Code for an autonomous plane I'm building. Includes process scheduling, process communication, and hardware sensor interfacing (via I2C). NOTE: currently in development, source code will be updated frequently.
control.cpp
00001 #include "control.h" 00002 #include "sensor.h" 00003 #include "steps.h" 00004 #include "mbed.h" 00005 00006 #define MAXPROC 15 00007 00008 process procs[MAXPROC] = {0}; 00009 00010 int main() 00011 { 00012 init(); 00013 while (true) 00014 { 00015 schedule(); 00016 } 00017 } 00018 00019 void init() 00020 { 00021 // initialize i2c sensors 00022 schedule_proc("INITSENSE", &init_sensors); 00023 // set initial processes 00024 procs[0].status = READY; 00025 procs[0].start = &get_sensor_data; 00026 return; 00027 } 00028 00029 char* get_output(int pid) 00030 { 00031 return procs[pid].output; 00032 } 00033 00034 void schedule() 00035 { 00036 for (int i=0; i<MAXPROC; i++) 00037 { 00038 process proc = procs[i]; 00039 if(proc.status == READY) 00040 { 00041 int ret = proc.start(i); 00042 // if the process returns 0, it means don't run again 00043 if (ret == 0) 00044 { 00045 // set proc.status to ZOMBIE 00046 // ZOMBIE means process 00047 // is no longer active, but 00048 // it's output buffer is still 00049 // needed. 00050 proc.status = ZOMBIE; 00051 } 00052 return; 00053 } 00054 } 00055 } 00056 00057 int schedule_proc(char *sid, int (*funcptr)(int)) 00058 { 00059 for (int i=0; i<MAXPROC; i++) 00060 { 00061 process proc = procs[i]; 00062 if(proc.status == EMPTY) 00063 { 00064 proc.status = READY; 00065 proc.start = funcptr; 00066 strncpy(proc.sid, sid, MAX_SID_LEN-1); 00067 // null terminate proc.sid 00068 proc.sid[MAX_SID_LEN-1] = 0; 00069 return i; 00070 } 00071 } 00072 // if no open processes, return -1 00073 return -1; 00074 }
Generated on Tue Jul 12 2022 21:39:02 by
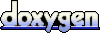