CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
math_helper.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * 00009 * Title: math_helper.c 00010 * 00011 * Description: Definition of all helper functions required. 00012 * 00013 * Target Processor: Cortex-M4/Cortex-M3 00014 * 00015 * Version 1.0.3 2010/11/29 00016 * Re-organized the CMSIS folders and updated documentation. 00017 * 00018 * Version 1.0.2 2010/11/11 00019 * Documentation updated. 00020 * 00021 * Version 1.0.1 2010/10/05 00022 * Production release and review comments incorporated. 00023 * 00024 * Version 1.0.0 2010/09/20 00025 * Production release and review comments incorporated. 00026 * 00027 * Version 0.0.7 2010/06/10 00028 * Misra-C changes done 00029 * -------------------------------------------------------------------- */ 00030 00031 /* ---------------------------------------------------------------------- 00032 * Include standard header files 00033 * -------------------------------------------------------------------- */ 00034 #include<math.h> 00035 00036 /* ---------------------------------------------------------------------- 00037 * Include project header files 00038 * -------------------------------------------------------------------- */ 00039 #include "math_helper.h" 00040 00041 /** 00042 * @brief Caluclation of SNR 00043 * @param float* Pointer to the reference buffer 00044 * @param float* Pointer to the test buffer 00045 * @param uint32_t total number of samples 00046 * @return float SNR 00047 * The function Caluclates signal to noise ratio for the reference output 00048 * and test output 00049 */ 00050 00051 float arm_snr_f32(float *pRef, float *pTest, uint32_t buffSize) 00052 { 00053 float EnergySignal = 0.0, EnergyError = 0.0; 00054 uint32_t i; 00055 float SNR; 00056 00057 for (i = 0; i < buffSize; i++) 00058 { 00059 EnergySignal += pRef[i] * pRef[i]; 00060 EnergyError += (pRef[i] - pTest[i]) * (pRef[i] - pTest[i]); 00061 } 00062 00063 00064 SNR = 10 * log10 (EnergySignal / EnergyError); 00065 00066 return (SNR); 00067 00068 } 00069 00070 00071 /** 00072 * @brief Provide guard bits for Input buffer 00073 * @param q15_t* Pointer to input buffer 00074 * @param uint32_t blockSize 00075 * @param uint32_t guard_bits 00076 * @return none 00077 * The function Provides the guard bits for the buffer 00078 * to avoid overflow 00079 */ 00080 00081 void arm_provide_guard_bits_q15 (q15_t * input_buf, uint32_t blockSize, 00082 uint32_t guard_bits) 00083 { 00084 uint32_t i; 00085 00086 for (i = 0; i < blockSize; i++) 00087 { 00088 input_buf[i] = input_buf[i] >> guard_bits; 00089 } 00090 } 00091 00092 /** 00093 * @brief Converts float to fixed in q12.20 format 00094 * @param uint32_t number of samples in the buffer 00095 * @return none 00096 * The function converts floating point values to fixed point(q12.20) values 00097 */ 00098 00099 void arm_float_to_q12_20(float *pIn, q31_t * pOut, uint32_t numSamples) 00100 { 00101 uint32_t i; 00102 00103 for (i = 0; i < numSamples; i++) 00104 { 00105 /* 1048576.0f corresponds to pow(2, 20) */ 00106 pOut[i] = (q31_t) (pIn[i] * 1048576.0f); 00107 00108 pOut[i] += pIn[i] > 0 ? 0.5 : -0.5; 00109 00110 if (pIn[i] == (float) 1.0) 00111 { 00112 pOut[i] = 0x000FFFFF; 00113 } 00114 } 00115 } 00116 00117 /** 00118 * @brief Compare MATLAB Reference Output and ARM Test output 00119 * @param q15_t* Pointer to Ref buffer 00120 * @param q15_t* Pointer to Test buffer 00121 * @param uint32_t number of samples in the buffer 00122 * @return none 00123 */ 00124 00125 uint32_t arm_compare_fixed_q15(q15_t *pIn, q15_t * pOut, uint32_t numSamples) 00126 { 00127 uint32_t i; 00128 int32_t diff, diffCrnt = 0; 00129 uint32_t maxDiff = 0; 00130 00131 for (i = 0; i < numSamples; i++) 00132 { 00133 diff = pIn[i] - pOut[i]; 00134 diffCrnt = (diff > 0) ? diff : -diff; 00135 00136 if(diffCrnt > maxDiff) 00137 { 00138 maxDiff = diffCrnt; 00139 } 00140 } 00141 00142 return(maxDiff); 00143 } 00144 00145 /** 00146 * @brief Compare MATLAB Reference Output and ARM Test output 00147 * @param q31_t* Pointer to Ref buffer 00148 * @param q31_t* Pointer to Test buffer 00149 * @param uint32_t number of samples in the buffer 00150 * @return none 00151 */ 00152 00153 uint32_t arm_compare_fixed_q31(q31_t *pIn, q31_t * pOut, uint32_t numSamples) 00154 { 00155 uint32_t i; 00156 int32_t diff, diffCrnt = 0; 00157 uint32_t maxDiff = 0; 00158 00159 for (i = 0; i < numSamples; i++) 00160 { 00161 diff = pIn[i] - pOut[i]; 00162 diffCrnt = (diff > 0) ? diff : -diff; 00163 00164 if(diffCrnt > maxDiff) 00165 { 00166 maxDiff = diffCrnt; 00167 } 00168 } 00169 00170 return(maxDiff); 00171 } 00172 00173 /** 00174 * @brief Provide guard bits for Input buffer 00175 * @param q31_t* Pointer to input buffer 00176 * @param uint32_t blockSize 00177 * @param uint32_t guard_bits 00178 * @return none 00179 * The function Provides the guard bits for the buffer 00180 * to avoid overflow 00181 */ 00182 00183 void arm_provide_guard_bits_q31 (q31_t * input_buf, 00184 uint32_t blockSize, 00185 uint32_t guard_bits) 00186 { 00187 uint32_t i; 00188 00189 for (i = 0; i < blockSize; i++) 00190 { 00191 input_buf[i] = input_buf[i] >> guard_bits; 00192 } 00193 } 00194 00195 /** 00196 * @brief Provide guard bits for Input buffer 00197 * @param q31_t* Pointer to input buffer 00198 * @param uint32_t blockSize 00199 * @param uint32_t guard_bits 00200 * @return none 00201 * The function Provides the guard bits for the buffer 00202 * to avoid overflow 00203 */ 00204 00205 void arm_provide_guard_bits_q7 (q7_t * input_buf, 00206 uint32_t blockSize, 00207 uint32_t guard_bits) 00208 { 00209 uint32_t i; 00210 00211 for (i = 0; i < blockSize; i++) 00212 { 00213 input_buf[i] = input_buf[i] >> guard_bits; 00214 } 00215 } 00216 00217 00218 00219 /** 00220 * @brief Caluclates number of guard bits 00221 * @param uint32_t number of additions 00222 * @return none 00223 * The function Caluclates the number of guard bits 00224 * depending on the numtaps 00225 */ 00226 00227 uint32_t arm_calc_guard_bits (uint32_t num_adds) 00228 { 00229 uint32_t i = 1, j = 0; 00230 00231 if (num_adds == 1) 00232 { 00233 return (0); 00234 } 00235 00236 while (i < num_adds) 00237 { 00238 i = i * 2; 00239 j++; 00240 } 00241 00242 return (j); 00243 } 00244 00245 /** 00246 * @brief Converts Q15 to floating-point 00247 * @param uint32_t number of samples in the buffer 00248 * @return none 00249 */ 00250 00251 void arm_apply_guard_bits (float32_t * pIn, 00252 uint32_t numSamples, 00253 uint32_t guard_bits) 00254 { 00255 uint32_t i; 00256 00257 for (i = 0; i < numSamples; i++) 00258 { 00259 pIn[i] = pIn[i] * arm_calc_2pow(guard_bits); 00260 } 00261 } 00262 00263 /** 00264 * @brief Calculates pow(2, numShifts) 00265 * @param uint32_t number of shifts 00266 * @return pow(2, numShifts) 00267 */ 00268 uint32_t arm_calc_2pow(uint32_t numShifts) 00269 { 00270 00271 uint32_t i, val = 1; 00272 00273 for (i = 0; i < numShifts; i++) 00274 { 00275 val = val * 2; 00276 } 00277 00278 return(val); 00279 } 00280 00281 00282 00283 /** 00284 * @brief Converts float to fixed q14 00285 * @param uint32_t number of samples in the buffer 00286 * @return none 00287 * The function converts floating point values to fixed point values 00288 */ 00289 00290 void arm_float_to_q14 (float *pIn, q15_t * pOut, 00291 uint32_t numSamples) 00292 { 00293 uint32_t i; 00294 00295 for (i = 0; i < numSamples; i++) 00296 { 00297 /* 16384.0f corresponds to pow(2, 14) */ 00298 pOut[i] = (q15_t) (pIn[i] * 16384.0f); 00299 00300 pOut[i] += pIn[i] > 0 ? 0.5 : -0.5; 00301 00302 if (pIn[i] == (float) 2.0) 00303 { 00304 pOut[i] = 0x7FFF; 00305 } 00306 00307 } 00308 00309 } 00310 00311 00312 /** 00313 * @brief Converts float to fixed q30 format 00314 * @param uint32_t number of samples in the buffer 00315 * @return none 00316 * The function converts floating point values to fixed point values 00317 */ 00318 00319 void arm_float_to_q30 (float *pIn, q31_t * pOut, 00320 uint32_t numSamples) 00321 { 00322 uint32_t i; 00323 00324 for (i = 0; i < numSamples; i++) 00325 { 00326 /* 1073741824.0f corresponds to pow(2, 30) */ 00327 pOut[i] = (q31_t) (pIn[i] * 1073741824.0f); 00328 00329 pOut[i] += pIn[i] > 0 ? 0.5 : -0.5; 00330 00331 if (pIn[i] == (float) 2.0) 00332 { 00333 pOut[i] = 0x7FFFFFFF; 00334 } 00335 } 00336 } 00337 00338 /** 00339 * @brief Converts float to fixed q30 format 00340 * @param uint32_t number of samples in the buffer 00341 * @return none 00342 * The function converts floating point values to fixed point values 00343 */ 00344 00345 void arm_float_to_q29 (float *pIn, q31_t * pOut, 00346 uint32_t numSamples) 00347 { 00348 uint32_t i; 00349 00350 for (i = 0; i < numSamples; i++) 00351 { 00352 /* 1073741824.0f corresponds to pow(2, 30) */ 00353 pOut[i] = (q31_t) (pIn[i] * 536870912.0f); 00354 00355 pOut[i] += pIn[i] > 0 ? 0.5 : -0.5; 00356 00357 if (pIn[i] == (float) 4.0) 00358 { 00359 pOut[i] = 0x7FFFFFFF; 00360 } 00361 } 00362 } 00363 00364 00365 /** 00366 * @brief Converts float to fixed q28 format 00367 * @param uint32_t number of samples in the buffer 00368 * @return none 00369 * The function converts floating point values to fixed point values 00370 */ 00371 00372 void arm_float_to_q28 (float *pIn, q31_t * pOut, 00373 uint32_t numSamples) 00374 { 00375 uint32_t i; 00376 00377 for (i = 0; i < numSamples; i++) 00378 { 00379 /* 268435456.0f corresponds to pow(2, 28) */ 00380 pOut[i] = (q31_t) (pIn[i] * 268435456.0f); 00381 00382 pOut[i] += pIn[i] > 0 ? 0.5 : -0.5; 00383 00384 if (pIn[i] == (float) 8.0) 00385 { 00386 pOut[i] = 0x7FFFFFFF; 00387 } 00388 } 00389 } 00390 00391 /** 00392 * @brief Clip the float values to +/- 1 00393 * @param pIn input buffer 00394 * @param numSamples number of samples in the buffer 00395 * @return none 00396 * The function converts floating point values to fixed point values 00397 */ 00398 00399 void arm_clip_f32 (float *pIn, uint32_t numSamples) 00400 { 00401 uint32_t i; 00402 00403 for (i = 0; i < numSamples; i++) 00404 { 00405 if(pIn[i] > 1.0f) 00406 { 00407 pIn[i] = 1.0; 00408 } 00409 else if( pIn[i] < -1.0f) 00410 { 00411 pIn[i] = -1.0; 00412 } 00413 00414 } 00415 } 00416 00417 00418 00419
Generated on Tue Jul 12 2022 14:13:55 by
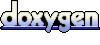