Library for big numbers from http://www.ttmath.org/
Dependents: PIDHeater82 Conceptcontroller_v_1_0 AlarmClockApp COG4050_adxl355_tilt ... more
Objects Class Reference
#include <ttmathobjects.h>
Data Structures | |
struct | Item |
Public Member Functions | |
bool | IsDefined (const std::string &name) |
bool | IsDefined (const std::wstring &name) |
ErrorCode | Add (const std::string &name, const std::string &value, int param=0) |
ErrorCode | Add (const std::wstring &name, const std::wstring &value, int param=0) |
bool | Empty () const |
void | Clear () |
CIterator | Begin () const |
CIterator | End () const |
ErrorCode | EditValue (const std::string &name, const std::string &value, int param=0) |
ErrorCode | EditValue (const std::wstring &name, const std::wstring &value, int param=0) |
ErrorCode | EditName (const std::string &old_name, const std::string &new_name) |
ErrorCode | EditName (const std::wstring &old_name, const std::wstring &new_name) |
ErrorCode | Delete (const std::string &name) |
ErrorCode | Delete (const std::wstring &name) |
ErrorCode | GetValue (const std::string &name, std::string &value) const |
ErrorCode | GetValue (const std::wstring &name, std::wstring &value) |
ErrorCode | GetValue (const std::string &name, const char **value) const |
ErrorCode | GetValue (const std::wstring &name, const char **value) |
ErrorCode | GetValueAndParam (const std::string &name, std::string &value, int *param) const |
ErrorCode | GetValueAndParam (const std::wstring &name, std::wstring &value, int *param) |
ErrorCode | GetValueAndParam (const std::string &name, const char **value, int *param) const |
ErrorCode | GetValueAndParam (const std::wstring &name, const char **value, int *param) |
Table * | GetTable () |
Static Public Member Functions | |
static bool | CorrectCharacter (int c, bool can_be_digit) |
template<class string_type > | |
static bool | IsNameCorrect (const string_type &name) |
Detailed Description
objects of this class are used with the mathematical parser they hold variables or functions defined by a user
each object has its own table in which we're keeping variables or functions
Definition at line 65 of file ttmathobjects.h.
Member Function Documentation
ErrorCode Add | ( | const std::string & | name, |
const std::string & | value, | ||
int | param = 0 |
||
) |
this method adds one object (variable of function) into the table
Definition at line 175 of file ttmathobjects.h.
ErrorCode Add | ( | const std::wstring & | name, |
const std::wstring & | value, | ||
int | param = 0 |
||
) |
this method adds one object (variable of function) into the table
Definition at line 197 of file ttmathobjects.h.
CIterator Begin | ( | ) | const |
this method returns 'const_iterator' on the first item on the table
Definition at line 234 of file ttmathobjects.h.
void Clear | ( | ) |
this method clears the table
Definition at line 225 of file ttmathobjects.h.
static bool CorrectCharacter | ( | int | c, |
bool | can_be_digit | ||
) | [static] |
this method returns true if a character 'c' is a character which can be in a name
if 'can_be_digit' is true that means when the 'c' is a digit this method returns true otherwise it returns false
Definition at line 102 of file ttmathobjects.h.
ErrorCode Delete | ( | const std::wstring & | name ) |
this method deletes an object
Definition at line 371 of file ttmathobjects.h.
ErrorCode Delete | ( | const std::string & | name ) |
this method deletes an object
Definition at line 349 of file ttmathobjects.h.
ErrorCode EditName | ( | const std::string & | old_name, |
const std::string & | new_name | ||
) |
this method changes the name of a specific object
Definition at line 295 of file ttmathobjects.h.
ErrorCode EditName | ( | const std::wstring & | old_name, |
const std::wstring & | new_name | ||
) |
this method changes the name of a specific object
Definition at line 330 of file ttmathobjects.h.
ErrorCode EditValue | ( | const std::string & | name, |
const std::string & | value, | ||
int | param = 0 |
||
) |
this method changes the value and the number of parameters for a specific object
Definition at line 253 of file ttmathobjects.h.
ErrorCode EditValue | ( | const std::wstring & | name, |
const std::wstring & | value, | ||
int | param = 0 |
||
) |
this method changes the value and the number of parameters for a specific object
Definition at line 276 of file ttmathobjects.h.
bool Empty | ( | ) | const |
this method returns 'true' if the table is empty
Definition at line 216 of file ttmathobjects.h.
CIterator End | ( | ) | const |
this method returns 'const_iterator' pointing at the space after last item (returns table.end())
Definition at line 244 of file ttmathobjects.h.
Table* GetTable | ( | ) |
this method returns a pointer into the table
Definition at line 576 of file ttmathobjects.h.
ErrorCode GetValue | ( | const std::string & | name, |
std::string & | value | ||
) | const |
this method gets the value of a specific object
Definition at line 389 of file ttmathobjects.h.
ErrorCode GetValue | ( | const std::wstring & | name, |
std::wstring & | value | ||
) |
this method gets the value of a specific object
Definition at line 413 of file ttmathobjects.h.
ErrorCode GetValue | ( | const std::wstring & | name, |
const char ** | value | ||
) |
this method gets the value of a specific object (this version is used for not copying the whole string)
Definition at line 459 of file ttmathobjects.h.
ErrorCode GetValue | ( | const std::string & | name, |
const char ** | value | ||
) | const |
this method gets the value of a specific object (this version is used for not copying the whole string)
Definition at line 434 of file ttmathobjects.h.
ErrorCode GetValueAndParam | ( | const std::string & | name, |
const char ** | value, | ||
int * | param | ||
) | const |
this method sets the value and the number of parameters of a specific object (this version is used for not copying the whole string)
Definition at line 527 of file ttmathobjects.h.
ErrorCode GetValueAndParam | ( | const std::wstring & | name, |
std::wstring & | value, | ||
int * | param | ||
) |
this method gets the value and the number of parameters of a specific object
Definition at line 505 of file ttmathobjects.h.
ErrorCode GetValueAndParam | ( | const std::string & | name, |
std::string & | value, | ||
int * | param | ||
) | const |
this method gets the value and the number of parameters of a specific object
Definition at line 478 of file ttmathobjects.h.
ErrorCode GetValueAndParam | ( | const std::wstring & | name, |
const char ** | value, | ||
int * | param | ||
) |
this method sets the value and the number of parameters of a specific object (this version is used for not copying the whole string but in fact we make one copying during AssignString())
Definition at line 557 of file ttmathobjects.h.
bool IsDefined | ( | const std::string & | name ) |
this method returns true if such an object is defined (name exists)
Definition at line 139 of file ttmathobjects.h.
bool IsDefined | ( | const std::wstring & | name ) |
this method returns true if such an object is defined (name exists)
Definition at line 157 of file ttmathobjects.h.
static bool IsNameCorrect | ( | const string_type & | name ) | [static] |
this method returns true if the name can be as a name of an object
Definition at line 118 of file ttmathobjects.h.
Generated on Tue Jul 12 2022 14:03:18 by
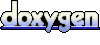