Code for an autonomous plane I'm building. Includes process scheduling, process communication, and hardware sensor interfacing (via I2C). NOTE: currently in development, source code will be updated frequently.
control.h
00001 #ifndef CONTROL_H 00002 #define CONTROL_H 00003 00004 #define OUTPUT_SIZE 512 00005 #define MAX_SID_LEN 32 00006 00007 char* get_output(int); 00008 void init(); 00009 void schedule(); 00010 int schedule_proc(char *sid, int (*funcptr)(int)); 00011 00012 typedef enum 00013 { 00014 READY, 00015 BLOCKED, 00016 ZOMBIE, 00017 EMPTY 00018 } proc_stat; 00019 00020 typedef struct 00021 { 00022 // process status 00023 unsigned int status; 00024 // if status == BLOCKED, wait till block_pid's status is ZOMBIE 00025 unsigned int block_pid; 00026 // function. each "process" is a function with a number of properties and storage space 00027 int (*start)(int); 00028 char sid[MAX_SID_LEN]; 00029 char output[OUTPUT_SIZE]; 00030 } process; 00031 00032 #endif //CONTROL_H
Generated on Tue Jul 12 2022 21:39:02 by
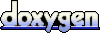