LidarLitev2 Library for distance reading. Capable of both single distance reads and continuous distance read setup.
Dependents: Lidar_Distance Lidar_DistanceContinuous Lidar_2D_Mapping Motions_Secure_Server_IUPUI ... more
LidarLitev2.h
00001 #ifndef LidarLitev2_H 00002 #define LidarLitev2_H 00003 00004 00005 #include "mbed.h" 00006 00007 /** My LidarLite class 00008 * Used for controlling and interacting with the LidarLitev2 00009 Example: 00010 * @code 00011 * //Measures distance from the lidarlite and prints it through serial 00012 * #include "LidarLitev2.h" 00013 * LidarLitev2 Lidar(p28, p27); 00014 * Serial pc(USBTX,USBRX); 00015 * 00016 * 00017 * Timer dt; 00018 * int main() 00019 * { 00020 * 00021 * pc.baud(115200); 00022 * Lidar.configure(); 00023 * dt.start(); 00024 * while(1){ 00025 * pc.printf("distance = %d cm frequency = %.2f Hz\n", Lidar.distance(), 1/dt.read()); 00026 * dt.reset(); 00027 * } 00028 * } 00029 * @endcode 00030 * 00031 * 00032 */ 00033 00034 class LidarLitev2 00035 { 00036 public: 00037 LidarLitev2(PinName sda, PinName scl, bool = true); // Constructor iniates I2C setup 00038 00039 /** Configure the different modes of the Lidar */ 00040 void configure(int = 0, int = 0xc4); // Configure the mode and slave address 00041 00042 /** Sets the Lidar to read continuously, indicating its ready to be read from by the modepin pulling down, a cerntain amount of times */ 00043 void beginContinuous(bool = true, char = 0x04, char = 0xff, int = 0xc4); //Enable if using continous setup with mode from Lidar and pulldown 00044 00045 /** Calclulates distance through I2C protocol of activating sensor with a write, then a write for the register, and a read. */ 00046 int distance(bool = true, bool = true, int = 0xc4); 00047 // Returns distance as a integer in cm 00048 00049 /** 1.) Set Lidar circuit for continuous mode 00050 *2.) utilize the beginContinous function and configure as desired 00051 * This function returns distance without any need to activate the lidar senore through a write command, 00052 * instead the mode pin pulls down when the lidar is ready for a read 00053 * Returns distance as a integer in cm */ 00054 int distanceContinuous(int = 0xc4); 00055 00056 00057 private: 00058 // I2C Functions // 00059 /////////////////// 00060 I2C i2c; // Kept private as to prevent changing the I2C once set in the constructor of LidarLitev2 00061 }; 00062 00063 #endif /* LidarLitev2_H */
Generated on Tue Jul 12 2022 19:59:00 by
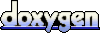