Library to control Silicon Labs SI570 10 MHZ TO 1.4 GHZ I2C PROGRAMMABLE XO/VCXO.
SI570 Class Reference
Interface to the popular SI570 VCXO. More...
#include <SI570.h>
Public Member Functions | |
SI570 (PinName sda, PinName scl, int address) | |
Create an instance of the SI570 connected to specified I2C pins, with the specified address. | |
void | si570reset (void) |
Resets the SI570. | |
double | get_frequency (void) |
Read the current frequency. | |
double | get_rfreq (void) |
Read the current rfreq value. | |
int | get_n1 (void) |
Read the current n1. | |
int | get_hsdiv (void) |
Read the current hsdiv. | |
int | set_frequency (double frequency) |
Set a new frequency. |
Detailed Description
Interface to the popular SI570 VCXO.
Definition at line 40 of file SI570.h.
Constructor & Destructor Documentation
SI570 | ( | PinName | sda, |
PinName | scl, | ||
int | address | ||
) |
Create an instance of the SI570 connected to specified I2C pins, with the specified address.
Example:
#include "mbed.h" #include "TextLCD.h" #include "SI570.h" #include "QEI.h" TextLCD lcd(p11, p12, p15, p16, p29, p30); // rs, e, d0-d3 SI570 si570(p9, p10, 0xAA); QEI wheel (p5, p6, NC, 360); int main() { int wp,swp=0; float startfreq=7.0; float freq; while (1) { wp = wheel.getPulses(); freq=startfreq+wp*0.00001; if (swp != wp) { si570.set_frequency(freq); swp = wp; } lcd.locate(0,0); lcd.printf("%f MHz", si570.get_frequency()); } }
- Parameters:
-
sda The I2C data pin scl The I2C clock pin address The I2C address for this SI570
Member Function Documentation
double get_frequency | ( | void | ) |
int get_hsdiv | ( | void | ) |
int get_n1 | ( | void | ) |
double get_rfreq | ( | void | ) |
int set_frequency | ( | double | frequency ) |
Set a new frequency.
Set the SI570 registers to output frequency When the new frequency is within the 3500 PPM range of the center frequency, hsdiv and n1 needs not to be reprogrammed, resulting in a glitch free transition. For changes larger than 3500 PPM, the process of freezing and unfreezing the DCO will cause the output clock to momentarily stop and start at any arbitrary point during a clock cycle. This process can take up to 10 ms.
- Parameters:
-
frequency the frequency to set.
- Returns:
- the current frequency
Generated on Tue Jul 12 2022 19:03:22 by
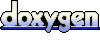