OS6. i2s BME280 library, also works with BMP280 without Humidity. See BME280.h for example code.
Embed:
(wiki syntax)
Show/hide line numbers
BME280.h
00001 #ifndef BME_H 00002 #define BME_H 00003 00004 #include "mbed.h" 00005 00006 #define _debug 1 // '1' to enable prinf BME's registers 00007 00008 /* 00009 Bosch Sensortec BMP280 BME280 i2c example code 00010 using 64bit numeric calculations as recomended by Bosch. 00011 Some sensors supplied from Chinese suppliers may have 'suspect' 00012 quality sensor with different i2c address. 00013 My sensors were address 0xEC and all the ones I have 00014 differ quite a bit in tolerance :( so be aware if accuracy is paramount. 00015 */ 00016 00017 /* 00018 #include "mbed.h" 00019 #include "BME280.h" 00020 00021 BME sensor(D0,D1, 0xEC); // sda, clk, 8bit address (NUCLEO-L432) 00022 DigitalOut led(LED1); 00023 00024 float temp, humidity, pressure, altitude; 00025 00026 int main() 00027 { 00028 00029 int BMPE_id = sensor.init(); // initialise and get sensor id 00030 00031 if(!BMPE_id) { 00032 printf("No sensor detected!!\n"); 00033 while(1){ 00034 led=!led; 00035 ThisThread::sleep_for(200ms); 00036 } 00037 } 00038 00039 if(_debug){ // set _debug to '1' to see BME's registers 00040 ThisThread::sleep_for(2s); 00041 } 00042 00043 while(1) { 00044 led=1; 00045 printf("\033[0m\033[2J\033[H------- BME280-BMP280 Sensor example -------\r\n\n"); 00046 if(BMPE_id==0x60) { 00047 printf("BME280 detected id: 0x%x\n\n",BMPE_id); 00048 } else if(BMPE_id==0x58) { 00049 printf("BMP280 detected id: 0x%x\n\n",BMPE_id); 00050 } 00051 00052 temp = sensor.getTemperature(); 00053 00054 printf("Temperature: %3.2f %cc \n",temp,0xb0); 00055 00056 if(sensor.chip_id==0x60) { // only BME has Humidity 00057 humidity = sensor.getHumidity(); 00058 printf("Humidity: %2.2f %cRh \n", humidity,0x25); 00059 } 00060 pressure = sensor.getPressure(); 00061 printf("Pressure: %4.2f mbar's \n\n",pressure); 00062 00063 altitude = 44330.0f*( 1.0f - pow((pressure/1013.25f), (1.0f/5.255f)))+18; // Calculate altitude in meters 00064 printf("Altitude: %3.1f meters %4.1f feet \r\n(Referenced to 1,013.25 millibars @ sea level) \r\n\n", altitude,altitude*3.2810f); 00065 led=0; 00066 ThisThread::sleep_for(1s); 00067 } 00068 } 00069 */ 00070 00071 class BME 00072 { 00073 public: 00074 00075 BME(PinName sda, PinName scl, char slave_adr); 00076 00077 int init(void); 00078 int chipID(void); 00079 float getTemperature(void); 00080 float getPressure(void); 00081 float getHumidity(void); 00082 00083 uint16_t chip_id; 00084 00085 private: 00086 00087 I2C bme; 00088 void initialize(void); 00089 char address; 00090 uint16_t dig_T1; 00091 int16_t dig_T2, dig_T3; 00092 uint16_t dig_P1; 00093 int16_t dig_P2, dig_P3, dig_P4, dig_P5, dig_P6, dig_P7, dig_P8, dig_P9; 00094 uint16_t dig_H1, dig_H3; 00095 int16_t dig_H2, dig_H4, dig_H5, dig_H6; 00096 int32_t t_fine; 00097 bool dbg_on; 00098 }; 00099 #endif // BME_H
Generated on Tue Jul 12 2022 17:41:07 by
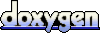