Ray casting engine implemented on the mBuino platform using the ST7735 LCD controller.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "common.h" 00003 #include "LCD_ST7735.h" 00004 #include "Color565.h" 00005 #include "Raycaster.h" 00006 00007 static const uint8_t g_map[] = 00008 { 00009 2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2, 00010 1,0,0,0,0,0,0,1,1,0,0,0,0,0,0,4, 00011 1,0,1,1,1,1,0,1,1,0,1,1,1,1,0,4, 00012 1,0,1,0,0,0,0,1,1,0,0,0,0,1,0,4, 00013 1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,4, 00014 1,1,3,4,3,3,3,0,0,2,2,2,2,2,2,4, 00015 1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,4, 00016 1,0,0,0,0,0,1,0,0,1,0,0,0,0,0,4, 00017 1,1,1,1,0,0,1,0,0,1,0,0,3,3,3,4, 00018 3,0,0,0,0,0,1,0,0,1,0,0,0,0,0,4, 00019 3,0,0,1,1,1,0,0,0,0,1,1,1,0,0,4, 00020 3,0,0,1,0,0,0,0,0,0,0,0,1,0,0,4, 00021 3,0,0,1,0,0,2,4,4,2,0,0,1,0,0,4, 00022 3,0,0,1,0,0,0,0,0,0,0,0,1,0,0,4, 00023 3,0,0,0,0,1,0,0,0,0,1,0,0,0,0,4, 00024 3,1,1,1,1,1,1,1,1,1,1,1,1,1,1,4, 00025 }; 00026 00027 static const uint16_t g_palette[] = 00028 { 00029 Color565::Black, 00030 Color565::Red, 00031 Color565::Green, 00032 Color565::Blue, 00033 Color565::Yellow 00034 }; 00035 00036 AnalogIn stickX(P0_11); 00037 AnalogIn stickY(P0_13); 00038 00039 main() 00040 { 00041 Raycaster rayCaster(0, 10, 160, 108, 00042 96, CELL_SIZE / 2, 00043 g_map, 16, 16, 00044 g_palette); 00045 00046 rayCaster.setCellPosition(8, 8); 00047 while (true) 00048 { 00049 rayCaster.renderFrame(); 00050 00051 if (stickX < 0.3) rayCaster.rotate(-0.1); 00052 else if (stickX > 0.7) rayCaster.rotate(0.1);; 00053 00054 if (stickY < 0.3) rayCaster.move(4); 00055 else if (stickY > 0.7) rayCaster.move(-4); 00056 } 00057 } 00058 00059 00060
Generated on Sat Jul 16 2022 14:43:34 by
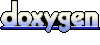