
an observer for temperature demo
Dependencies: BLE_API mbed nRF51822 TMP_nrf51
Fork of BLE_Observer by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLE.h" 00019 #include "TMP_nrf51/TMP_nrf51.h" 00020 00021 #define APP_SPECIFIC_ID_TEST 0xFEFE 00022 00023 #pragma pack(1) 00024 /* Advertising data */ 00025 struct AdvertisingData_t { 00026 uint8_t length; /* doesn't include itself */ 00027 GapAdvertisingData::DataType dataType; 00028 uint8_t data[1]; 00029 }; 00030 00031 struct ApplicationData_t { 00032 uint16_t applicationSpecificId; /* An ID used to identify temperature value 00033 in the manufacture specific AD data field */ 00034 TMP_nrf51::tmpSensorValue_t tmpSensorValue; /* User defined application data */ 00035 }; 00036 #pragma pack() 00037 00038 BLE ble; 00039 DigitalOut led1(LED1); 00040 00041 void periodicCallback(void) 00042 { 00043 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00044 } 00045 00046 void advertisementCallback(const Gap::AdvertisementCallbackParams_t *params) 00047 { 00048 AdvertisingData_t *pAdvData = NULL; 00049 uint8_t len = 0; 00050 00051 /* Search for the manufacturer data */ 00052 while(len < params->advertisingDataLen) { 00053 pAdvData = (AdvertisingData_t *)¶ms->advertisingData[len]; 00054 if(pAdvData->dataType == GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA) { 00055 ApplicationData_t *pAppData = (ApplicationData_t *)pAdvData->data; 00056 if(pAppData->applicationSpecificId == APP_SPECIFIC_ID_TEST) { 00057 printf("From [%02x %02x %02x], ", params->peerAddr[2], params->peerAddr[1], params->peerAddr[0]); 00058 printf("Temp is %f\r\n", (float)pAppData->tmpSensorValue); 00059 break; 00060 } 00061 } 00062 len += (pAdvData->length + 1); 00063 } 00064 } 00065 00066 int main(void) 00067 { 00068 led1 = 1; 00069 Ticker ticker; 00070 ticker.attach(periodicCallback, 1); 00071 00072 ble.init(); 00073 ble.gap().setScanParams(1800 /* scan interval */, 1500 /* scan window */); 00074 ble.gap().startScan(advertisementCallback); 00075 00076 while (true) { 00077 ble.waitForEvent(); 00078 } 00079 }
Generated on Wed Jul 13 2022 10:06:16 by
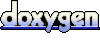