CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_var_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_var_q31.c 00009 * 00010 * Description: Variance of an array of Q31 type. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 /** 00034 * @addtogroup variance 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Variance of the elements of a Q31 vector. 00040 * @param[in] *pSrc points to the input vector 00041 * @param[in] blockSize length of the input vector 00042 * @param[out] *pResult variance value returned here 00043 * @return none. 00044 * 00045 * @details 00046 * <b>Scaling and Overflow Behavior:</b> 00047 * 00048 *\par 00049 * The function is implemented using an internal 64-bit accumulator. 00050 * The input is represented in 1.31 format, and intermediate multiplication 00051 * yields a 2.62 format. 00052 * The accumulator maintains full precision of the intermediate multiplication results, 00053 * but provides only a single guard bit. 00054 * There is no saturation on intermediate additions. 00055 * If the accumulator overflows it wraps around and distorts the result. 00056 * In order to avoid overflows completely the input signal must be scaled down by 00057 * log2(blockSize) bits, as a total of blockSize additions are performed internally. 00058 * Finally, the 2.62 accumulator is right shifted by 31 bits to yield a 1.31 format value. 00059 * 00060 */ 00061 00062 00063 void arm_var_q31( 00064 q31_t * pSrc, 00065 uint32_t blockSize, 00066 q63_t * pResult) 00067 { 00068 q63_t sum = 0; /* Accumulator */ 00069 q31_t meanOfSquares, squareOfMean; /* Mean of square and square of mean */ 00070 q31_t mean; /* Mean */ 00071 q31_t in; /* Input variable */ 00072 q31_t t; /* Temporary variable */ 00073 uint32_t blkCnt; /* loop counter */ 00074 q31_t *pIn; /* Temporary pointer */ 00075 00076 pIn = pSrc; 00077 00078 /*loop Unrolling */ 00079 blkCnt = blockSize >> 2u; 00080 00081 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00082 ** a second loop below computes the remaining 1 to 3 samples. */ 00083 while(blkCnt > 0u) 00084 { 00085 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00086 /* Compute Sum of squares of the input samples 00087 * and then store the result in a temporary variable, sum. */ 00088 in = *pSrc++; 00089 sum += ((q63_t) (in) * (in)); 00090 in = *pSrc++; 00091 sum += ((q63_t) (in) * (in)); 00092 in = *pSrc++; 00093 sum += ((q63_t) (in) * (in)); 00094 in = *pSrc++; 00095 sum += ((q63_t) (in) * (in)); 00096 00097 /* Decrement the loop counter */ 00098 blkCnt--; 00099 } 00100 00101 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00102 ** No loop unrolling is used. */ 00103 blkCnt = blockSize % 0x4u; 00104 00105 while(blkCnt > 0u) 00106 { 00107 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00108 /* Compute Sum of squares of the input samples 00109 * and then store the result in a temporary variable, sum. */ 00110 in = *pSrc++; 00111 sum += ((q63_t) (in) * (in)); 00112 00113 /* Decrement the loop counter */ 00114 blkCnt--; 00115 } 00116 00117 /* Compute Mean of squares of the input samples 00118 * and then store the result in a temporary variable, meanOfSquares. */ 00119 t = (q31_t) ((1.0 / (blockSize - 1)) * 1073741824LL); 00120 sum = (sum >> 31); 00121 meanOfSquares = (q31_t) ((sum * t) >> 30); 00122 00123 /* Reset the accumulator */ 00124 sum = 0; 00125 00126 /*loop Unrolling */ 00127 blkCnt = blockSize >> 2u; 00128 00129 /* Reset the input working pointer */ 00130 pSrc = pIn; 00131 00132 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00133 ** a second loop below computes the remaining 1 to 3 samples. */ 00134 while(blkCnt > 0u) 00135 { 00136 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00137 /* Compute sum of all input values and then store the result in a temporary variable, sum. */ 00138 sum += *pSrc++; 00139 sum += *pSrc++; 00140 sum += *pSrc++; 00141 sum += *pSrc++; 00142 00143 /* Decrement the loop counter */ 00144 blkCnt--; 00145 } 00146 00147 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00148 ** No loop unrolling is used. */ 00149 blkCnt = blockSize % 0x4u; 00150 00151 while(blkCnt > 0u) 00152 { 00153 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00154 /* Compute sum of all input values and then store the result in a temporary variable, sum. */ 00155 sum += *pSrc++; 00156 00157 /* Decrement the loop counter */ 00158 blkCnt--; 00159 } 00160 00161 /* Compute mean of all input values */ 00162 t = (q31_t) ((1.0 / (blockSize * (blockSize - 1u))) * 2147483648LL); 00163 mean = (q31_t) (sum); 00164 00165 /* Compute square of mean */ 00166 squareOfMean = (q31_t) (((q63_t) mean * mean) >> 31); 00167 squareOfMean = (q31_t) (((q63_t) squareOfMean * t) >> 31); 00168 00169 /* Compute variance and then store the result to the destination */ 00170 *pResult = (q63_t) meanOfSquares - squareOfMean; 00171 00172 } 00173 00174 /** 00175 * @} end of variance group 00176 */
Generated on Tue Jul 12 2022 14:13:55 by
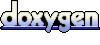