
This car parking system uses Freedom board KL25, ultrasonic sensor, keypad, and LCD. The distance is measured via ultrasonic sensor, and it is displayed on LCD. The keypad takes user input of the that decides what led to turn on. If user types 25, after 25 cm, the light of the Freedom board turns to a specific color. After half if this distance 12.5 cm, it starts to to turn yellow. And after 90% of distance is covered then it starts blinking.
Dependencies: HC_SR04_Ultrasonic_Library Keypad TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 #include "Keypad.h" 00004 #include "ultrasonic.h" 00005 00006 TextLCD my_lcd(PTE20, PTE21, PTE22, PTE23, PTE29, PTE30, TextLCD::LCD16x2); 00007 Keypad kpad(PTA12, PTD4, PTA2, PTA1, PTC9, PTC8, PTA5, PTA4); 00008 00009 void dist(int distance){ 00010 } 00011 00012 ultrasonic mu(PTA13, PTD5, .1, 1, &dist); //Set the trigger pin to PTA13 and the echo pin to PTD5 00013 //have updates every .1 seconds and a timeout after 1 00014 //second, and call dist when the distance changes 00015 float dispDist; 00016 float prevDist; 00017 float interDist; 00018 float input; 00019 00020 PwmOut ledRed(LED_RED); 00021 PwmOut ledGreen(LED_GREEN); 00022 PwmOut ledBlue(LED_BLUE); 00023 00024 int main() 00025 { 00026 my_lcd.printf("Input distance (cm) then press #:"); 00027 wait(2); 00028 my_lcd.cls(); 00029 char key; 00030 int released = 1; 00031 char inputArray[16]; 00032 int i = 0 ; 00033 while(i<16){ 00034 key = kpad.ReadKey(); //read the current key pressed 00035 00036 if(key == '\0') 00037 released = 1; //set the flag when all keys are released 00038 00039 if((key != '\0') && (released == 1)) { //if a key is pressed AND previous key was released 00040 00041 if (key == '#'){ 00042 my_lcd.printf("="); 00043 break; 00044 } 00045 else{ 00046 my_lcd.printf("%c", key); 00047 } 00048 00049 inputArray[i] = key; 00050 i++; 00051 released = 0; //clear the flag to indicate that key is still pressed 00052 } 00053 } 00054 sscanf(inputArray, "%f", &input); 00055 my_lcd.locate(0, 1); 00056 my_lcd.printf("Input: %.1f", input); 00057 wait(1); 00058 my_lcd.cls(); 00059 00060 mu.startUpdates();//start mesuring the distance 00061 while(1) 00062 { 00063 dispDist = mu.getCurrentDistance(); 00064 dispDist = dispDist / 10; 00065 interDist = ((2 * input) / 3 - input / 3) / 5; 00066 if (dispDist > input){ 00067 PwmOut extRedLed(PTD2); 00068 PwmOut extGreenLed(PTD0); 00069 extRedLed = 0; 00070 extGreenLed = 0; 00071 wait(0.01); 00072 } 00073 00074 else if ((dispDist < input && dispDist > 2 * input / 3)){ 00075 PwmOut extRedLed(PTD2); 00076 PwmOut extGreenLed(PTD0);; 00077 extRedLed = 0; 00078 extGreenLed = 1; 00079 wait(0.01); 00080 } 00081 00082 else if (dispDist >= input / 3 & dispDist <= (input / 3) + interDist){ 00083 //Do something 00084 PwmOut extRedLed(PTD2); 00085 PwmOut extGreenLed(PTD0); 00086 extRedLed = 0.95; 00087 extGreenLed = 0; 00088 wait(0.01); 00089 } 00090 else if (dispDist >= (input / 3) + interDist & dispDist <= (input / 3) + 2 * interDist){ 00091 //Do something 00092 PwmOut extRedLed(PTD2); 00093 PwmOut extGreenLed(PTD0); 00094 extRedLed = 0.75; 00095 extGreenLed = 0; 00096 wait(0.01); 00097 } 00098 else if (dispDist >= (input / 3) + 2 * interDist & dispDist <= (input / 3) + 3 * interDist){ 00099 //Do something 00100 PwmOut extRedLed(PTD2); 00101 PwmOut extGreenLed(PTD0); 00102 extRedLed = 0.55; 00103 extGreenLed = 0; 00104 wait(0.01); 00105 } 00106 else if (dispDist >= (input / 3) + 3 * interDist & dispDist <= (input / 3) + 4 * interDist){ 00107 //Do something 00108 PwmOut extRedLed(PTD2); 00109 PwmOut extGreenLed(PTD0); 00110 extRedLed = 0.35; 00111 extGreenLed = 0; 00112 wait(0.01); 00113 } 00114 else if (dispDist >= (input / 3) + 4 * interDist & dispDist <= (input / 3) + 5 * interDist){ 00115 //Do something 00116 PwmOut extRedLed(PTD2); 00117 PwmOut extGreenLed(PTD0); 00118 extRedLed = 0.15; 00119 extGreenLed = 0; 00120 wait(0.01); 00121 } 00122 else if (dispDist < (input / 3)){ 00123 PwmOut extRedLed(PTD2); 00124 PwmOut extGreenLed(PTD0); 00125 extRedLed = 0.95; 00126 extGreenLed = 0; 00127 wait(0.01); 00128 } 00129 00130 if (prevDist > dispDist){ 00131 ledRed = 0; 00132 ledGreen = 0; 00133 ledBlue = 1; 00134 wait(0.01); 00135 } 00136 00137 else if (prevDist < dispDist){ 00138 ledRed = 1; 00139 ledGreen = 1; 00140 ledBlue = 0; 00141 wait(0.01); 00142 } 00143 00144 else{ 00145 ledRed.pulsewidth(1); 00146 ledGreen.pulsewidth(1); 00147 ledBlue.pulsewidth(1); 00148 wait(0.01); 00149 } 00150 00151 prevDist = dispDist; 00152 my_lcd.printf("%.1f", dispDist); 00153 my_lcd.locate(6, 0); 00154 my_lcd.printf("cm"); 00155 wait(0.5); 00156 my_lcd.cls(); 00157 wait(0.01); 00158 } 00159 }
Generated on Sat Oct 8 2022 10:38:44 by
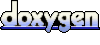