
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskGPS.h
00001 /** 00002 * @file TaskGPS.h 00003 * 00004 * @author Adrian 00005 * @date 30.05.2016 00006 * 00007 */ 00008 #include "uBlox.h " 00009 #include "UBloxGPSMessage.h " 00010 #include "main.h" 00011 00012 #ifndef TASKGPS_H_ 00013 #define TASKGPS_H_ 00014 00015 /** 00016 * @class TaskGPS 00017 * @brief This TaskGPS Class handles the GPS measurement using the uBlox. 00018 * Starting the task using the start() starts the measurement. 00019 * It can be used alongside with other measurement Tasks inside the mbed::rtos 00020 * environment. The Task Class basically wraps mbeds Thread functionality. 00021 */ 00022 class TaskGPS { 00023 public: 00024 TaskGPS(uBlox*,Mutex*, Queue<UBloxGPSMessage,GPS_QUEUE_LENGHT>*); 00025 TaskGPS(uBlox*,Mutex*,Queue<UBloxGPSMessage,GPS_QUEUE_LENGHT>*, 00026 osPriority, uint32_t, unsigned char*); 00027 virtual ~TaskGPS(); 00028 00029 00030 /** 00031 * Starts the task by building and its measurement 00032 * @return 00033 */ 00034 osStatus start(); 00035 00036 /** 00037 * Stops the task. Should only be used after start() was used 00038 * @return 00039 */ 00040 osStatus stop(); 00041 00042 00043 /** 00044 * Gets the actual state of the Task either RUNNING or SLEEPING 00045 * @return 00046 */ 00047 TASK_STATE getState(); 00048 00049 private: 00050 rtos::Thread* thread; 00051 rtos::Queue<UBloxGPSMessage,GPS_QUEUE_LENGHT>* queue; 00052 rtos::Mutex* mutexUART ; 00053 osPriority priority ; 00054 uint32_t stack_size; 00055 unsigned char *stack_pointer; 00056 00057 TASK_STATE state; 00058 00059 uBlox* mUBlox; 00060 uBLOX_MODE uBloxMode; 00061 00062 00063 /** 00064 * @brief A Callback function thats called by the mbed::Thread of this TaskClass 00065 * @param 00066 */ 00067 static void callBack(void const *); 00068 00069 /** 00070 * @brief A thread safe method that acquires GPS values. After acquiring GPS Data 00071 * it stores the data inside a uBLoxGPSMessage 00072 */ 00073 void measureGps(); 00074 00075 00076 /** 00077 * @brief Sets the message Queue of the Task where the acquired data will be stored 00078 * after the acquisition 00079 * @param queueGps the queue where the MPU9250AccelerationMessage will be stored 00080 */ 00081 void setQueue(Queue<UBloxGPSMessage,GPS_QUEUE_LENGHT>* queueGps); 00082 00083 /** 00084 * @brief Sets the mutex thats used for a thread safe acquisition 00085 * @param mutexI2C the I2C mutex 00086 */ 00087 void setMutex(Mutex* mutexI2C); 00088 00089 /** 00090 * @brief Sets the priority of the Task 00091 * @param priority priority of the Task 00092 */ 00093 void setPriority(osPriority priority); 00094 00095 /** 00096 * @brief Sets the size of the Task 00097 * @param stackSize the stack size in Bytes 00098 */ 00099 void setStackSize(uint32_t stackSize); 00100 00101 /** 00102 * @brief Sets the stack pointer of for the task stack 00103 * @param stackPointer 00104 */ 00105 void setStackPointer(unsigned char* stackPointer); 00106 00107 00108 /** 00109 * @brief Sets the actual state of the Task 00110 * @param taskState either RUNNING or SLEEPING 00111 */ 00112 void setState(TASK_STATE taskState); 00113 }; 00114 00115 #endif /* TASKGPS_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
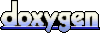