
a fork to test out advertising process
Dependencies: BLE_API TxIR mbed nRF51822
Fork of ir-puck by
IR.cpp
00001 #include "mbed.h" 00002 #include "IR.h" 00003 #include "TxIR.hpp" 00004 00005 #define WORD(x, i) ((x)[(i)] << 8 | (x)[(i) + 1]) 00006 00007 TxIR txir(p14); 00008 00009 #define DEBUG 1 00010 #ifdef DEBUG 00011 Serial px(USBTX, USBRX); 00012 #define LOG(args...) px.printf(args) 00013 #else 00014 #define LOG(args...) 00015 #endif 00016 00017 void fireIRCode(uint8_t* header, uint8_t* one, uint8_t* zero, uint8_t* ptrail, uint8_t* predata, uint8_t* code) { 00018 LOG("Going to fire.\n"); 00019 int raw_codes_length = 67; 00020 unsigned raw_codes[raw_codes_length]; 00021 raw_codes[0] = WORD(header, 0); 00022 raw_codes[1] = WORD(header, 2); 00023 int offset = 2; 00024 for(int i = 0; i < 16 * 2; i += 2) { 00025 int bit = predata[i / 16] & 0x80; 00026 uint8_t* signal = bit ? one : zero; 00027 raw_codes[i + offset] = WORD(signal, 0); 00028 raw_codes[i + offset + 1] = WORD(signal, 2); 00029 predata[i / 16] <<= 1; 00030 } 00031 offset = 34; 00032 for(int i = 0; i < 16 * 2; i += 2) { 00033 int bit = code[i / 16] & 0x80; 00034 uint8_t* signal = bit ? one : zero; 00035 raw_codes[i + offset] = WORD(signal, 0); 00036 raw_codes[i + offset + 1] = WORD(signal, 2); 00037 code[i / 16] <<= 1; 00038 } 00039 raw_codes[67] = WORD(ptrail, 0); 00040 00041 txir.txSeq(26.32 / 1000000.0, 67, raw_codes); 00042 00043 LOG("Wrote message :)\n"); 00044 }
Generated on Wed Jul 13 2022 08:54:52 by
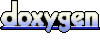