
car_test
Dependencies: APDS_9960 AutomationElements ESP8266 HALLFX_ENCODER HCSR04 MotorDriver PID
Fork of car_test by
main.cpp
00001 #include "mbed.h" 00002 00003 #include "HALLFX_ENCODER.h" 00004 #include "MotorDriver.h" 00005 00006 #include "HCSR04.h" 00007 #include "AutomationElements.h" 00008 00009 #include "glibr.h" 00010 00011 Thread thread; 00012 DigitalOut led1(LED1); 00013 volatile bool running = true; 00014 00015 //----------------------motor 00016 MotorDriver mtrR(PA_0, PA_4, PA_1, 10000.0, true); // in1, in2, pwm, pwmFreq, isBrakeable 00017 MotorDriver mtrL(PC_1, PC_0, PB_0, 10000.0, true); // in1, in2, pwm, pwmFreq, isBrakeable 00018 HALLFX_ENCODER encR(PB_3); 00019 HALLFX_ENCODER encL(PB_5); 00020 00021 //------------------------hc-sr04 00022 Serial pc(USBTX, USBRX); 00023 HCSR04 sensor(PA_6, PA_7); 00024 float sampleTime = 0.1; 00025 PT1 filter(1, 2, sampleTime); 00026 Timer timer; 00027 float distance; 00028 float filteredDistance; 00029 00030 //----------------------------GSensor APDS-9960 00031 glibr GSensor(PB_9,PB_8);//i2c 00032 int ge_diff;//gesture differentiate 00033 00034 Thread thread_sonar; 00035 Thread thread_motor; 00036 Thread thread_gsensor; 00037 00038 int txlength_sonar; 00039 int txlength_motor; 00040 int txlength_gsensor; 00041 00042 char txbuf_sonar[256]; 00043 char txbuf_motor[256]; 00044 char txbuf_gsensor[256]; 00045 00046 Thread thread_pc_send; 00047 void pc_send_Thread(){ 00048 while(true) 00049 { 00050 if(txlength_sonar>0) 00051 { 00052 puts(txbuf_sonar); 00053 memset(&txbuf_sonar,0,256); 00054 txlength_sonar=0; 00055 } 00056 else if(txlength_motor>0) 00057 { 00058 puts(txbuf_motor); 00059 memset(&txbuf_motor,0,256); 00060 txlength_motor=0; 00061 } 00062 else if(txlength_gsensor>0) 00063 { 00064 puts(txbuf_gsensor); 00065 memset(&txbuf_gsensor,0,256); 00066 txlength_gsensor=0; 00067 led1=!led1; 00068 } 00069 //wait_ms(50); 00070 } 00071 } 00072 //void motorThread(void const *argument){ 00073 void motorThread(){ 00074 pc.printf("motor : start.....\r\n"); 00075 encL.reset(); 00076 encR.reset(); 00077 while(true) 00078 { 00079 switch ( ge_diff ) 00080 { // gesture differentiate 00081 case DIR_UP: 00082 mtrL.forceSetSpeed(0.1); 00083 mtrR.forceSetSpeed(0.1); 00084 break; 00085 case DIR_DOWN: 00086 mtrL.forceSetSpeed(-0.1); 00087 mtrR.forceSetSpeed(-0.1); 00088 break; 00089 case DIR_LEFT: 00090 mtrL.forceSetSpeed(-0.1); 00091 mtrR.forceSetSpeed(0.1); 00092 break; 00093 case DIR_RIGHT: 00094 mtrL.forceSetSpeed(0.1); 00095 mtrR.forceSetSpeed(-0.1); 00096 break; 00097 case DIR_NEAR: 00098 mtrL.forceSetSpeed(0); 00099 mtrR.forceSetSpeed(0); 00100 break; 00101 case DIR_FAR: 00102 mtrL.forceSetSpeed(0.1); 00103 mtrR.forceSetSpeed(0.1); 00104 break; 00105 default: 00106 if(filteredDistance>2 && filteredDistance <=25) 00107 { 00108 mtrL.forceSetSpeed(-0.1); 00109 mtrR.forceSetSpeed(0.1); 00110 } 00111 else if( filteredDistance>2 && filteredDistance <400) 00112 { 00113 mtrL.forceSetSpeed(0.1); 00114 mtrR.forceSetSpeed(0.1); 00115 } 00116 else 00117 { 00118 mtrL.forceSetSpeed(0); 00119 mtrR.forceSetSpeed(0); 00120 } 00121 break; 00122 } 00123 00124 /* 00125 // Set motor speed in open loop mode 00126 // Motor direction based on working setpoint var 00127 int dirL, dirR; 00128 if(working_setpoint < 0.0){ 00129 dirL = -1; dirR = -1; 00130 } 00131 else{ 00132 dirL = 1; dirR = 1; 00133 } 00134 float speed = abs(working_setpoint) / 90.0; // Normalize based on 90 RPM 00135 mtrL.forceSetSpeed(speed * dirL); 00136 mtrR.forceSetSpeed(speed * dirR); 00137 */ 00138 wait(0.1); 00139 if(ge_diff!=DIR_NEAR)ge_diff=DIR_NONE; 00140 } 00141 } 00142 00143 //void sonarThread(void const *argument){ 00144 void sonarThread(){ 00145 //int t_ms=0; 00146 sensor.setRanges(2, 400); 00147 pc.printf("Minimum sensor range = %g cm\n\rMaximum sensor range = %g cm\n\r", sensor.getMinRange(), sensor.getMaxRange()); 00148 pc.printf("Sensor: Filtered:\n\r"); 00149 while(true) { 00150 led1=1; 00151 timer.reset(); 00152 timer.start(); 00153 sensor.startMeasurement(); 00154 00155 /* 00156 while(!sensor.isNewDataReady()) { 00157 // waiting time depends on the distance 00158 // wait for new data 00159 } 00160 */ 00161 wait_ms(50); 00162 00163 distance = sensor.getDistance_cm(); 00164 filter.in(distance); 00165 filteredDistance = filter.out(); 00166 //pc.printf("%7.1f cm %7.1f cm\n\r", distance, filteredDistance); 00167 sprintf(txbuf_sonar,"sensor : %7.1f cm %7.1f cm\n\r", distance, filteredDistance); 00168 txlength_sonar=8; 00169 //timer.stop(); 00170 //t_ms=timer.read_ms(); 00171 //if(t_ms<100)wait_ms(sampleTime * 1000 - timer.read_ms()); // time the loop 00172 led1=0; 00173 } 00174 } 00175 00176 //void gsensorThread(void const *argument){ 00177 void gsensorThread(){ 00178 // Initialize Sensor with I2C 00179 if ( GSensor.ginit() ) { 00180 pc.printf("APDS-9960 initialization complete\r\n"); 00181 } else { 00182 pc.printf("Something went wrong during APDS-9960 init\r\n"); 00183 } 00184 00185 // Start running the APDS-9960 gesture sensor engine 00186 if ( GSensor.enableGestureSensor(true) ) { 00187 pc.printf("Gesture sensor is now running\r\n"); 00188 } else { 00189 pc.printf("Something went wrong during gesture sensor init!\r\n"); 00190 } 00191 00192 while(1) { 00193 if ( GSensor.isGestureAvailable() ) { // gesture detect 00194 00195 switch ( GSensor.readGesture() ) { // gesture differentiate 00196 case DIR_UP: 00197 ge_diff=DIR_UP; 00198 //pc.printf("UP\r\n"); 00199 sprintf(txbuf_gsensor,"GSensor : UP\r\n"); 00200 txlength_gsensor=16; 00201 break; 00202 case DIR_DOWN: 00203 ge_diff=DIR_DOWN; 00204 //pc.printf("DOWN\r\n"); 00205 sprintf(txbuf_gsensor,"GSensor : DOWN\r\n"); 00206 txlength_gsensor=18; 00207 break; 00208 case DIR_LEFT: 00209 ge_diff=DIR_LEFT; 00210 //pc.printf("LEFT\r\n"); 00211 sprintf(txbuf_gsensor,"GSensor : LEFT\r\n"); 00212 txlength_gsensor=18; 00213 break; 00214 case DIR_RIGHT: 00215 ge_diff=DIR_RIGHT; 00216 //pc.printf("RIGHT\r\n"); 00217 sprintf(txbuf_gsensor,"GSensor : RIGHT\r\n"); 00218 txlength_gsensor=19; 00219 break; 00220 case DIR_NEAR: 00221 ge_diff=DIR_NEAR; 00222 //pc.printf("NEAR\r\n"); 00223 sprintf(txbuf_gsensor,"GSensor : NEAR\r\n"); 00224 txlength_gsensor=18; 00225 break; 00226 case DIR_FAR: 00227 ge_diff=DIR_FAR; 00228 //pc.printf("FAR\r\n"); 00229 sprintf(txbuf_gsensor,"GSensor : FAR\r\n"); 00230 txlength_gsensor=17; 00231 break; 00232 default: 00233 //ge_diff=DIR_NONE; 00234 //pc.printf("NONE\r\n"); 00235 break; 00236 } 00237 } 00238 } 00239 } 00240 // Blink function toggles the led in a long running loop 00241 void blink(DigitalOut *led) { 00242 while (running) { 00243 *led = !*led; 00244 wait(1); 00245 } 00246 } 00247 void callback_ex() { 00248 // Note: you need to actually read from the serial to clear the RX interrupt 00249 int tmp=pc.getc(); 00250 //printf("%c\n", tmp); 00251 } 00252 // Spawns a thread to run blink for 5 seconds 00253 int main() { 00254 pc.baud(9600); 00255 pc.attach(&callback_ex); 00256 thread_pc_send.start(callback(pc_send_Thread)); 00257 00258 thread.start(callback(blink, &led1)); 00259 00260 wait(5); 00261 running = false; 00262 thread.join(); 00263 00264 thread_gsensor.start(callback(gsensorThread)); 00265 wait(1); 00266 thread_motor.start(callback(motorThread)); 00267 wait(1); 00268 //thread_sonar.start(callback(sonarThread)); 00269 00270 //Thread thread_sonar(sonarThread); 00271 } 00272 00273 /* 00274 主程序指定中断函数 00275 flipper.attach(&flip, 2.0); // the address of the function to be attached (flip) and the interval (2 seconds) 00276 00277 主程序指定 类 的 方法 00278 t.attach(callback(&f, &Flipper::flip), 2.0); // the address of the object, member function, and interval 00279 00280 00281 1、线程 00282 需加载mbed-os 00283 00284 #include "mbed.h" 00285 00286 Thread thread; 00287 DigitalOut led1(LED1); 00288 volatile bool running = true; 00289 00290 // Blink function toggles the led in a long running loop 00291 void blink(DigitalOut *led) { 00292 while (running) { 00293 *led = !*led; 00294 wait(1); 00295 } 00296 } 00297 00298 // Spawns a thread to run blink for 5 seconds 00299 int main() { 00300 thread.start(callback(blink, &led1)); 00301 wait(5); 00302 running = false; 00303 thread.join(); 00304 } 00305 00306 00307 2、休眠+按键中断唤醒 00308 需更新mbed 00309 使用F401板时,IDD电流测试,运行时:18mA,灯亮时21 .2mA,睡眠时:6.26 mA,深度睡眠时:0.68 mA, 00310 00311 RTC唤醒使用第三方类(未测试) 00312 00313 #include "mbed.h" 00314 00315 InterruptIn event(USER_BUTTON); 00316 DigitalOut myled(LED1); 00317 00318 int go_to_sleep = 0; 00319 00320 void pressed() 00321 { 00322 printf("Button pressed\n"); 00323 go_to_sleep = go_to_sleep + 1; 00324 if (go_to_sleep > 3) go_to_sleep = 0; 00325 00326 } 00327 00328 int main() 00329 { 00330 int i = 0; 00331 00332 printf("\nPress Button to enter/exit sleep & deepsleep\n"); 00333 00334 event.fall(&pressed); 00335 00336 while (1) { 00337 00338 if ((go_to_sleep == 0) || (go_to_sleep == 2)) { 00339 printf("%d: Running\n", i); 00340 myled = !myled; 00341 wait(1.0); 00342 } 00343 00344 if (go_to_sleep == 1) { 00345 myled = 0; 00346 printf("%d: Entering sleep (press user button to resume)\n", i); 00347 sleep(); 00348 } 00349 00350 if (go_to_sleep == 3) { 00351 myled = 0; 00352 printf("%d: Entering deepsleep (press user button to resume)\n", i); 00353 deepsleep(); 00354 } 00355 00356 i++; 00357 } 00358 } 00359 00360 00361 3、系统定时器中断 00362 #include "mbed.h" 00363 00364 Ticker toggle_led_ticker; 00365 00366 DigitalOut led1(LED1); 00367 00368 void toggle_led() { 00369 led1 = !led1; 00370 } 00371 00372 int main() { 00373 // Init the ticker with the address of the function (toggle_led) to be attached and the interval (100 ms) 00374 toggle_led_ticker.attach(&toggle_led, 0.1); 00375 while (true) { 00376 // Do other things... 00377 } 00378 } 00379 00380 4、超时定时器中断 00381 #include "mbed.h" 00382 00383 // A class for flip()-ing a DigitalOut 00384 class Flipper { 00385 public: 00386 Flipper(PinName pin) : _pin(pin) { 00387 _pin = 0; 00388 } 00389 void flip() { 00390 _pin = !_pin; 00391 } 00392 private: 00393 DigitalOut _pin; 00394 }; 00395 00396 DigitalOut led1(LED1); 00397 Flipper f(LED2); 00398 Timeout t; 00399 00400 int main() { 00401 // the address of the object, member function, and interval 00402 t.attach(callback(&f, &Flipper::flip), 2.0); 00403 00404 // spin in a main loop. flipper will interrupt it to call flip 00405 while(1) { 00406 led1 = !led1; 00407 wait(0.2); 00408 } 00409 } 00410 00411 00412 5、通用定时器中断 00413 00414 // Count the time to toggle a LED 00415 00416 #include "mbed.h" 00417 00418 Timer timer; 00419 DigitalOut led(LED1); 00420 int begin, end; 00421 00422 int main() { 00423 timer.start(); 00424 begin = timer.read_us(); 00425 led = !led; 00426 end = timer.read_us(); 00427 printf("Toggle the led takes %d us", end - begin); 00428 } 00429 00430 6、外部中断附加事件 00431 需加载mbed-os 00432 00433 #include "mbed.h" 00434 #include "mbed_events.h" 00435 00436 DigitalOut led1(LED1); 00437 InterruptIn sw(SW2); 00438 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00439 Thread t; 00440 00441 void rise_handler(void) { 00442 // Toggle LED 00443 led1 = !led1; 00444 } 00445 00446 void fall_handler(void) { 00447 printf("fall_handler in context %p\r\n", Thread::gettid()); 00448 // Toggle LED 00449 led1 = !led1; 00450 } 00451 00452 int main() { 00453 // Start the event queue 00454 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00455 printf("Starting in context %p\r\n", Thread::gettid()); 00456 // The 'rise' handler will execute in IRQ context 00457 sw.rise(rise_handler); 00458 // The 'fall' handler will execute in the context of thread 't' 00459 sw.fall(queue.event(fall_handler)); 00460 } 00461 00462 00463 7、线程间信号量的传递 00464 需加载mbed-os 00465 00466 #include "mbed.h" 00467 00468 Thread thread; 00469 DigitalOut led(LED1); 00470 00471 void led_thread() { 00472 while (true) { 00473 // Signal flags that are reported as event are automatically cleared. 00474 Thread::signal_wait(0x1); 00475 led = !led; 00476 } 00477 } 00478 00479 int main (void) { 00480 thread.start(callback(led_thread)); 00481 00482 while (true) { 00483 wait(1); 00484 thread.signal_set(0x1); 00485 } 00486 } 00487 00488 */
Generated on Wed Jul 20 2022 02:01:11 by
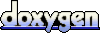