BMP180 Pressure/Temperature Sensor library
Dependents: frdm_mikroklimat Weather_Station WMG Sample_BMP180 ... more
BMP180 Class Reference
#include <BMP180.h>
Public Member Functions | |
BMP180 (PinName sda, PinName scl, int address=BMP180_I2C_ADDRESS) | |
Create a BMP180 instance. | |
BMP180 (I2C &i2c, int address=BMP180_I2C_ADDRESS) | |
Create a BMP180 instance. | |
int | Initialize (float altitude=0.F, int overSamplingSetting=BMP180_OSS_NORMAL) |
Initialization: set member values and read BMP180 calibration parameters. | |
int | ReadData (float *pTemperature=NULL, float *pPressure=NULL) |
Read pressure and temperature from the BMP180. | |
float | GetTemperature () |
Get temperature from a previous measurement. | |
float | GetPressure () |
Get pressure from a previous measurement. | |
Protected Member Functions | |
int | ReadRawTemperature (long *pUt) |
Perform temperature measurement. | |
int | ReadRawPressure (long *pUp) |
Perform pressure measurement. | |
float | TrueTemperature (long ut) |
Calculation of the temperature from the digital output. | |
float | TruePressure (long up) |
Calculation of the pressure from the digital output. |
Detailed Description
BMP180 class.
Read Pressure and temperature from the BMP180 Breakout I2C sensor
Example:
#include "mbed.h" #include "BMP180.h" #if defined(TARGET_LPC1768) #define PIN_SDA p9 #define PIN_SCL p10 #elif defined(TARGET_KL25Z) // watch out for the PTE0/PTE1 mixed up in the KL25Z doc #define PIN_SDA PTE0 #define PIN_SCL PTE1 #endif int main() { BMP180 bmp180(PIN_SDA, PIN_SCL); float pressure, temperature; // bmp180.Initialize(); // no altitude compensation and normal oversampling bmp180.Initialize(64, BMP180_OSS_ULTRA_LOW_POWER); // 64m altitude compensation and low power oversampling while(1) { if (bmp180.ReadData(&pressure, &temperature)) printf("Pressure(hPa): %8.2f \t Temperature(C): %8.2f\n", pressure, temperature); wait(1); } }
Definition at line 67 of file BMP180.h.
Constructor & Destructor Documentation
BMP180 | ( | PinName | sda, |
PinName | scl, | ||
int | address = BMP180_I2C_ADDRESS |
||
) |
Create a BMP180 instance.
- Parameters:
-
sda pin scl pin address,: I2C slave address
Definition at line 24 of file BMP180.cpp.
BMP180 | ( | I2C & | i2c, |
int | address = BMP180_I2C_ADDRESS |
||
) |
Create a BMP180 instance.
- Parameters:
-
i2c object address,: I2C slave address
Definition at line 33 of file BMP180.cpp.
Member Function Documentation
float GetPressure | ( | ) |
float GetTemperature | ( | ) |
int Initialize | ( | float | altitude = 0.F , |
int | overSamplingSetting = BMP180_OSS_NORMAL |
||
) |
Initialization: set member values and read BMP180 calibration parameters.
- Parameters:
-
altitude (in meter) overSamplingSetting
Definition at line 42 of file BMP180.cpp.
int ReadData | ( | float * | pTemperature = NULL , |
float * | pPressure = NULL |
||
) |
Read pressure and temperature from the BMP180.
- Parameters:
-
pressure (hPa) temperature (C)
- Returns:
- 1 on success, 0 on error
Definition at line 90 of file BMP180.cpp.
int ReadRawPressure | ( | long * | pUp ) | [protected] |
int ReadRawTemperature | ( | long * | pUt ) | [protected] |
float TruePressure | ( | long | up ) | [protected] |
Calculation of the pressure from the digital output.
Definition at line 197 of file BMP180.cpp.
float TrueTemperature | ( | long | ut ) | [protected] |
Calculation of the temperature from the digital output.
Definition at line 183 of file BMP180.cpp.
Generated on Mon Jul 18 2022 05:11:00 by
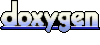