CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_shift_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_shift_q31.c 00009 * 00010 * Description: Shifts the elements of a Q31 vector 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.7 2010/06/10 00027 * Misra-C changes done 00028 * -------------------------------------------------------------------- */ 00029 00030 #include "arm_math.h" 00031 00032 /** 00033 * @ingroup groupMath 00034 */ 00035 /** 00036 * @defgroup shift Vector Shift 00037 * 00038 * Shifts the elements of a fixed-point vector by a specified number of bits. 00039 * There are separate functions for Q7, Q15, and Q31 data types. 00040 * The underlying algorithm used is: 00041 * 00042 * <pre> 00043 * pDst[n] = pSrc[n] << shift, 0 <= n < blockSize. 00044 * </pre> 00045 * 00046 * If <code>shift</code> is positive then the elements of the vector are shifted to the left. 00047 * If <code>shift</code> is negative then the elements of the vector are shifted to the right. 00048 */ 00049 00050 /** 00051 * @addtogroup shift 00052 * @{ 00053 */ 00054 00055 /** 00056 * @brief Shifts the elements of a Q31 vector a specified number of bits. 00057 * @param *pSrc points to the input vector 00058 * @param shiftBits number of bits to shift. A positive value shifts left; a negative value shifts right. 00059 * @param *pDst points to the output vector 00060 * @param blockSize number of samples in the vector 00061 * @return none. 00062 * 00063 * 00064 * <b>Scaling and Overflow Behavior:</b> 00065 * \par 00066 * The function uses saturating arithmetic. 00067 * Results outside of the allowable Q31 range [0x80000000 0x7FFFFFFF] will be saturated. 00068 */ 00069 00070 void arm_shift_q31( 00071 q31_t * pSrc, 00072 int8_t shiftBits, 00073 q31_t * pDst, 00074 uint32_t blockSize) 00075 { 00076 uint32_t blkCnt; /* loop counter */ 00077 uint32_t sign; /* Sign of shiftBits */ 00078 00079 00080 /*loop Unrolling */ 00081 blkCnt = blockSize >> 2u; 00082 00083 /* Getting the sign of shiftBits */ 00084 sign = (shiftBits & 0x80000000); 00085 00086 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00087 ** a second loop below computes the remaining 1 to 3 samples. */ 00088 while(blkCnt > 0u) 00089 { 00090 /* C = A (>> or <<) shiftBits */ 00091 /* Shift the input and then store the results in the destination buffer. */ 00092 *pDst++ = (sign == 0u) ? clip_q63_to_q31((q63_t) * pSrc++ << shiftBits) : 00093 (*pSrc++ >> -shiftBits); 00094 *pDst++ = (sign == 0u) ? clip_q63_to_q31((q63_t) * pSrc++ << shiftBits) : 00095 (*pSrc++ >> -shiftBits); 00096 *pDst++ = (sign == 0u) ? clip_q63_to_q31((q63_t) * pSrc++ << shiftBits) : 00097 (*pSrc++ >> -shiftBits); 00098 *pDst++ = (sign == 0u) ? clip_q63_to_q31((q63_t) * pSrc++ << shiftBits) : 00099 (*pSrc++ >> -shiftBits); 00100 00101 /* Decrement the loop counter */ 00102 blkCnt--; 00103 } 00104 00105 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00106 ** No loop unrolling is used. */ 00107 blkCnt = blockSize % 0x4u; 00108 00109 while(blkCnt > 0u) 00110 { 00111 /* C = A (>> or <<) shiftBits */ 00112 /* Shift the input and then store the result in the destination buffer. */ 00113 *pDst++ = (sign == 0u) ? clip_q63_to_q31((q63_t) * pSrc++ << shiftBits) : 00114 (*pSrc++ >> -shiftBits); 00115 00116 /* Decrement the loop counter */ 00117 blkCnt--; 00118 } 00119 00120 } 00121 00122 /** 00123 * @} end of shift group 00124 */
Generated on Tue Jul 12 2022 14:13:54 by
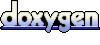