Library for big numbers from http://www.ttmath.org/
Dependents: PIDHeater82 Conceptcontroller_v_1_0 AlarmClockApp COG4050_adxl355_tilt ... more
Parser< ValueType > Class Template Reference
Mathematical parser. More...
#include <ttmathparser.h>
Data Structures | |
struct | Item |
Public Member Functions | |
bool | GetValueOfUserDefinedVariable (const std::string &variable_name, ValueType &result) |
bool | GetValueOfFunctionLocalVariable (const std::string &variable_name, ValueType &result) |
ValueType | GetValueOfVariable (const std::string &variable_name) |
Parser () | |
Parser< ValueType > & | operator= (const Parser< ValueType > &p) |
Parser (const Parser< ValueType > &p) | |
void | SetBase (int b) |
void | SetDegRadGrad (int angle) |
void | SetStopObject (const volatile StopCalculating *ps) |
void | SetVariables (const Objects *pv) |
void | SetFunctions (const Objects *pf) |
void | SetGroup (int g) |
void | SetComma (int c, int c2=0) |
void | SetParamSep (int s) |
ErrorCode | Parse (const char *str) |
ErrorCode | Parse (const std::string &str) |
ErrorCode | Parse (const wchar_t *str) |
ErrorCode | Parse (const std::wstring &str) |
bool | Calculated () |
void | InitCGamma () |
Data Fields | |
std::vector< Item > | stack |
Detailed Description
template<class ValueType>
class ttmath::Parser< ValueType >
Mathematical parser.
let x will be an input string meaning an expression for converting:
x = [+|-]Value[operator[+|-]Value][operator[+|-]Value]... where: an operator can be: ^ (pow) (the heighest priority)
(mul) (or multiplication without an operator -- short mul) / (div) (* and / have the same priority)
+ (add)
- (sub) (+ and - have the same priority)
< (lower than) > (greater than) <= (lower or equal than) >= (greater or equal than) == (equal) != (not equal) (all above logical operators have the same priority)
&& (logical and)
|| (logical or) (the lowest priority)
short mul: if the second Value (Var below) is either a variable or function there might not be an operator between them, e.g. "[+|-]Value Var" is treated as "[+|-]Value * Var" and the multiplication has the same priority as a normal multiplication: 4x = 4 * x 2^3m = (2^3)* m 6h^3 = 6 * (h^3) 2sin(pi) = 2 * sin(pi) etc.
Value can be: constant e.g. 100, can be preceded by operators for changing the base (radix): [#|&] # - hex & - bin sample: #10 = 16 &10 = 2 variable e.g. pi another expression between brackets e.g (x) function e.g. sin(x)
for example a correct input string can be: "1" "2.1234" "2,1234" (they are the same, by default we can either use a comma or a dot) "1 + 2" "(1 + 2) * 3" "pi" "sin(pi)" "(1+2)*(2+3)" "log(2;1234)" there's a semicolon here (not a comma), we use it in functions for separating parameters "1 < 2" (the result will be: 1) "4 < 3" (the result will be: 0) "2+x" (of course if the variable 'x' is defined) "4x+10" "#20+10" = 32 + 10 = 42 "10 ^ -&101" = 10 ^ -5 = 0.00001 "8 * -&10" = 8 * -2 = -16 etc.
we can also use a semicolon for separating any 'x' input strings for example: "1+2;4+5" the result will be on the stack as follows: stack[0].value=3 stack[1].value=9
Definition at line 138 of file ttmathparser.h.
Constructor & Destructor Documentation
Parser | ( | ) |
the default constructor
Definition at line 2527 of file ttmathparser.h.
the copying constructor
Definition at line 2582 of file ttmathparser.h.
Member Function Documentation
bool Calculated | ( | ) |
this method returns true is something was calculated (at least one mathematical operator was used or a function or variable) e.g. true if the string to Parse() looked like this: "1+1" "2*3" "sin(5)"
if the string was e.g. "678" the result is false
Definition at line 2752 of file ttmathparser.h.
bool GetValueOfFunctionLocalVariable | ( | const std::string & | variable_name, |
ValueType & | result | ||
) |
this method returns the value of a local variable of a function
Definition at line 618 of file ttmathparser.h.
bool GetValueOfUserDefinedVariable | ( | const std::string & | variable_name, |
ValueType & | result | ||
) |
this method returns the user-defined value of a variable
Definition at line 598 of file ttmathparser.h.
ValueType GetValueOfVariable | ( | const std::string & | variable_name ) |
this method returns the value of a variable from variables' table
we make an object of type ValueType then call a method which sets the correct value in it and finally we'll return the object
Definition at line 640 of file ttmathparser.h.
void InitCGamma | ( | ) |
initializing coefficients used when calculating the gamma (or factorial) function this speed up the next calculations you don't have to call this method explicitly these coefficients will be calculated when needed
Definition at line 2764 of file ttmathparser.h.
the assignment operator
Definition at line 2550 of file ttmathparser.h.
ErrorCode Parse | ( | const wchar_t * | str ) |
the main method using for parsing string
Definition at line 2721 of file ttmathparser.h.
ErrorCode Parse | ( | const std::wstring & | str ) |
the main method using for parsing string
Definition at line 2734 of file ttmathparser.h.
ErrorCode Parse | ( | const char * | str ) |
the main method using for parsing string
Definition at line 2682 of file ttmathparser.h.
ErrorCode Parse | ( | const std::string & | str ) |
the main method using for parsing string
Definition at line 2710 of file ttmathparser.h.
void SetBase | ( | int | b ) |
the new base of mathematic system default is 10
Definition at line 2592 of file ttmathparser.h.
void SetComma | ( | int | c, |
int | c2 = 0 |
||
) |
setting the main comma operator and the additional comma operator the additional operator can be zero (which means it is not used) default are: '.' and ','
Definition at line 2660 of file ttmathparser.h.
void SetDegRadGrad | ( | int | angle ) |
the unit of angles used in: sin,cos,tan,cot,asin,acos,atan,acot 0 - deg 1 - rad (default) 2 - grad
Definition at line 2605 of file ttmathparser.h.
void SetFunctions | ( | const Objects * | pf ) |
this method sets the new table of user-defined functions if you don't want any other functions just put zero value into the 'puser_functions' variable
(you can have only one table at the same time)
Definition at line 2639 of file ttmathparser.h.
void SetGroup | ( | int | g ) |
setting the group character default zero (not used)
Definition at line 2649 of file ttmathparser.h.
void SetParamSep | ( | int | s ) |
setting an additional character which is used as a parameters separator the main parameters separator is a semicolon (is used always)
this character is used also as a global separator
Definition at line 2673 of file ttmathparser.h.
void SetStopObject | ( | const volatile StopCalculating * | ps ) |
this method sets a pointer to the object which tell us whether we should stop calculations
Definition at line 2615 of file ttmathparser.h.
void SetVariables | ( | const Objects * | pv ) |
this method sets the new table of user-defined variables if you don't want any other variables just put zero value into the 'puser_variables' variable
(you can have only one table at the same time)
Definition at line 2627 of file ttmathparser.h.
Field Documentation
stack on which we're keeping the Items
at the end of parsing we'll have the result here the result don't have to be one value, it can be more than one if we have used a semicolon in the global space e.g. such input string "1+2;3+4" will generate a result: stack[0].value=3 stack[1].value=7
you should check if the stack is not empty, because if there was a syntax error in the input string then we do not have any results on the stack
Definition at line 296 of file ttmathparser.h.
Generated on Tue Jul 12 2022 14:03:18 by
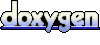