BMP180 Pressure/Temperature Sensor library
Dependents: frdm_mikroklimat Weather_Station WMG Sample_BMP180 ... more
BMP180.h
00001 /* 00002 @file BMP180.h 00003 00004 @brief Barometric Pressure and Temperature Sensor BMP180 Breakout I2C Library 00005 00006 @Author spiridion (http://mailme.spiridion.net) 00007 00008 Tested on LPC1768 and FRDM-KL25Z 00009 00010 Copyright (c) 2014 spiridion 00011 Released under the MIT License (see http://mbed.org/license/mit) 00012 00013 Documentation regarding the BMP180 can be found here: 00014 http://mbed.org/media/uploads/spiridion/bst-bmp180-ds000-09.pdf 00015 */ 00016 00017 #ifndef BMP180_H 00018 #define BMP180_H 00019 00020 #include "mbed.h" 00021 00022 /// default address is 0xEF 00023 #define BMP180_I2C_ADDRESS 0xEF 00024 00025 // Oversampling settings 00026 #define BMP180_OSS_ULTRA_LOW_POWER 0 // 1 sample and 4.5ms for conversion 00027 #define BMP180_OSS_NORMAL 1 // 2 samples and 7.5ms for conversion 00028 #define BMP180_OSS_HIGH_RESOLUTION 2 // 4 samples and 13.5ms for conversion 00029 #define BMP180_OSS_ULTRA_HIGH_RESOLUTION 3 // 8 samples and 25.5ms for conversion 00030 00031 #define UNSET_BMP180_PRESSURE_VALUE 0.F 00032 #define UNSET_BMP180_TEMPERATURE_VALUE -273.15F // absolute zero 00033 00034 /** BMP180 class. 00035 * Read Pressure and temperature from the BMP180 Breakout I2C sensor 00036 * 00037 * Example: 00038 * @code 00039 * #include "mbed.h" 00040 * #include "BMP180.h" 00041 * 00042 * #if defined(TARGET_LPC1768) 00043 * #define PIN_SDA p9 00044 * #define PIN_SCL p10 00045 * #elif defined(TARGET_KL25Z) // watch out for the PTE0/PTE1 mixed up in the KL25Z doc 00046 * #define PIN_SDA PTE0 00047 * #define PIN_SCL PTE1 00048 * #endif 00049 * 00050 * int main() 00051 * { 00052 * BMP180 bmp180(PIN_SDA, PIN_SCL); 00053 * float pressure, temperature; 00054 * 00055 * // bmp180.Initialize(); // no altitude compensation and normal oversampling 00056 * bmp180.Initialize(64, BMP180_OSS_ULTRA_LOW_POWER); // 64m altitude compensation and low power oversampling 00057 * 00058 * while(1) 00059 * { 00060 * if (bmp180.ReadData(&pressure, &temperature)) 00061 * printf("Pressure(hPa): %8.2f \t Temperature(C): %8.2f\n", pressure, temperature); 00062 * wait(1); 00063 * } 00064 * } 00065 * @endcode 00066 */ 00067 class BMP180 00068 { 00069 00070 public: 00071 00072 /** Create a BMP180 instance 00073 * @param sda pin 00074 * @param scl pin 00075 * @param address: I2C slave address 00076 */ 00077 BMP180(PinName sda, PinName scl, int address = BMP180_I2C_ADDRESS); 00078 00079 /** Create a BMP180 instance 00080 * @param i2c object 00081 * @param address: I2C slave address 00082 */ 00083 BMP180(I2C& i2c, int address = BMP180_I2C_ADDRESS); 00084 00085 /** Initialization: set member values and read BMP180 calibration parameters 00086 * @param altitude (in meter) 00087 * @param overSamplingSetting 00088 */ 00089 int Initialize(float altitude = 0.F, int overSamplingSetting = BMP180_OSS_NORMAL); 00090 00091 /** Read pressure and temperature from the BMP180. 00092 * @param pressure (hPa) 00093 * @param temperature (C) 00094 * @returns 00095 * 1 on success, 00096 * 0 on error 00097 */ 00098 int ReadData(float* pTemperature = NULL, float* pPressure = NULL); 00099 00100 /** Get temperature from a previous measurement 00101 * 00102 * @returns 00103 * temperature (C) 00104 */ 00105 float GetTemperature() {return m_temperature;}; 00106 00107 /** Get pressure from a previous measurement 00108 * 00109 * @returns 00110 * pressure (hPa) 00111 */ 00112 float GetPressure() {return m_pressure;}; 00113 00114 protected: 00115 00116 /** Perform temperature measurement 00117 * 00118 * @returns 00119 * temperature (C) 00120 */ 00121 int ReadRawTemperature(long* pUt); 00122 00123 /** Perform pressure measurement 00124 * 00125 * @returns 00126 * temperature (C) 00127 */ 00128 int ReadRawPressure(long* pUp); 00129 00130 /** Calculation of the temperature from the digital output 00131 */ 00132 float TrueTemperature(long ut); 00133 00134 /** Calculation of the pressure from the digital output 00135 */ 00136 float TruePressure(long up); 00137 00138 int m_oss; 00139 float m_temperature; 00140 float m_pressure; 00141 float m_altitude; 00142 00143 I2C m_i2c; 00144 int m_addr; 00145 char m_data[4]; 00146 00147 short ac1, ac2, ac3; 00148 unsigned short ac4, ac5, ac6; 00149 short b1, b2; 00150 short mb, mc, md; 00151 long x1, x2, x3, b3, b5, b6; 00152 unsigned long b4, b7; 00153 00154 }; 00155 00156 #endif
Generated on Mon Jul 18 2022 05:11:00 by
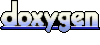