY or ogl::Buffer::READ_WRITE .
Definition at line 740 of file opengl.cpp.
void release | ( | void | ) | [inherited] |
Decrements the reference counter and destroys the buffer object if needed.
The function will call setAutoRelease(true) .
Definition at line 554 of file opengl.cpp.
void release | ( | void | ) | [inherited] |
Decrements the reference counter and destroys the texture object if needed.
The function will call setAutoRelease(true) .
Definition at line 1075 of file opengl.cpp.
void release | ( | void | ) | [inherited] |
Releases all inner buffers.
Definition at line 1317 of file opengl.cpp.
void render | ( | const Arrays & | arr, |
int | mode = POINTS , |
||
Scalar | color = Scalar::all(255) |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Array of privitives vertices. mode Render mode. One of cv::ogl::RenderModes color Color for all vertices. Will be used if arr doesn't contain color array.
Definition at line 1487 of file opengl.cpp.
void render | ( | const Texture2D & | tex, |
Rect_< double > | wndRect = Rect_<double>(0.0, 0.0, 1.0, 1.0) , |
||
Rect_< double > | texRect = Rect_<double>(0.0, 0.0, 1.0, 1.0) |
||
) |
Render OpenGL texture or primitives.
- Parameters:
-
tex Texture to draw. wndRect Region of window, where to draw a texture (normalized coordinates). texRect Region of texture to draw (normalized coordinates).
Definition at line 1415 of file opengl.cpp.
void render | ( | const Arrays & | arr, |
InputArray | indices, | ||
int | mode = POINTS , |
||
Scalar | color = Scalar::all(255) |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Array of privitives vertices. indices Array of vertices indices (host or device memory). mode Render mode. One of cv::ogl::RenderModes color Color for all vertices. Will be used if arr doesn't contain color array.
Definition at line 1506 of file opengl.cpp.
void resetColorArray | ( | ) | [inherited] |
Resets vertex colors.
Definition at line 1274 of file opengl.cpp.
void resetNormalArray | ( | ) | [inherited] |
Resets vertex normals.
Definition at line 1293 of file opengl.cpp.
void resetTexCoordArray | ( | ) | [inherited] |
Resets vertex texture coordinates.
Definition at line 1312 of file opengl.cpp.
void resetVertexArray | ( | ) | [inherited] |
Resets vertex coordinates.
Definition at line 1256 of file opengl.cpp.
void setAutoRelease | ( | bool | flag ) | [inherited] |
Sets auto release mode.
The lifetime of the OpenGL object is tied to the lifetime of the context. If OpenGL context was bound to a window it could be released at any time (user can close a window). If object's destructor is called after destruction of the context it will cause an error. Thus ogl::Buffer doesn't destroy OpenGL object in destructor by default (all OpenGL resources will be released with OpenGL context). This function can force ogl::Buffer destructor to destroy OpenGL object.
- Parameters:
-
flag Auto release mode (if true, release will be called in object's destructor).
Definition at line 566 of file opengl.cpp.
void setAutoRelease | ( | bool | flag ) | [inherited] |
Sets auto release mode.
- Parameters:
-
flag Auto release mode (if true, release will be called in object's destructor).
The lifetime of the OpenGL object is tied to the lifetime of the context. If OpenGL context was bound to a window it could be released at any time (user can close a window). If object's destructor is called after destruction of the context it will cause an error. Thus ogl::Texture2D doesn't destroy OpenGL object in destructor by default (all OpenGL resources will be released with OpenGL context). This function can force ogl::Texture2D destructor to destroy OpenGL object.
Definition at line 1087 of file opengl.cpp.
void setAutoRelease | ( | bool | flag ) | [inherited] |
Sets auto release mode all inner buffers.
- Parameters:
-
flag Auto release mode.
Definition at line 1325 of file opengl.cpp.
void setColorArray | ( | InputArray | color ) | [inherited] |
Sets an array of vertex colors.
- Parameters:
-
color array with vertex colors, can be both host and device memory.
Definition at line 1262 of file opengl.cpp.
void setGlDevice | ( | int | device = 0 ) |
Sets a CUDA device and initializes it for the current thread with OpenGL interoperability.
This function should be explicitly called after OpenGL context creation and before any CUDA calls.
- Parameters:
-
device System index of a CUDA device starting with 0.
Definition at line 116 of file opengl.cpp.
void setNormalArray | ( | InputArray | normal ) | [inherited] |
Sets an array of vertex normals.
- Parameters:
-
normal array with vertex normals, can be both host and device memory.
Definition at line 1279 of file opengl.cpp.
void setTexCoordArray | ( | InputArray | texCoord ) | [inherited] |
Sets an array of vertex texture coordinates.
- Parameters:
-
texCoord array with vertex texture coordinates, can be both host and device memory.
Definition at line 1298 of file opengl.cpp.
void setVertexArray | ( | InputArray | vertex ) | [inherited] |
Sets an array of vertex coordinates.
- Parameters:
-
vertex array with vertex coordinates, can be both host and device memory.
Definition at line 1240 of file opengl.cpp.
int size | ( | ) | const [inherited] |
Returns the vertex count.
unsigned int texId | ( | ) | const [inherited] |
get OpenGL opject id
Definition at line 1226 of file opengl.cpp.
Texture2D | ( | int | arows, |
int | acols, | ||
Format | aformat, | ||
unsigned int | atexId, | ||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 952 of file opengl.cpp.
Texture2D | ( | ) | [inherited] |
The constructors.
Creates empty ogl::Texture2D object, allocates memory for ogl::Texture2D object or copies from host/device memory.
Definition at line 943 of file opengl.cpp.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 969 of file opengl.cpp.
Texture2D | ( | int | arows, |
int | acols, | ||
Format | aformat, | ||
bool | autoRelease = false |
||
) | [inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arows Number of rows. acols Number of columns. aformat Image format. See cv::ogl::Texture2D::Format . autoRelease Auto release mode (if true, release will be called in object's destructor).
Texture2D | ( | InputArray | arr, |
bool | autoRelease = false |
||
) | [explicit, inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Input array (host or device memory, it can be Mat , cuda::GpuMat or ogl::Buffer ). autoRelease Auto release mode (if true, release will be called in object's destructor).
Definition at line 985 of file opengl.cpp.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
asize 2D array size. aformat Image format. See cv::ogl::Texture2D::Format . autoRelease Auto release mode (if true, release will be called in object's destructor).
void unbind | ( | Target | target ) | [static, inherited] |
Unbind any buffers from the specified binding point.
- Parameters:
-
target Binding point. See cv::ogl::Buffer::Target .
Definition at line 729 of file opengl.cpp.
void unmapDevice | ( | cuda::Stream & | stream ) | [inherited] |
Unmaps OpenGL buffer.
Definition at line 805 of file opengl.cpp.
void unmapGLBuffer | ( | UMat & | u ) |
Unmaps Buffer object (releases UMat, previously mapped from Buffer).
Function must be called explicitly by the user for each UMat previously constructed by the call to mapGLBuffer() function.
- Parameters:
-
u - source UMat, created by mapGLBuffer().
Definition at line 1858 of file opengl.cpp.
void unmapHost | ( | ) | [inherited] |
Unmaps OpenGL buffer.
Definition at line 751 of file opengl.cpp.
Generated on Tue Jul 12 2022 15:17:34 by
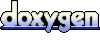