used with BBC microbit
Fork of MAG3110 by
Embed:
(wiki syntax)
Show/hide line numbers
MAG3110.h
00001 /* 00002 * MAG3110 Sensor Library for mbed 00003 * TODO: Add proper header 00004 */ 00005 00006 #ifndef MAG3110_H 00007 #define MAG3110_H 00008 00009 #include "mbed.h" 00010 00011 #define PI 3.14159265359 00012 00013 #define MAG_ADDR 0x1D 00014 00015 // define registers 00016 #define MAG_DR_STATUS 0x00 00017 #define MAG_OUT_X_MSB 0x01 00018 #define MAG_OUT_X_LSB 0x02 00019 #define MAG_OUT_Y_MSB 0x03 00020 #define MAG_OUT_Y_LSB 0x04 00021 #define MAG_OUT_Z_MSB 0x05 00022 #define MAG_OUT_Z_LSB 0x06 00023 #define MAG_WHO_AM_I 0x07 00024 #define MAG_SYSMOD 0x08 00025 #define MAG_OFF_X_MSB 0x09 00026 #define MAG_OFF_X_LSB 0x0A 00027 #define MAG_OFF_Y_MSB 0x0B 00028 #define MAG_OFF_Y_LSB 0x0C 00029 #define MAG_OFF_Z_MSB 0x0D 00030 #define MAG_OFF_Z_LSB 0x0E 00031 #define MAG_DIE_TEMP 0x0F 00032 #define MAG_CTRL_REG1 0x10 00033 #define MAG_CTRL_REG2 0x11 00034 00035 // what should WHO_AM_I return? 00036 #define MAG_3110_WHO_AM_I_VALUE 0xC4 00037 00038 00039 // Fields in registers 00040 // CTRL_REG1: dr2,dr1,dr0 os1,os0 fr tm ac 00041 00042 // Sampling rate from 80Hz down to 0.625Hz 00043 #define MAG_3110_SAMPLE80 0 00044 #define MAG_3110_SAMPLE40 0x20 00045 #define MAG_3110_SAMPLE20 0x40 00046 #define MAG_3110_SAMPLE10 0x60 00047 #define MAG_3110_SAMPLE5 0x80 00048 #define MAG_3110_SAMPLE2_5 0xA0 00049 #define MAG_3110_SAMPLE1_25 0xC0 00050 #define MAG_3110_SAMPLE0_625 0xE0 00051 00052 // How many samples to average (lowers data rate) 00053 #define MAG_3110_OVERSAMPLE1 0 00054 #define MAG_3110_OVERSAMPLE2 0x08 00055 #define MAG_3110_OVERSAMPLE3 0x10 00056 #define MAG_3110_OVERSAMPLE4 0x18 00057 00058 // read only 1 byte per axis 00059 #define MAG_3110_FASTREAD 0x04 00060 // do one measurement (even if in standby mode) 00061 #define MAG_3110_TRIGGER 0x02 00062 // put in active mode 00063 #define MAG_3110_ACTIVE 0x01 00064 00065 // CTRL_REG2: AUTO_MRST_EN _ RAW MAG_RST _ _ _ _ _ 00066 // reset sensor after each reading 00067 #define MAG_3110_AUTO_MRST_EN 0x80 00068 // don't subtract user offsets 00069 #define MAG_3110_RAW 0x20 00070 // reset magnetic sensor after too-large field 00071 #define MAG_3110_MAG_RST 0x10 00072 00073 // DR_STATUS Register ZYXOW ZOW YOW XOW ZYXDR ZDR YDR XDR 00074 #define MAG_3110_ZYXDR 0x08 00075 00076 /** 00077 * MAG3110 Class to read X/Y/Z data from the magentometer 00078 * 00079 */ 00080 class MAG3110 00081 { 00082 public: 00083 /** 00084 * Main constructor 00085 * @param sda SDA pin 00086 * @param sdl SCL pin 00087 * @param addr addr of the I2C peripheral 00088 */ 00089 MAG3110(PinName sda, PinName scl); 00090 /** 00091 * Debug version of constructor 00092 * @param sda SDA pin 00093 * @param sdl SCL pin 00094 * @param addr Address of the I2C peripheral 00095 * @param pc Serial object to output debug messages 00096 */ 00097 MAG3110(PinName sda, PinName scl, Serial *pc); //pass serial for debug 00098 /** 00099 * Setup the Magnetometer 00100 * 00101 */ 00102 void begin(); 00103 /** 00104 * Read a register, return its value as int 00105 * @param regAddr The address to read 00106 * @return value in register 00107 */ 00108 int readReg(char regAddr); 00109 /** 00110 * Read a value from a pair of registers, return as int 00111 * @param regAddr The address to read 00112 * @return Value from 2 consecutive registers 00113 */ 00114 int readVal(char regAddr); 00115 /** 00116 * Calculate the heading 00117 * @return heading in degrees 00118 */ 00119 float getHeading(); 00120 /** 00121 * Perform a read on the X, Y and Z values. 00122 * @param xVal Pointer to X value 00123 * @param yVal Pointer to Y value 00124 * @param zVal Pointer to Z value 00125 */ 00126 void getValues(int *xVal, int *yVal, int *zVal); 00127 /** 00128 * Set the calibration parameters if required. 00129 * @param minX Minimum value for X range 00130 * @param maxX Maximum value for X range 00131 * @param minY Minimum value for Y range 00132 * @param maxY maximum value for Y range 00133 */ 00134 void setCalibration(int minX, int maxX, int minY, int maxY); 00135 00136 private: 00137 I2C _i2c; 00138 int _i2c_address; 00139 Serial *_pc; 00140 bool _debug; 00141 int _avgX, _avgY; 00142 00143 }; 00144 #endif
Generated on Thu Jul 14 2022 06:48:24 by
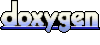